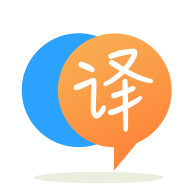
[英]C# - How do I set the selected item in a combobox by comparing my int value?
[英]How do I set the selected item in a comboBox to match my string using C#?
我有一個字符串“test1”,我的 comboBox 包含test1
、 test2
和test3
。 如何將所選項目設置為“test1”? 也就是說,如何將我的字符串與 comboBox 項之一匹配?
我正在考慮下面的行,但這行不通。
comboBox1.SelectedText = "test1";
這應該可以解決問題:
Combox1.SelectedIndex = Combox1.FindStringExact("test1")
假設您的組合框不是數據綁定的,您需要在表單的“items”集合中找到對象的索引,然后將“selectedindex”屬性設置為適當的索引。
comboBox1.SelectedIndex = comboBox1.Items.IndexOf("test1");
請記住,如果未找到該項目,IndexOf 函數可能會拋出參數異常。
如果您的 ComboBox 中的項目是字符串,您可以嘗試:
comboBox1.SelectedItem = "test1";
對我來說,這只有效:
foreach (ComboBoxItem cbi in someComboBox.Items)
{
if (cbi.Content as String == "sometextIntheComboBox")
{
someComboBox.SelectedItem = cbi;
break;
}
}
MOD:如果您有自己的對象作為組合框中設置的項目,則將 ComboBoxItem 替換為其中之一,例如:
foreach (Debitor d in debitorCombo.Items)
{
if (d.Name == "Chuck Norris")
{
debitorCombo.SelectedItem = d;
break;
}
}
ComboBox1.SelectedIndex= ComboBox1.FindString("Matching String");
在 Windows 窗體中試試這個。
SelectedText是獲取或設置字符串編輯所選項目的實際文本在下拉列表作為記錄在這里。 如果您設置,這將不可編輯:
comboBox1.DropDownStyle = System.Windows.Forms.ComboBoxStyle.DropDownList;
采用:
comboBox1.SelectedItem = "test1";
要么:
comboBox1.SelectedIndex = comboBox1.Items.IndexOf("test1");
我使用了一種擴展方法:
public static void SelectItemByValue(this ComboBox cbo, string value)
{
for(int i=0; i < cbo.Items.Count; i++)
{
var prop = cbo.Items[i].GetType().GetProperty(cbo.ValueMember);
if (prop!=null && prop.GetValue(cbo.Items[i], null).ToString() == value)
{
cbo.SelectedIndex = i;
break;
}
}
}
然后只需使用該方法:
ddl.SelectItemByValue(value);
comboBox1.SelectedItem.Text = "test1";
假設 test1、test2、test3 屬於 comboBox1 集合,下面的語句將起作用。
comboBox1.SelectedIndex = 0;
我已經用從數據庫中填充的 een DataTable 填充了我的 ComboBox。 然后我設置了 DisplayMember 和 ValueMember。 我使用此代碼來設置所選項目。
foreach (DataRowView Row in ComboBox1.Items)
{
if (Row["ColumnName"].ToString() == "Value") ComboBox1.SelectedItem = Row;
}
該解決方案基於MSDN,並進行了一些修改。
它找到字符串的精確或部分並設置它。
private int lastMatch = 0; private void textBoxSearch_TextChanged(object sender, EventArgs e) { // Set our intial index variable to -1. int x = 0; string match = textBoxSearch.Text; // If the search string is empty set to begining of textBox if (textBoxSearch.Text.Length != 0) { bool found = true; while (found) { if (comboBoxSelect.Items.Count == x) { comboBoxSelect.SelectedIndex = lastMatch; found = false; } else { comboBoxSelect.SelectedIndex = x; match = comboBoxSelect.SelectedValue.ToString(); if (match.Contains(textBoxSearch.Text)) { lastMatch = x; found = false; } x++; } } } else comboBoxSelect.SelectedIndex = 0; }
我希望我有所幫助!
我使用KeyValuePair進行 ComboBox 數據綁定,我想按值查找項目,因此這在我的情況下有效:
comboBox.SelectedItem = comboBox.Items.Cast<KeyValuePair<string,string>>().First(item=> item.Value == "value to match");
但是如果我作為代碼審查者看到這樣的代碼,我會建議重新考慮所有方法算法。
您在 ComboBox 中沒有該屬性。 您有 SelectedItem 或 SelectedIndex。 如果您有用於填充組合框的對象,則可以使用 SelectedItem。
如果不是,您可以獲取項目集合(屬性項目)並對其進行迭代,直到獲得所需的值並將其與其他屬性一起使用。
希望能幫助到你。
_cmbTemplates.SelectedText = "test1"
或者可能
_cmbTemplates.SelectedItem= _cmbTemplates.Items.Equals("test1");
在組合框(包含 MyObjects 列表)中找到 mySecondObject(MyObject 類型)並選擇項目:
foreach (MyObject item in comboBox.Items)
{
if (item.NameOrID == mySecondObject.NameOrID)
{
comboBox.SelectedItem = item;
break;
}
}
設置 ComboBox 項的所有方法、技巧和代碼行將無法工作,直到 ComboBox 具有父項。
我創建了一個函數,它將返回值的索引
public static int SelectByValue(ComboBox comboBox, string value)
{
int i = 0;
for (i = 0; i <= comboBox.Items.Count - 1; i++)
{
DataRowView cb;
cb = (DataRowView)comboBox.Items[i];
if (cb.Row.ItemArray[0].ToString() == value)// Change the 0 index if your want to Select by Text as 1 Index
{
return i;
}
}
return -1;
}
這對我有用.....
comboBox.DataSource.To<DataTable>().Select(" valueMember = '" + valueToBeSelected + "'")[0]["DislplayMember"];
我知道這不是 OP 所問的,但他們可能不知道嗎? 這里已經有幾個答案,所以即使這很長,我認為它可能對社區有用。
使用枚舉填充組合框允許輕松使用 SelectedItem 方法以編程方式選擇組合框中的項目以及從組合框中加載和讀取。
public enum Tests
{
Test1,
Test2,
Test3,
None
}
// Fill up combobox with all the items in the Tests enum
foreach (var test in Enum.GetNames(typeof(Tests)))
{
cmbTests.Items.Add(test);
}
// Select combobox item programmatically
cmbTests.SelectedItem = Tests.None.ToString();
如果雙擊組合框,您可以處理選定的索引更改事件:
private void cmbTests_SelectedIndexChanged(object sender, EventArgs e)
{
if (!Enum.TryParse(cmbTests.Text, out Tests theTest))
{
MessageBox.Show($"Unable to convert {cmbTests.Text} to a valid member of the Tests enum");
return;
}
switch (theTest)
{
case Tests.Test1:
MessageBox.Show("Running Test 1");
break;
case Tests.Test2:
MessageBox.Show("Running Test 2");
break;
case Tests.Test3:
MessageBox.Show("Running Test 3");
break;
case Tests.None:
// Do nothing
break;
default:
MessageBox.Show($"No support for test {theTest}. Please add");
return;
}
}
然后,您可以從按鈕單擊處理程序事件運行測試:
private void btnRunTest1_Click(object sender, EventArgs e)
{
cmbTests.SelectedItem = Tests.Test1.ToString();
}
ListItem li = DropDownList.Items.FindByValue("13001");
DropDownList.SelectedIndex = ddlCostCenter.Items.IndexOf(li);
對於您的情況,您可以使用
DropDownList.Items.FindByText("Text");
如果您通過數據集綁定數據源,那么您應該使用“SelectedValue”
cmbCategoryList.SelectedValue = (int)dsLookUp.Tables[0].Select("WHERE PRODUCTCATEGORYID = 1")[0]["ID"];
combo.Items.FindByValue("1").Selected = true;
你可以說comboBox1.Text = comboBox1.Items[0].ToString();
請嘗試這種方式,它對我有用:
Combobox1.items[Combobox1.selectedIndex] = "replaced text";
它應該工作
Yourcomboboxname.setselecteditem("yourstring");
如果你想設置數據庫字符串使用這個
Comboboxname.setselecteditem(ps.get string("databasestring"));
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.