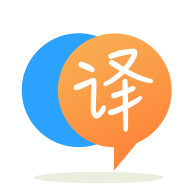
[英]Code First With Existing Database, Entity Framework: Cannot insert explicit value for identity column in table when IDENTITY_INSERT is set to OFF
[英]Entity Framework: Cannot insert explicit value for identity column in table '[table]' when IDENTITY_INSERT is set to OFF
當IDENTITY_INSERT設置為OFF時,無法為表“批量”中的標識列插入顯式值。
我對這個(透明的)明顯的錯誤感到困惑。 BatchId列標記為KEY,在SQL中是自動遞增的PK字段。 當我嘗試分配自己的ID時,出現此錯誤。 當我不分配ID時,會出現此錯誤。 我正在使用EF 6+,從數據庫中以代碼優先樣式反向生成類,沒有任何EDMX文件,只有POCO模型和EntityTypeConfiguration映射類。
我的地圖課程:
public class BatchMap : EntityTypeConfiguration<Batch>
{
public BatchMap()
{
// Primary Key
this.HasKey(t => t.BatchID);
// Properties
this.Property(t => t.BatchDesc)
.HasMaxLength(50);
this.Property(t => t.UserID)
.HasMaxLength(50);
// Table & Column Mappings
this.ToTable("Batch");
this.Property(t => t.BatchID).HasColumnName("BatchID");
this.Property(t => t.VendorID).HasColumnName("VendorID");
this.Property(t => t.BatchDesc).HasColumnName("BatchDesc");
this.Property(t => t.ImportDate).HasColumnName("ImportDate");
this.Property(t => t.ImportMethodID).HasColumnName("ImportMethodID");
this.Property(t => t.BatchTotal).HasColumnName("BatchTotal");
this.Property(t => t.BatchCount).HasColumnName("BatchCount");
this.Property(t => t.StartDateTime).HasColumnName("StartDateTime");
this.Property(t => t.EndDateTime).HasColumnName("EndDateTime");
this.Property(t => t.UserID).HasColumnName("UserID");
}
}
我的POCO課程:
public partial class Batch
{
public decimal BatchID { get; set; }
public Nullable<decimal> VendorID { get; set; }
public string BatchDesc { get; set; }
public Nullable<System.DateTime> ImportDate { get; set; }
public Nullable<decimal> ImportMethodID { get; set; }
public Nullable<decimal> BatchTotal { get; set; }
public Nullable<decimal> BatchCount { get; set; }
public Nullable<System.DateTime> StartDateTime { get; set; }
public Nullable<System.DateTime> EndDateTime { get; set; }
public string UserID { get; set; }
}
最后,上下文定義:
public partial class PaymentProcessingContext : DbContext
{
static PaymentProcessingContext()
{
Database.SetInitializer<PaymentProcessingContext>(null);
}
public PaymentProcessingContext() : base("Name=PaymentProcessingContext")
{
}
public DbSet<AccountLookup> AccountLookups { get; set; }
public DbSet<Batch> Batches { get; set; }
public DbSet<Channel> Channels { get; set; }
public DbSet<Deposit> Deposits { get; set; }
public DbSet<ExceptionType> ExceptionTypes { get; set; }
public DbSet<FeeType> FeeTypes { get; set; }
public DbSet<ImportMethod> ImportMethods { get; set; }
public DbSet<PaymentMethod> PaymentMethods { get; set; }
public DbSet<PaymentTranDetail> PaymentTranDetails { get; set; }
public DbSet<PpaAdmin> PpaAdmins { get; set; }
public DbSet<Preference> Preferences { get; set; }
public DbSet<PrefType> PrefTypes { get; set; }
public DbSet<Return> Returns { get; set; }
public DbSet<SessionData> SessionDatas { get; set; }
public DbSet<SettleBatchReport> SettleBatchReports { get; set; }
public DbSet<Settlement> Settlements { get; set; }
public DbSet<SplitPayment> SplitPayments { get; set; }
public DbSet<TransCode> TransCodes { get; set; }
public DbSet<UTLData> UTLDatas { get; set; }
public DbSet<Vendor> Vendors { get; set; }
public DbSet<VendorRefLookup> VendorRefLookups { get; set; }
public DbSet<Missing_Trans_Key> Missing_Trans_Keys { get; set; }
public DbSet<UnmatchedDeposit> UnmatchedDeposits { get; set; }
protected override void OnModelCreating(DbModelBuilder modelBuilder)
{
modelBuilder.Configurations.Add(new AccountLookupMap());
modelBuilder.Configurations.Add(new BatchMap());
modelBuilder.Configurations.Add(new ChannelMap());
modelBuilder.Configurations.Add(new DepositMap());
modelBuilder.Configurations.Add(new ExceptionTypeMap());
modelBuilder.Configurations.Add(new FeeTypeMap());
modelBuilder.Configurations.Add(new ImportMethodMap());
modelBuilder.Configurations.Add(new PaymentMethodMap());
modelBuilder.Configurations.Add(new PaymentTranDetailMap());
modelBuilder.Configurations.Add(new PpaAdminMap());
modelBuilder.Configurations.Add(new PreferenceMap());
modelBuilder.Configurations.Add(new PrefTypeMap());
modelBuilder.Configurations.Add(new ReturnMap());
modelBuilder.Configurations.Add(new SessionDataMap());
modelBuilder.Configurations.Add(new SettleBatchReportMap());
modelBuilder.Configurations.Add(new SettlementMap());
modelBuilder.Configurations.Add(new SplitPaymentMap());
modelBuilder.Configurations.Add(new TransCodeMap());
modelBuilder.Configurations.Add(new UTLDataMap());
modelBuilder.Configurations.Add(new VendorMap());
modelBuilder.Configurations.Add(new VendorRefLookupMap());
modelBuilder.Configurations.Add(new Missing_Trans_KeyMap());
modelBuilder.Configurations.Add(new UnmatchedDepositMap());
}
}
在SQL中,該表定義為:
USE [PaymentProcessing]
GO
/****** Object: Table [dbo].[Batch] Script Date: 11/13/2014 10:30:06 AM ******/
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
SET ANSI_PADDING ON
GO
CREATE TABLE [dbo].[Batch](
[BatchID] [numeric](18, 0) IDENTITY(1,1) NOT NULL,
[VendorID] [numeric](18, 0) NULL,
[BatchDesc] [varchar](50) NULL,
[ImportDate] [datetime] NULL,
[ImportMethodID] [numeric](18, 0) NULL,
[BatchTotal] [numeric](18, 2) NULL,
[BatchCount] [numeric](18, 0) NULL,
[StartDateTime] [datetime] NULL,
[EndDateTime] [datetime] NULL,
[UserID] [varchar](50) NULL,
CONSTRAINT [PK_Batch] PRIMARY KEY CLUSTERED
(
[BatchID] ASC
)WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY]
) ON [PRIMARY]
GO
SET ANSI_PADDING OFF
GO
我看不到任何FK限制。 (看到FK在另一個SO問題中引起了一些問題。)
現在,我的代碼:
public Batch InsertNewBatchRecord(int vendorId)
{
Batch batch;
using (var uow = new UnitOfWorkPaymentProcessingEf())
{
var repVendor = new RepositoryVendor(uow);
var repBatch = new RepositoryBatch(uow);
var vendor = repVendor.GetById(vendorId); // get vendor info...
var maxId = repBatch.NewId(); // Get next BatchId...
// Create full description...
var desc = vendor != null
? string.Format("{0}", vendor.VendorLongName)
: string.Format("Could not find vendor for ID [{0}]", vendorId);
desc = string.Format("{0} - {1} : {2}", desc, DateTime.Now, maxId);
// Create new batch record
batch = new Batch
{
//BatchId = maxId, <------ DOES NOT MATTER IF COMMENTED OUT OR NOT - same error both ways!
VendorID = vendorId,
BatchDesc = desc,
ImportDate = DateTime.Now,
ImportMethodID = 1,
UserID = Environment.UserName
};
repBatch.Insert(batch);
uow.Commit(); // <----- THIS FAILS!!! Internally it calls SaveChanges() only.
}
return batch;
}
任何想法為什么甚至會引發錯誤?
聽起來像缺少HasDatabaseGeneratedOption
。 你應該有:
this.Property(t => t.BatchID).
HasDatabaseGeneratedOption(DatabaseGeneratedOption.Identity);
順便說一句:[ decimal
| numeric
]聽起來很奇怪。 通常使用int
或bigint
。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.