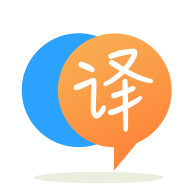
[英]error: argument of type ‘void* (Thread::)(void*)’ does not match ‘void* (*)(void*)’
[英]argument of type 'void* (…)(void*)' does not match 'void* (*)(void*)
我目前正在GNU Radio上開發一個bloc,我想使用一個線程。 該線程在那里可以從UDP套接字獲取數據,因此我可以在GNU Radio bloc中使用它。 “一般工作”功能是完成所有信號和數據處理的功能。
主源文件的組織方式如下:
namespace gr {
namespace adsb {
out::sptr
out::make()
{
return gnuradio::get_initial_sptr
(new out_impl());
}
/*
* UDP thread
*/
void *task_UdpRx (void *arg)
{
while(true)
{
printf("Task UdpRx\n\r");
usleep(500*1000);
}
pthread_exit(NULL);
}
/*
* The private constructor
*/
out_impl::out_impl()
: gr::block("out",
gr::io_signature::make(1, 1, sizeof(int)),
gr::io_signature::make(1, 1, sizeof(char)))
{
pthread_t Thread_UdpRx;
//Thread init
if(pthread_create(&Thread_UdpRx, NULL, task_UdpRx, NULL))
{
err("Pthread error");
}
else
{
printf("UDP thread initialization completed\n\r");
}
}
/*
* Our virtual destructor.
*/
out_impl::~out_impl()
{
}
void out_impl::forecast (int noutput_items, gr_vector_int &ninput_items_required)
{
ninput_items_required[0] = noutput_items;
}
int out_impl::general_work (int noutput_items, gr_vector_int &ninput_items, gr_vector_const_void_star &input_items, gr_vector_void_star &output_items)
{
const int *in = (const int *) input_items[0];
char *out = (char *) output_items[0];
// Do <+signal processing+>
for(int i = 0; i < noutput_items; i++)
{
printf("General work\n\r");
}/* for < noutput_items */
// Tell runtime system how many input items we consumed on
// each input stream.
consume_each (noutput_items);
// Tell runtime system how many output items we produced.
return noutput_items;
} /* general work */
} /* namespace adsb */
} /* namespace gr */`
我得到的問題是,當我嘗試編譯時,出現此錯誤:
In constructor ‘gr::adsb::out_impl::out_impl()’:
error: argument of type ‘void* (gr::adsb::out_impl::)(void*)’ does not match ‘void* (*)(void*)’
此錯誤涉及該行,並且涉及task_UdpRx:
if(pthread_create(&Thread_UdpRx, NULL, task_UdpRx, NULL))
有人知道嗎?
如果需要,請隨時詢問更多詳細信息。 我所顯示的代碼是我能做的最短的代碼,以使您盡可能獲得最佳理解。
謝謝 !
您不能將指向成員函數的指針傳遞給pthread_create()
。
您需要一個免費功能:
void *thread_start(void *object)
{
gr::adsb::out_impl *object = reinterpret_cast<gr::adsb::out_impl *>(object);
object.task_UpdRx(0);
return 0;
}
或其附近,則需要將此函數傳遞給pthread_create()
並將this
指針作為數據參數傳遞:
if (pthread_create(&Thread_UdpRx, NULL, thread_start, this))
我保留濫用reinterpret_cast<>()
-替換正確的強制轉換符號。 我對在構造函數中啟動線程有所保留,但這是應該起作用的方案的概述。
該代碼仍然不是MCVE。 我無法復制並編譯它。 缺少許多標題。 未定義/聲明out
和out_impl
類型。 一些GNU Radio繼承的東西可能會被忽略掉。
這是我的MCVE版本。 它不是完美的,因為它不會重現您的問題。 它可以在帶有GCC 4.9.0的Ubuntu 12.04 LTS衍生版本上干凈地編譯。 假定您具有定義函數err()
的頭文件<err.h>
。 我不確定MCVE是否需要析構函數(刪除析構函數將消除另外5行左右)。
#include <cstdio>
#include <pthread.h>
#include <unistd.h>
#include <err.h>
namespace gr
{
namespace adsb
{
class out_impl
{
public:
out_impl();
virtual ~out_impl();
};
void *task_UdpRx(void *arg)
{
while (true)
{
printf("Task UdpRx %p\n\r", arg);
usleep(500*1000);
}
pthread_exit(NULL);
}
out_impl::out_impl()
{
pthread_t Thread_UdpRx;
if (pthread_create(&Thread_UdpRx, NULL, task_UdpRx, NULL))
err(1, "Pthread error");
else
printf("UDP thread initialization completed\n\r");
}
out_impl::~out_impl()
{
}
}
}
$ g++ --version
g++ (GCC) 4.9.0
Copyright (C) 2014 Free Software Foundation, Inc.
This is free software; see the source for copying conditions. There is NO
warranty; not even for MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
$ make gr.o
g++ -g -O3 -std=c++11 -Wall -Wextra -Werror -c gr.cpp
$ nm -C -g gr.o
0000000000000010 T gr::adsb::task_UdpRx(void*)
0000000000000050 T gr::adsb::out_impl::out_impl()
0000000000000050 T gr::adsb::out_impl::out_impl()
0000000000000040 T gr::adsb::out_impl::~out_impl()
0000000000000000 T gr::adsb::out_impl::~out_impl()
0000000000000000 T gr::adsb::out_impl::~out_impl()
0000000000000000 V typeinfo for gr::adsb::out_impl
0000000000000000 V typeinfo name for gr::adsb::out_impl
U vtable for __cxxabiv1::__class_type_info
0000000000000000 V vtable for gr::adsb::out_impl
U operator delete(void*)
U err
U printf
U pthread_create
U usleep
$
從這里可以通過以下幾種方式進行問答:
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.