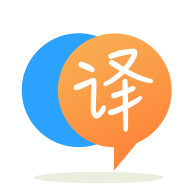
[英]How to mock a method with CancellationToken passed as parameter?
[英]How to restrict passed parameter in method
我的問題在示例代碼中。 我如何限制開發人員傳遞真實參數。 我嘗試了一些有關泛型的方法,但無法解決。
這里重要的是我想限制編譯時間。 所以我知道如何在運行時中進行預防。
namespace TheLiving
{
public interface IFood
{
int Protein { get; set; }
int Carbohydrate { get; set; }
}
public interface IMeat : IFood
{
int Nitrogen { get; set; }
}
public interface IVegetable : IFood
{
int Vitamin { get; set; }
}
public class Veal : IMeat
{
public int Protein { get; set; }
public int Carbohydrate { get; set; }
public int Nitrogen { get; set; }
}
public class Spinach : IVegetable
{
public int Protein { get; set; }
public int Carbohydrate { get; set; }
public int Vitamin { get; set; }
}
public interface IEating
{
void Eat(IFood food);
}
public class Lion : IEating
{
public int Protein { get; set; }
public int Carbohydrate { get; set; }
public int Nitrogen { get; set; }
//But lion is eating only Meat. So any developer can pass vegatable to lion for eating.
//May be god is not a good developer. So i want restrict him on Compile Time!! for passing only Meat. :)
//The important thing here is i want restrict on Compile Time not RunTime!!!
public void Eat(IFood food)
{
Protein = food.Protein;
Carbohydrate = food.Carbohydrate;
//Nitrogen = ?? //So i know that i can cast and validate food but i want ensure this on DesignTime!!
}
}
public class Sheep : IEating
{
public int Protein { get; set; }
public int Carbohydrate { get; set; }
public int Vitamin { get; set; }
public void Eat(IFood food)
{
Protein = food.Protein;
Carbohydrate = food.Carbohydrate;
//Vitamin = food.??
}
}
}
我認為您必須具有IHerbivore
, ICarnivore
和IOmnivore
才能在設計時允許它。
public interface IHerbivore
{
void Eat(IVegetable food);
}
public interface ICarnivore
{
void Eat(IMeat food);
}
public interface IOmnivore : IHerbivore, ICarnivore
{
}
這樣,您的獅子可以成為ICarnivore
,只能吃肉
或者,您可以更改界面...
public interface IEating
{
bool Eat(IFood food); //return wheater the eater eats the food or not
}
像這樣實現獅子:
public class Lion : IEating
{
public int Protein { get; set; }
public int Carbohydrate { get; set; }
public int Nitrogen { get; set; }
public bool Eat(IFood food)
{
IMeat meat = food as IMeat;
if (meat != null)
{
Protein = meat.Protein;
Carbohydrate = meat.Carbohydrate;
Nitrogen = meat.Nitrogen;
return true;
}
return false;
}
}
public interface IEating<in T> where T : IFood {
void Eat(T food);
}
public class Lion : IEating<IMeat>, IEating<IFood> {
public int Protein { get; set; }
public int Carbohydrate { get; set; }
public int Nitrogen { get; set; }
public void Eat(IMeat food) {
Protein = food.Protein;
Carbohydrate = food.Carbohydrate;
Nitrogen = food.Nitrogen;
}
public void Eat(IFood food) {
var meat = food as IMeat;
if (meat == null) return;
Eat(meat);
}
}
public class Sheep : IEating<IVegetable>, IEating<IFood> {
public int Protein { get; set; }
public int Carbohydrate { get; set; }
public int Vitamin { get; set; }
public void Eat(IVegetable food) {
Protein = food.Protein;
Carbohydrate = food.Carbohydrate;
Vitamin = food.Vitamin;
}
public void Eat(IFood food) {
var vegetable = food as IVegetable;
if (vegetable == null) return;
Eat(vegetable);
}
}
MS代碼合同呢? 我發誓它的Visual Studio集成至少在編譯時添加警告。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.