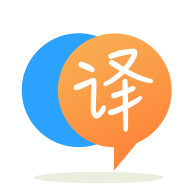
[英]Deserialise Dynamic JSON string with Hierarchical data using JSON.NET
[英]JSON Web Request string to parsed data with JSON.NET
我正在使用.net調用Web服務,然后將其解析為可用數據。
現在,我正在嘗試使用此調用: http : //www.reddit.com/r/all.json ,該調用返回: http ://pastebin.com/AbV4yVuC。這被放入一個字符串中,我稱為jsontxt。
我正在使用JSON.NET解析信息,但它似乎無法正常工作。 我最初嘗試將其反序列化為一個對象,但由於變量中未放入任何內容而無法正常工作
然后我嘗試將其反序列化為數據集,但我再也沒有運氣了。 我的錯誤是
Newtonsoft.Json.dll中發生了類型為'Newtonsoft.Json.JsonException'的未處理異常
我的密碼:
using Newtonsoft.Json;
using Newtonsoft.Json.Linq;
using System;
using System.Collections.Generic;
using System.Data;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace tutorialresult1
{
class Program
{
static void Main(string[] args)
{
using (var webClient = new System.Net.WebClient())
{
var jsontxt = webClient.DownloadString("http://www.reddit.com/r/all.json");
// Console.Write(json);
// -----Deserializing by Object--------------
//MediaEmbed account = JsonConvert.DeserializeObject<MediaEmbed>(jsontxt);
//Console.WriteLine(account.width); //COMES OUT TO NULL
// -----Deserializing by DataSet--------------
DataSet dataSet = JsonConvert.DeserializeObject<DataSet>(jsontxt);
DataTable dataTable = dataSet.Tables["Children"];
Console.WriteLine(dataTable.Rows.Count);
}
}
public class MediaEmbed
{
public string content { get; set; }
public int width { get; set; }
public bool scrolling { get; set; }
public int height { get; set; }
}
.... //rest of classes here for each json which were generated with http://json2csharp.com/
}
}
我只是想通過解析使JSON易於訪問。
您正在嘗試將json字符串反序列化為數據集對象。 但是json-string沒有數據集的格式。 因此,您需要創建一個與json匹配的類,或將其反序列化為字典或sth。 像這樣。
使用json2charp,我生成了以下類集。 使用這些,您應該能夠使用JSON.NET將JSON反序列化為RootObject。
var account = JsonConvert.DeserializeObject<RootObject>(jsontxt);
。
public class MediaEmbed
{
public string content { get; set; }
public int? width { get; set; }
public bool? scrolling { get; set; }
public int? height { get; set; }
}
public class Oembed
{
public string provider_url { get; set; }
public string description { get; set; }
public string title { get; set; }
public string url { get; set; }
public string type { get; set; }
public string author_name { get; set; }
public int height { get; set; }
public int width { get; set; }
public string html { get; set; }
public int thumbnail_width { get; set; }
public string version { get; set; }
public string provider_name { get; set; }
public string thumbnail_url { get; set; }
public int thumbnail_height { get; set; }
public string author_url { get; set; }
}
public class SecureMedia
{
public Oembed oembed { get; set; }
public string type { get; set; }
}
public class SecureMediaEmbed
{
public string content { get; set; }
public int? width { get; set; }
public bool? scrolling { get; set; }
public int? height { get; set; }
}
public class Oembed2
{
public string provider_url { get; set; }
public string description { get; set; }
public string title { get; set; }
public int thumbnail_width { get; set; }
public int height { get; set; }
public int width { get; set; }
public string html { get; set; }
public string version { get; set; }
public string provider_name { get; set; }
public string thumbnail_url { get; set; }
public string type { get; set; }
public int thumbnail_height { get; set; }
public string url { get; set; }
public string author_name { get; set; }
public string author_url { get; set; }
}
public class Media
{
public string type { get; set; }
public Oembed2 oembed { get; set; }
}
public class Data2
{
public string domain { get; set; }
public object banned_by { get; set; }
public MediaEmbed media_embed { get; set; }
public string subreddit { get; set; }
public string selftext_html { get; set; }
public string selftext { get; set; }
public object likes { get; set; }
public SecureMedia secure_media { get; set; }
public string link_flair_text { get; set; }
public string id { get; set; }
public int gilded { get; set; }
public SecureMediaEmbed secure_media_embed { get; set; }
public bool clicked { get; set; }
public bool stickied { get; set; }
public string author { get; set; }
public Media media { get; set; }
public int score { get; set; }
public object approved_by { get; set; }
public bool over_18 { get; set; }
public bool hidden { get; set; }
public string thumbnail { get; set; }
public string subreddit_id { get; set; }
public object edited { get; set; }
public string link_flair_css_class { get; set; }
public object author_flair_css_class { get; set; }
public int downs { get; set; }
public bool saved { get; set; }
public bool is_self { get; set; }
public string permalink { get; set; }
public string name { get; set; }
public double created { get; set; }
public string url { get; set; }
public object author_flair_text { get; set; }
public string title { get; set; }
public double created_utc { get; set; }
public int ups { get; set; }
public int num_comments { get; set; }
public bool visited { get; set; }
public object num_reports { get; set; }
public object distinguished { get; set; }
}
public class Child
{
public string kind { get; set; }
public Data2 data { get; set; }
}
public class Data
{
public string modhash { get; set; }
public List<Child> children { get; set; }
public string after { get; set; }
public object before { get; set; }
}
public class RootObject
{
public string kind { get; set; }
public Data data { get; set; }
}
您是否嘗試過使用以下方法反序列化? 對我來說似乎更強大。
using System.Web.Script.Serialization;
...
var x = new JavaScriptSerializer().Deserialize<Obj_type>(jsonstring);
如purplej0kr所述,您將需要具有.Net模型以用於Obj_type
(並且它將需要與您正在解析的對象匹配),否則將無法正常工作。
您將需要在項目中引用System.Web.Extensions.dll
(右鍵單擊添加引用,dll通常位於“程序集”下)。 否則: 在哪里可以找到程序集System.Web.Extensions dll?
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.