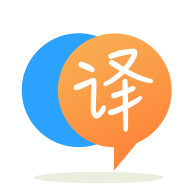
[英]Accessing an object's array of objects attribute gives attribute error in python
[英]Accessing list of Python objects by object attribute
我不知道我試圖做的是不是Pythonic,我只是試圖做錯,或者我不知道如何正確地提出這個問題。 能夠做到這一點對我來說是有意義的,但我已經搜索了15種不同的方法,但找不到答案。
我想做的事情看起來很簡單:我有一個對象列表。 我想通過對象的屬性訪問該列表。 此代碼有效:
class Fruit:
def __init__(self, name, color):
self.name = name
self.color = color
def __str__(self):
return self.name
class BaseballPlayer:
def __init__(self, name, number, position):
self.name = name
self.number = number
self.position = position
def __str__(self):
return self.name
class ListByProperty(list):
def __init__(self, property, list):
super(ListByProperty, self).__init__(list)
self.property = property
def __getitem__(self, key):
return [item for item in self if getattr(item, self.property) == key][0]
fruits = ListByProperty('name', [Fruit('apple', 'red'), Fruit('banana', 'yellow')])
baseballTeam = ListByProperty('position', [BaseballPlayer('Greg Maddux', 31, 'P'),
BaseballPlayer('Javy Lopez', 8, 'C')])
teamByNumber = ListByProperty('number', baseballTeam)
print 'Apples are', fruits['apple'].color
pitcher = baseballTeam['P']
print 'The pitcher is #%s, %s' % (pitcher.number, pitcher)
print '#8 is', teamByNumber[8]
>>> Apples are red
The pitcher is #31, Greg Maddux
#8 is Javy Lopez
但是,我真的必須讓我自己的列表類做這么簡單的事情嗎? 除了循環或listcomp之外,沒有通用的方法嗎? 這似乎應該是一個非常常見的事情,通過對象的屬性獲得列表中的對象和訪問項。 它似乎應該以類似於sorted(key=...)
的方式得到普遍支持。
請注意,這與需要dict的情況不同。 實際上,使用對象列表而不是字典的全部意義在於避免必須執行以下操作:
fruits = {'apple': Fruit('apple', 'red')}
...這需要你輸入兩次apple
。 看起來應該有一種通用的方法來做這樣的事情:
print 'Apples are', fruits['apple'].color
...無需子類list
。
好吧,你可以建立一個像這樣的字典:
fruits = [Fruit('apple', 'red'), Fruit('banana', 'yellow')]
fruits = {f.name: f for f in fruits}
或者你可以單行,但看起來仍然......呃......語法上是酸的? :)
我到目前為止最好的解決方法是:
class DictByProperty(dict):
def __init__(self, property, list):
super(DictByProperty, self).__init__({getattr(i, property): i for i in list})
self.property = property
fruits = DictByProperty('name', [Fruit('apple', 'red')])
哦,謝謝,我已經從這個問題中學到了很多東西。
class Fruit:
def __init__(self, name, color):
self.name = name
self.color = color
fruits = dict(zip(['apple', 'banana'], [Fruit('apple', 'red'), Fruit('banana', 'yellow')]))
print("An apple is %s" % fruits['apple'].color)
要么:
fruits = {fruit.name : fruit for fruit in [Fruit('apple', 'red'), Fruit('banana', 'yellow')]}
print("An apple is %s" % fruits['apple'].color)
以下確實產生了一組:
fruits = {Fruit('apple', 'red'), Fruit('banana', 'yellow')}
注意與我創建dict的方式的區別
fruits = [Fruit('apple', 'red'), Fruit('banana', 'yellow')]
print("An apple is %s" % fruits[fruits.index('apple')].color)
不起作用,因為你的列表包含水果類型的對象而不是字符串,這是相同的故事:
fruits = FruitList([Fruit('apple', 'red'), Fruit('banana', 'yellow')])
print("An apple is %s" % fruits['apple'].color)
要使上述方法有效,請執行以下操作:
class Fruit:
def __init__(self, name, color):
self.name = name
self.color = color
def __eq__(self, other):
if isinstance(other, Fruit):
return (self.name, self.color) == (other.name, other.color)
return self.name == other
fruits = [Fruit('apple', 'red'), Fruit('banana', 'yellow')]
print("An apple is %s" % fruits[fruits.index('apple')].color)
我不推薦這個,因為在你的列表恰好包含字符串apple
的情況下,對color
屬性調用變得無效,因為字符串沒有名為color
的屬性
您可以創建一個實例。
apple = Fruit('apple', 'red')
print(apple.color) # I use Python 3.x
我不確定我是在關注你的問題。 但也許這會有所幫助。
編輯:試圖獲得我的聲譽...
你可以在字典中使用WITHIN實例。 例如...
banana = Fruit('banana', 'yellow')
apple = Fruit('apple', 'red')
fruits = {'apple':apple, 'banana':banana}
或者如果您願意:
fruits = {'apple':Fruit('apple', 'red'), 'banana':Fruit('banana', 'yellow')}
無論哪種方式,您都可以簡單地調用水果的顏色
print(fruits['banana'].color)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.