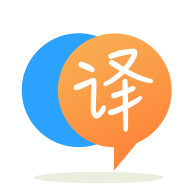
[英]How can i make that when i move the mouse ober pictureBox1 it will draw points automatic on pictureBox2?
[英]How can I make timer that will change the images display in pictureBox1 forward or backward?
在form1頂部,我做了:
private bool farwardbackward;
private FileInfo[] fi;
然后,我有4個按鈕單擊事件:
向前:
private void button4_Click(object sender, EventArgs e)
{
farwardbackward = true;
button5.Enabled = true;
button6.Enabled = true;
timer5.Start();
}
和向后:
private void button3_Click(object sender, EventArgs e)
{
farwardbackward = false;
button5.Enabled = true;
button6.Enabled = true;
if (current == -1)
current = fi.Length;
timer5.Start();
}
並停止動畫按鈕:
private void button6_Click(object sender, EventArgs e)
{
timer5.Stop();
button6.Enabled = false;
button5.Text = "Pause Animation";
button5.Enabled = false;
Bitmap lastdownloadedimage = new Bitmap(fi[fi.Length -1].FullName);
pictureBox1.Image = lastdownloadedimage;
}
最后一個按鈕是暫停/繼續:
private void button5_Click(object sender, EventArgs e)
{
b = (Button)sender;
if (b.Text == "Pause Animation")
{
timer5.Enabled = false;
b.Text = "Continue Animation";
}
else
{
timer5.Enabled = true;
b.Text = "Pause Animation";
}
}
然后在timer5
tick事件中將其間隔設置為1000ms,我這樣做了:
int current = -1;
private void timer5_Tick(object sender, EventArgs e)
{
if (farwardbackward)
current = Math.Min(current + 1, fi.Length - 1);
else
current = Math.Max(current - 1, 0);
if (fi.Length > 0)
{
Bitmap newbmp = new Bitmap(fi[current].FullName);
pictureBox1.Image = newbmp;
pictureBox1.Refresh();
newbmp.Dispose();
}
}
當我運行程序時,我總是在pictureBox1中看到變量fi的最新/最新圖像。 例如,fi中的第一個圖像是000004.gif,最后一個圖像是050122.gif,我看到050122.gif
現在,當使圖像以動畫gif樣式顯示在pictureBox1中時,邏輯應該是什么?
如果是我第一次單擊按鈕前進,那么現在發生的是它跳轉到圖像000004.gif,然后顯示000005.gif,依此類推...
如果是第一次單擊后退按鈕,那么它將從050122.gif返回到050121.gif,依此類推...
問題是當我向后單擊時,在某些圖像后單擊向前按鈕,然后從原來的圖像繼續並向前移動,但是當到達050122.gif時,它停止了。 並且應該繼續不間斷。 想法是使它不停地移動。 如果到達050122.gif,我不希望它停止。 如果要到達第一個圖像或最后一個圖像,則不斷循環播放。 但是由於某種原因,如果在050122.gif上向后移動時單擊前進按鈕,它將停止。
如果我現在沒記錯的話,是當我在中間單擊以更改動畫方向時。 然后,當到達最后一個圖像或第一個圖像時,它停止。 而且我希望它僅在單擊停止按鈕時才繼續不間斷。
另一件事是在停止按鈕單擊事件中我應該做什么? 是否應該重置一些值或變量?
這是timer5滴答事件代碼:
int current = -1;
private void timer5_Tick(object sender, EventArgs e)
{
if (fi.Length > 0)
{
if (farwardbackward)
{
current = (current >= fi.Length - 1) ? 0 : current++;
}
else
{
current = (current <= 0) ? fi.Length - 1 : current--;
}
pictureBox1.Image = new Bitmap(fi[current].FullName);
pictureBox1.Refresh();
}
}
單擊以向前或向后移動時,我遇到相同的異常:索引超出數組的范圍
System.IndexOutOfRangeException was caught
HResult=-2146233080
Message=Index was outside the bounds of the array.
Source=My Weather Station
StackTrace:
at mws.Form1.timer5_Tick(Object sender, EventArgs e) in d:\C-Sharp\Download File\Downloading-File-Project-Version-012\Downloading File\Form1.cs:line 1317
at System.Windows.Forms.Timer.OnTick(EventArgs e)
at System.Windows.Forms.Timer.TimerNativeWindow.WndProc(Message& m)
at System.Windows.Forms.NativeWindow.DebuggableCallback(IntPtr hWnd, Int32 msg, IntPtr wparam, IntPtr lparam)
at System.Windows.Forms.UnsafeNativeMethods.DispatchMessageW(MSG& msg)
at System.Windows.Forms.Application.ComponentManager.System.Windows.Forms.UnsafeNativeMethods.IMsoComponentManager.FPushMessageLoop(IntPtr dwComponentID, Int32 reason, Int32 pvLoopData)
at System.Windows.Forms.Application.ThreadContext.RunMessageLoopInner(Int32 reason, ApplicationContext context)
at System.Windows.Forms.Application.ThreadContext.RunMessageLoop(Int32 reason, ApplicationContext context)
at System.Windows.Forms.Application.Run(Form mainForm)
at mws.Program.Main() in d:\C-Sharp\Download File\Downloading-File-Project-Version-012\Downloading File\Program.cs:line 28
InnerException:
1317行是:
pictureBox1.Image = new Bitmap(fi[current].FullName);
我認為這對於您的tick方法將是更好的代碼:
if(farwardbackward){
current = (current >= fi.Length-1) ? 0 : current++;
}else{
current = (current <= 0) ? fi.Length-1 : current--;
}
picturebox1.Image = new Bitmap(fi[current].Fullname);
picturebox1.Refresh();
除此之外,我會稍微改變兩個按鈕的方法。
@γηράσκωδ'αείπολλάδιδασκόμε:thx,我錯過了這一點
我的解決方案以這種方式很好地工作:
public partial class Form1 : Form
{
private string[] fi;
private int current = 0;
private Boolean forwardbackward = true;
private Boolean timerRunning = true;
public Form1()
{
InitializeComponent();
fi = Directory.GetFiles(@"C://temp/pics");
timer1.Start();
}
private void timer1_Tick(object sender, EventArgs e)
{
if (fi.Length > 0)
{
if (forwardbackward)
{
current = (current >= fi.Length - 1) ? 0 : ++current;
}
else
{
current = (current <= 0) ? fi.Length - 1 : --current;
}
pictureBox1.Image = new Bitmap(fi[current]);
pictureBox1.Refresh();
}
}
private void btn_changeDirection_Click(object sender, EventArgs e)
{
timer1.Stop();
forwardbackward = (forwardbackward) ? false : true;
timer1.Start();
}
private void btn_Pause_Continue_Click(object sender, EventArgs e)
{
if (timerRunning)
{
timer1.Stop();
timerRunning = false;
btn_Pause_Continue.Text = "Continue";
}
else
{
timer1.Start();
timerRunning = true;
btn_Pause_Continue.Text = "Pause";
}
}
private void btn_stop_Click(object sender, EventArgs e)
{
timer1.Stop();
}
}
沒有完美的解決方案,但是它確實可以做到!
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.