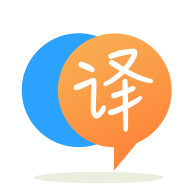
[英]ngrx how to find object by string id and replace in array of objects
[英]How to find object by id in array of objects?
我有這個json文件:
var data = [{
"id": 0,
"parentId": null,
"name": "Comapny",
"children": [
{
"id": 1235,
"parentId": 0,
"name": "Experiences",
"children": [
{
"id": 3333,
"parentId": 154,
"name": "Lifestyle",
"children": []
},
{
"id": 319291392,
"parentId": 318767104,
"name": "Other Experiences",
"children": []
}
]
}
]
}];
我需要按ID查找對象。 例如,如果需要查找ID為319291392的對象,則必須獲取:
{"id": 319291392,"parentId": 318767104,"name": "Other Experiences","children": []}
我怎樣才能做到這一點?
我嘗試使用此功能:
function findId(obj, id) {
if (obj.id == id) {
return obj;
}
if (obj.children) {
for (var i = 0; i < obj.children.length; i++) {
var found = findId(obj.children[i], id);
if (found) {
return found;
}
}
}
return false;
}
但這不起作用,因為它是一個對象數組。
如果您的起點是一個數組,那么您要稍微反轉一下邏輯,從數組而不是對象開始:
function findId(array, id) {
var i, found, obj;
for (i = 0; i < array.length; ++i) {
obj = array[i];
if (obj.id == id) {
return obj;
}
if (obj.children) {
found = findId(obj.children, id);
if (found) {
return found;
}
}
}
return false; // <= You might consider null or undefined here
}
然后
var result = findId(data, 319291392);
...找到id
319291392的對象。
這應該為您工作:
var serachById = function (id,data) {
for (var i = 0; i < data.length; i++) {
if(id==data[i].id)
return data[i];
if(data[i].children.length>0)
return serachById(id,data[i].children);
};
return null;
}
console.log(serachById(0,data));
這是使用對象表示法的另一種簡單解決方案。 即使您決定放棄數組並稍后使用對象符號,此解決方案也將起作用。 因此代碼將保持不變。
當您有沒有子元素的元素時,它也會支持這種情況。
function findId(obj, id) {
var current, index, reply;
// Use the object notation instead of index.
for (index in obj) {
current = obj[index];
if (current.id === id) {
return current;
}
reply = findId(current.children, id);
if (reply) {
return reply;
}
// If you reached this point nothing was found.
console.log('No match found');
}
}
console.log(findId(data, 319291392));
這樣做:
for (var obj in arr) {
if(arr[obj].id== id) {
console.log(arr[obj]);
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.