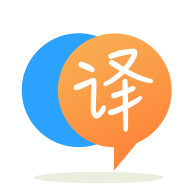
[英]How do I add items to a C# array so that I have exactly 3 of each item?
[英]C# How do I append the previous item in array to the next item in the array for unknown number of items?
對於未知數量的項目,如何將數組中的上一個項目追加到數組中的下一個項目?
這就是我想要做的。 我有一個包含LDAP路徑的字符串,例如“ OU = 3,OU = 2,OU = 1,DC = Internal,DC = Net”,我想在上面的LDAP路徑中創建每個容器,因此需要從上面的字符串開始用下面的內容創建一個數組,這樣我就可以創建每個容器。 在創建第二個等之前,需要先創建第一個數組項。
“OU = 1,DC =內部,DC =凈”
“OU = 2,OU = 1,DC =內部,DC =凈”
“OU = 3,OU = 2,OU = 1,DC =內部,DC =凈”
我的字符串示例只是一個示例,因此路徑可能更長或更短,並且可能包含1個數組項或10+個,我只是不知道,所以我不知道有多少個數組項需要遍歷它們,所以我有數組中的所有路徑。
另一個例子:
從“ OU = Test4,OU = Number3,OU = Item2,OU = 1,DC =內部,DC =凈”中,我需要:
“ OU = 1,DC =內部,DC =凈”“ OU =項目2,OU = 1,DC =內部,DC =凈”“ OU =數字3,OU =項目2,OU = 1,DC =內部,DC =凈“” OU = Test4,OU = Number3,OU = Item2,OU = 1,DC =內部,DC =凈“
感謝您的幫助。
Ĵ
我不確定我是否正確理解。 您是否需要以下內容:
string input = "OU=3,OU=2,OU=1,DC=Internal,DC=Net";
string[] split = input.Split(',');
string path = "";
for (int i=split.Length-1; i>=0; i--)
{
path = ((path == "") ? split[i] : split[i] + "," + path);
if (path.StartsWith("OU"))
DoSomething(path);
}
對於以下字符串,這將調用DoSomething()3次:
如果字符串始終以“,DC = Internal,DC = Net”結尾,則以下解決方案應該可以幫助您。
static string[] Split(string path)
{
const string postfix = ",DC=Internal,DC=Net";
string shortPath = path.Substring(0, path.Length - postfix.Length);
return shortPath.Split(',').Select(x => x + postfix).ToArray();
}
編輯2.0解決方案
static string[] Split(string path)
{
const string postfix = ",DC=Internal,DC=Net";
string shortPath = path.Substring(0, path.Length - postfix.Length);
string[] items = shortPath.Split(',');
List<string> final = new List<string>();
foreach ( string item in items ) {
final.Add(item + postfix);
}
return final.ToArray();
}
這是代碼示例(假設您擁有.NET 3.5):
// First we need to split up the initial string
string[] items = initialString.Split("'");
// Then we filter it so that we have a list of OU and non-OU items
string[] ouItems = items.Where(s=>s.StartsWith("OU=")).ToArray();
string[] nonOuItems = items.Where(s=>!s.StartsWith("OU=")).ToArray();
List<string> mainList = new List<string>();
// Our main loop
for (int i = 0; i < ouItems.Length; i++)
{
List<string> localList = new List<string>();
// We loop through all the previous items including the current one
for (int j = 0; j <= i; j++)
{
localList.Add(ouItems[i]);
}
// Then we add all of the non-OU items
localList.AddRange(nonOuItems);
// Then we build the string itself
bool first = true;
StringBuilder sb = new StringBuilder();
foreach (string s in localList)
{
if (first) first = false;
else sb.Append (","); // Separating the items with commas
sb.Append(s);
}
mainList.Add(sb.ToString());
}
// Now we only have to convert it back to an array
string[] finalArray = mainList.ToArray();
即使您沒有.NET 3.5,也應該非常簡單地將其移植回.NET 2.0。 另外,我還沒有測試過,所以里面可能有錯誤或錯別字。 另外,這絕對不是最有效的方法,但我認為這很明顯並且可能會起作用。
這應該給您一個按正確順序排列的數組,因為它是一個實際的控制台應用程序,所以需要更長的時間:
using System;
using System.Text;
namespace Splitomatic
{
public class Program
{
/// <summary>
/// Sample Input:
/// arg[0]: OU=Test4,OU=Number3,OU=Item2,OU=1
/// arg[1]: DC=Internal,DC=Net
/// </summary>
/// <param name="args"></param>
public static void Main(string[] args)
{
StringBuilder builder;
string[] containers;
string[] parts;
if (args.Length != 2)
{
Console.WriteLine("Usage: <exe> <containers> <append");
}
builder = new StringBuilder(args[1]);
parts = args[0].Split(',');
containers = new string[parts.Length];
for (int i = parts.Length - 1; i >= 0; --i)
{
builder.Insert(0, ",");
builder.Insert(0, parts[i]);
containers[Math.Abs(i - parts.Length + 1)] = builder.ToString();
}
Console.WriteLine("Dumping containers[]:");
for (int i = 0; i < containers.Length; ++i)
{
Console.WriteLine("containers[{0}]: {1}", i, containers[i]);
}
Console.WriteLine("Press enter to quit");
Console.ReadLine();
}
}
}
樣本輸出:
Dumping containers[]: containers[0]: OU=1,DC=Internal,DC=Net containers[1]: OU=Item2,OU=1,DC=Internal,DC=Net containers[2]: OU=Number3,OU=Item2,OU=1,DC=Internal,DC=Net containers[3]: OU=Test4,OU=Number3,OU=Item2,OU=1,DC=Internal,DC=Net Press enter to quit
一種方法。 這也將為每個DC創建一個字符串。
string[] GetArray(string input)
{
string[] vals = input.Split(',');
List<string> entries = new List<string>();
string s;
for(int i=vals.Length - 1; i > 0; i--)
{
s = vals[i];
if(i < vals.Length - 1)
s += "," + entries[(vals.Length - 2) - i];
entries.Add(s);
}
return entries.ToArray();
}
我的代碼幾乎類似於Martin的代碼,但是他的代碼在所有path對象的末尾輸出一個逗號。
這是正確的功能:
static string[] SplitLDAPPath(string input)
{
List<String> r = new List<string>();
string[] split = input.Split(',');
string path = "";
for (int i = split.Length - 1; i >= 0; i--)
{
path = split[i] + "," + path;
if (path.StartsWith("OU"))
r.Add(path.Substring(0, path.Length-1));
}
return r.ToArray();
}
使用:
String s = "OU=Test4,OU=Number3,OU=Item2,OU=1,DC=Internal,DC=Net";
String[] r = SplitLDAPPath(s);
foreach (String ss in r)
lt.Text += ss + "<br/>";
輸出:
OU=1,DC=Internal,DC=Net
OU=Item2,OU=1,DC=Internal,DC=Net
OU=Number3,OU=Item2,OU=1,DC=Internal,DC=Net
OU=Test4,OU=Number3,OU=Item2,OU=1,DC=Internal,DC=Net
如果我正確理解您的問題:
然后,實現應該非常簡單:
public static IEnumerable<String> LastToFirstOrSomething(String s)
{
String[] parts = s.Split(';');
String result = String.Empty;
for (Int32 index = parts.Length - 1; index >= 0; index--)
{
if (result.Length > 0)
result = ";" + result;
result = parts[index] + result;
yield return result;
}
}
當然,可以通過使用StringBuilder在性能方面進行改進。
現在,說了這么多,我知道您的問題與我的答案沒有100%的匹配,因為您還沒有說為什么不提供第一個X元素,所以可以跳過這些。 這是為什么?
您需要提供更多信息以獲得更好的答案。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.