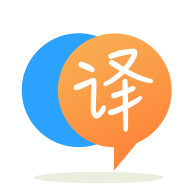
[英]how do i pass program arguments in java for my Fitnesse Fixture?
[英]How can I pass arguments like these for example: runType=EOD threadCnt=10 cleanLogs=true in my commandline java program?
將這些參數傳遞給Java命令行程序的最佳方法是什么。 當前,我有用戶必須記住的依賴於順序的參數。 我的程序如下所示:
CommandLineRunner EOD 10 true
我希望用戶能夠執行此操作:
CommandLineRunner runType=EOD threadCnt=10 cleanLogs=true
或更改參數的順序。
CommandLineRunner threadCnt=10 runType=EOD cleanLogs=true
我不希望為此使用外部庫,但是如果java中有一個好的庫,請提供一個示例,說明如何使用它來構建我的示例。 =
符號必須存在,因為我需要使該程序遵循我們組織中其他基於python / ruby的cli應用程序的標准。
先感謝您。
您可以在主類之后在命令行中傳遞參數:
java CommandLineRunner "runType=EOD" "threadCnt=10" "cleanLogs=true"
然后在程序中:
import java.util.*;
public class CommandLineRunner {
public static void main(String args[]){
//Collect the input parameters...
HashMap<String, String> argsMap = new HashMap<String, String>();
for (int i = 0; i < args.length; i++){
StringTokenizer st = new StringTokenizer(args[i], "=");
while(st.hasMoreTokens()) {
String key = st.nextToken();
//Handle the case you don't define a key/value with '='
try{
String val = st.nextToken();
argsMap.put(key, val);
}catch(NoSuchElementException e){
argsMap.put(key, "");
}
}
}
//Then use them when needed...
for (Map.Entry<String,String> entry : argsMap.entrySet()) {
String key = entry.getKey();
String value = entry.getValue();
System.out.println("arg: "+ key + "\tval:" + value);
if(key.equals("runType")){
//Add your business logic here
}
else if (key.equals("threadCnt")){
//Add your business logic here
}
else if (key.equals("cleanLogs")){
//Add your business logic here
}else
//Unknown argument
throw new RuntimeException("Invalid argument!");
}
}
}
因此,無論輸入順序如何,您都將在地圖中包含參數。
在Java7
您也可以使用更優雅的switch
構造來處理String
(因此可以使用您的參數):
//Then use them when needed...
for (Map.Entry<String,String> entry : argsMap.entrySet()) {
String key = entry.getKey();
String value = entry.getValue();
System.out.println("arg: "+ key + "\tval:" + value);
switch (key) {
case "runType":
//Add your business logic here
break;
case "threadCnt":
//Add your business logic here
break;
case "cleanLogs":
//Add your business logic here
break;
default:
//Unknown argument
throw new RuntimeException("Invalid argument!");
}
}
只要您在值中不使用空格,這將起作用:
java CommandLineRunner threadCnt=10 runType=EOD cleanLogs=true
然后,您必須解析這三個參數中的每一個。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.