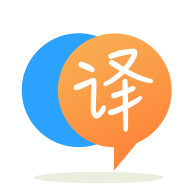
[英]With OpenGL ES 2.0 on Android, is there a way preserve multisampling when rendering on a framebuffer?
[英]Android Opengl ES 2.0 FrameBuffer not working
我正在研究2D Android項目,我希望使用FBO將內容繪制到空紋理,然后在屏幕上繪制該紋理。 出於調試目的,FBO附加紋理應立即變為藍色,以便我可以告訴我能夠編輯紋理,因為它目前甚至不會影響紋理。
幀緩沖區:
public static class FrameBuffer{
private final FaustEngine engine;
private final int width;
private final int height;
private final int[] fboId = new int[1];
private final int[] renId = new int[1];
public FrameBuffer( FaustEngine Engine, int Width, int Height ){
engine = Engine;
width = Width;
height = Height;
GLES20.glGenRenderbuffers( 1, renId, 0 );
GLES20.glBindRenderbuffer( GLES20.GL_RENDERBUFFER, renId[0] );
GLES20.glRenderbufferStorage( GLES20.GL_RENDERBUFFER, GLES20.GL_DEPTH_COMPONENT, width, height );
GLES20.glGenFramebuffers( 1, fboId, 0 );
GLES20.glBindFramebuffer( GLES20.GL_FRAMEBUFFER, fboId[0] );
GLES20.glFramebufferRenderbuffer( GLES20.GL_FRAMEBUFFER, GLES20.GL_DEPTH_ATTACHMENT, GLES20.GL_RENDERBUFFER, renId[0] );
GLES20.glBindRenderbuffer( GLES20.GL_RENDERBUFFER, 0);
GLES20.glBindFramebuffer( GLES20.GL_FRAMEBUFFER, 0);
}
public void bind( int texId, int texWidth, int texHeight ){
GLES20.glBindFramebuffer( GLES20.GL_FRAMEBUFFER, fboId[0] );
GLES20.glFramebufferTexture2D( GLES20.GL_FRAMEBUFFER, GLES20.GL_COLOR_ATTACHMENT0, GLES20.GL_TEXTURE_2D, texId, 0 );
GLES20.glViewport( 0, 0, texWidth, texHeight );
GLES20.glClearColor(0f, 0f, 1f, 1f );
GLES20.glClear( GLES20.GL_COLOR_BUFFER_BIT ); //Turn blue
//Fix projection matrix
Matrix.orthoM( engine.getOrthoMatrix() , 0, 0, texWidth, texHeight, 0, -1, 1 );
}
public void unbind(){
GLES20.glBindFramebuffer( GLES20.GL_FRAMEBUFFER, 0 );
GLES20.glViewport( 0, 0, engine.getScreenWidth(), engine.getScreenHeight() );
//Reset projection matrix
Matrix.orthoM( engine.getOrthoMatrix() , 0, 0, engine.getScreenWidth(), engine.getScreenHeight(), 0, -1, 1 );
}
}
渲染代碼:
FrameBuffer fbo = new FrameBuffer( game.getEngine(), 1024, 1024 );
final int[] texIds = new int[1];
game.getEngine().createBlankTextures( texIds, 1024, 1024 );
fbo.bind( texIds[0], 1024, 1024 );
//texture should turn blue here since in the bind() function I placed a glClear with blue
fbo.unbind();
紋理創建:
public void createBlankTextures( int[] texIds, int width, int height ){
GLES20.glGenTextures( texIds.length, texIds, 0 );
for( int i=0; i<texIds.length; i++ ){
GLES20.glBindTexture( GLES20.GL_TEXTURE_2D, texIds[i] );
GLES20.glTexParameteri( GLES20.GL_TEXTURE_2D, GLES20.GL_TEXTURE_MIN_FILTER, GLES20.GL_NEAREST );
GLES20.glTexParameteri( GLES20.GL_TEXTURE_2D, GLES20.GL_TEXTURE_MAG_FILTER, GLES20.GL_NEAREST );
GLES20.glTexImage2D( GLES20.GL_TEXTURE_2D, 0, GLES20.GL_RGBA, width, height, 0, GLES20.GL_RGBA, GLES20.GL_UNSIGNED_BYTE, null );
GLES20.glGenerateMipmap( GLES20.GL_TEXTURE_2D );
}
}
所以我有2個問題。
1.)我是否需要使用Framebuffer的Renderbuffer? 我不想跟蹤深度。 我只想編輯現有的純2D紋理。
2.)為什么這不起作用?
如果您不需要FBO渲染的深度緩沖區,則無需創建渲染緩沖區。
GL_DEPTH_COMPONENT
不是ES 2.0中glRenderbufferStorage()
的internalformat
參數的glRenderbufferStorage()
。 如果查看支持的值( http://www.khronos.org/opengles/sdk/docs/man/xhtml/glRenderbufferStorage.xml ),唯一的深度格式是GL_DEPTH_COMPONENT16
。
和往常一樣, glGetError()
是你的朋友。 它應該指示glRenderbufferStorage()
調用中的GL_INVALID_ENUM
錯誤。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.