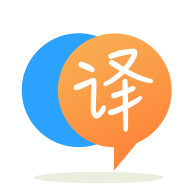
[英]Convert IEnumerable<Dictionary<int, string>> to List<Dictionary<int, string>>
[英]Convert dictionary with List to IEnumerable
我有一本字典:
Dictionary<String, List<Foo>> test = new Dictionary<String, List<Foo>>();
然后我填充這個字典,因此我需要列表,所以我可以調用Add()。 我的問題是函數需要返回:
Dictionary<String, IEnumerable<Foo>>
是否有任何簡單的方法可以做到這一點,而不是通過我的原始字典並手動完成顯而易見的循環?
return dictionary.ToDictionary(x => x.Key,x => x.Value.AsEnumerable())
使用List<Foo>
添加內容但將其添加到Dictionary<String, IEnumerable<Foo>>
更高效,更容易。 這是沒有問題,因為List<Foo>
實現IEnumerable<Foo>
,它甚至不需要強制轉換。
這樣的事情(偽代碼):
var test = new Dictionary<String, IEnumerable<Foo>>();
foreach(var x in something)
{
var list = new List<Foo>();
foreach(var y in x.SomeCollection)
list.Add(y.SomeProperty);
test.Add(x.KeyProperty, list); // works since List<T> is also an IEnumerable<T>
}
我也嘗試了這條路線,將Dictionary<string, List<Foo>>
轉換為ReadOnlyDictionary<string, IEnumerable<Foo>>
。 當我試圖轉換為只讀字典時,將List
轉換為IEnumerable
的整個目的是創建一個只讀集合。 OP的方法存在的問題是:
Dictionary<string, List<string>> errors = new Dictionary<string, List<string>>();
errors["foo"] = new List<string>() { "You can't do this" };
Dictionary<string, IEnumerable<string>> readOnlyErrors = // convert errors...
readOnlyErrors["foo"] = new List<string>() { "I'm not actually read-only!" };
IEnumerable<Foo>
讓你覺得這是只讀且安全的,而實際上並非如此。 在閱讀問題LINQ Convert Dictionary to Lookup a Lookup
對象之后更合適,因為它允許您:
將一個鍵與多個值相關聯
您無法使用新值覆蓋密鑰
// This results in a compiler error lookUp["foo"] = new List<Foo>() { ... };
“多個值”已經定義為IEnumerable<T>
您仍然可以使用相同的外部和內部循環算法來提取單個值:
ILookup<string, string> lookup = // Convert to lookup foreach (IGrouping<string, string> grouping in lookup) { Console.WriteLine(grouping.Key + ":"); foreach (string item in grouping) { Console.WriteLine(" item: " + item); } }
Dictionary<string, List<Foo>>
為ILookup<string, Foo>
這是一個快速的雙線程:
Dictionary<string, List<Foo>> foos = // Create and populate 'foos'
ILookup<string, Foo> lookup = foos.SelectMany(item => item.Value, Tuple.Create)
.ToLookup(p => p.Item1.Key, p => p.Item2);
現在,您可以使用與Dictionary<string, IEnumerable<Foo>>
相同的兩步循環:
foreach (IGrouping<string, Foo> grouping in lookup)
{
string key = grouping.Key;
foreach (Foo foo in grouping)
{
// Do stuff with key and foo
}
}
來源: LINQ將字典轉換為查找
轉換為具有IEnumerable值的另一個詞典就像嘗試將方形釘插入圓孔中一樣。 更合適,更安全的方法(從面向對象的角度來看)是將讀/寫字典轉換為查找。 這為您提供了只讀對象的真正安全性( Foo
項除外,它們可能不是不可變的)。
我甚至會說,大多數時候使用ReadOnlyDictionary
,你可以使用ILookup
並獲得相同的功能。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.