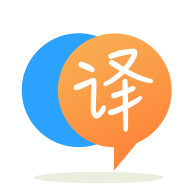
[英]BitmapFactory.decodeStream(inputStream) always return null when some bytes are wrong
[英]Android BinaryFactory.decodeStream always return null
我正在嘗試下載圖像並使用BitmapFactory將其解碼為位圖,但encodeStream始終返回null。 我在Google上搜索了許多類似的問題,嘗試了許多示例,但沒有找到解決方案。
這是我的代碼:
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
public void downloadButton(View v)
{
String imageUrl = "http://www.picgifs.com/bird-graphics/bird-graphics/elf-owl/bird-graphics-elf-owl-527150.bmp";
new Thread(new ImageDownloader(imageUrl)).start();
}
public void showImageButton(View v)
{
Bitmap image = ImageHandler.getImage();
if (image == null)
Log.e("Error", "No image available");
else {
ImageView imageView = (ImageView)findViewById(R.id.ImageView);
imageView.setImageBitmap(image);
}
}
}
class ImageHandler
{
static protected List<Bitmap> imagesList;
static public void addImage(Bitmap image)
{
imagesList.add(image);
}
static public Bitmap getImage()
{
if (!imagesList.isEmpty())
return imagesList.get(0);
else
return null;
}
}
class ImageDownloader implements Runnable
{
public Bitmap bmpImage;
private String urlString;
public ImageDownloader(String urlString)
{
this.urlString = urlString;
}
public void run()
{
try
{
AndroidHttpClient client = AndroidHttpClient.newInstance("Android");
HttpGet getRequest = new HttpGet(urlString);
HttpResponse response = client.execute(getRequest);
HttpEntity entity = response.getEntity();
InputStream inputStream = null;
inputStream = (new BufferedHttpEntity(entity)).getContent();
bmpImage = BitmapFactory.decodeStream(inputStream);
//bmpImage = BitmapFactory.decodeStream(new URL(urlString).openConnection().getInputStream());
}
catch (Exception e)
{
Log.e("ImageDownloadError", e.toString());
return;
}
if (bmpImage != null)
{
ImageHandler.addImage(bmpImage);
Log.i("Info", "Image download successfully");
}
else
Log.e("Error", "Bitmap is null");
}
}
ps showImageButton
拋出IllegalStateException
,但是我已經厭倦了它。
我認為問題在於,一旦您從HttpUrlConnection使用了InputStream,就無法后退並再次使用相同的InputStream。 因此,您必須為圖像的實際采樣創建一個新的InputStream。 否則,我們必須中止http請求。
只有當我們可以將inputstream用於httprequest時,如果您嘗試下載其他圖像,則它將像“已創建InputStream”那樣拋出錯誤。 因此,一旦使用httpRequest.abort()下載后,我們需要中止httprequest。
用這個:
HttpGet httpRequest = new HttpGet(URI.create(path) );
HttpClient httpclient = new DefaultHttpClient();
HttpResponse response = (HttpResponse) httpclient.execute(httpRequest);
HttpEntity entity = response.getEntity();
BufferedHttpEntity bufHttpEntity = new BufferedHttpEntity(entity);
bmp = BitmapFactory.decodeStream(bufHttpEntity.getContent());
httpRequest.abort();
根據我的觀點,有兩個原因
我正在上傳一個有效的代碼,希望這可以解決您的問題。
主要活動
public class MainActivity extends Activity {
private ProgressDialog progressDialog;
private ImageView imageView;
private String url = "http://www.9ori.com/store/media/images/8ab579a656.jpg";
private Bitmap bitmap = null;
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
imageView = (ImageView) findViewById(R.id.imageView);
Button start = (Button) findViewById(R.id.button1);
start.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
progressDialog = ProgressDialog.show(MainActivity.this,
"", "Loading..");
new Thread() {
public void run() {
bitmap = downloadBitmap(url);
messageHandler.sendEmptyMessage(0);
}
}.start();
}
});
}
private Handler messageHandler = new Handler() {
public void handleMessage(Message msg) {
super.handleMessage(msg);
imageView.setImageBitmap(bitmap);
progressDialog.dismiss();
}
};
private Bitmap downloadBitmap(String url) {
// Initialize the default HTTP client object
final DefaultHttpClient client = new DefaultHttpClient();
//forming a HttoGet request
final HttpGet getRequest = new HttpGet(url);
try {
HttpResponse response = client.execute(getRequest);
//check 200 OK for success
final int statusCode = response.getStatusLine().getStatusCode();
if (statusCode != HttpStatus.SC_OK) {
Log.w("ImageDownloader", "Error " + statusCode +
" while retrieving bitmap from " + url);
return null;
}
final HttpEntity entity = response.getEntity();
if (entity != null) {
InputStream is = null;
try {
// getting contents from the stream
is = entity.getContent();
// decoding stream data back into image Bitmap
final Bitmap bitmap = BitmapFactory.decodeStream(is);
return bitmap;
} finally {
if (is != null) {
is.close();
}
entity.consumeContent();
}
}
} catch (Exception e) {
getRequest.abort();
Log.e(getString(R.string.app_name), "Error "+ e.toString());
}
return null;
}
}
activity_main.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity" >
<Button
android:id="@+id/button1"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_margin="15dp"
android:text="Download Image" />
<ImageView
android:id="@+id/imageView"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:scaleType="centerInside"
android:src="@drawable/ic_launcher" />
</LinearLayout>
並且不要忘記在Manifest.xml文件中授予INTERNET權限
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.