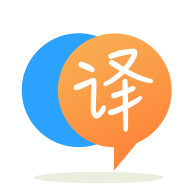
[英]Winforms textbox allow user to input only letters, numbers, dots and spaces between words. Regex
[英]WinForms Textbox only allow numbers between 1 and 6
我想限制我的 WinForms 文本框,以便它只允許輸入 1 到 6 之間的數字。 沒有字母,沒有其他符號或特殊字符,只有那些數字。
我怎么做?
你可以把它放在文本框的KeyPress
事件上,
private void textBox3_KeyPress(object sender, KeyPressEventArgs e)
{
switch (e.KeyChar)
{
//allowed keys 1-6 + backspace and delete + arrowkeys
case (char)Keys.Back:
case (char)Keys.Delete:
case (char)Keys.D0:
case (char)Keys.D1:
case (char)Keys.D2:
case (char)Keys.D3:
case (char)Keys.D4:
case (char)Keys.D5:
case (char)Keys.D6:
case (char)Keys.Left:
case (char)Keys.Up:
case (char)Keys.Down:
case (char)Keys.Right:
break;
default:
e.Handled = true;
break;
}
}
如果按下的鍵是 (numpad) 1-6 ,則退格鍵、刪除鍵或箭頭鍵允許它們。 如果它是不同的鍵,請不要放置它並說它已處理。 我在一個快速項目中測試了這段代碼,它允許我使用小鍵盤 1-6 放置,但不知道其他數字。 如果其他人不起作用,您只需將它們添加為允許的並且不測試箭頭鍵,但只需要左右移動即可。
添加限制: 0到6並使用它:
private void txtField_KeyPress(object sender, KeyPressEventArgs e)
{
if (!char.IsControl(e.KeyChar) && !char.IsDigit(e.KeyChar) && (e.KeyChar != '.'))
e.Handled = true;
if ((e.KeyChar == '.') && ((sender as TextBox).Text.IndexOf('.') > -1))
e.Handled = true;
}
以下正則表達式應該可以工作
Regex rgx = new Regex(@"^[1-6]+$");
rgx.IsMatch("1a23"); //Returns False
rgx.IsMatch("1234"); //Returns True;
您應該能夠將它與 ASP.Net Validations 和 WinForms 一起使用
https://msdn.microsoft.com/en-us/library/3y21t6y4(v=vs.110).aspx
https://msdn.microsoft.com/en-us/library/az24scfc%28v=vs.110%29.aspx
請記住,我建議在提交值后進行驗證,這可能不是您想要的。 對我來說,這里的問題是模棱兩可的。 假設您正在使用 ASP.Net 並且您想要限制輸入值,那么先前答案的按鍵事件解決方案應該可以工作,但它們至少需要部分回發。 如果您希望在沒有回發的情況下處理此問題,則需要一個客戶端腳本。
這是如何在客戶端執行此操作的示例:
由於該示例適用於任何數字,因此您必須將其限制為數字 1 - 6。
在這里,您有一個簡單的 WebForm C#,在提交時隱藏了代碼,它將驗證 0-6 之間數字的正則表達式。
HTML
<div class="jumbotron">
<h1>ASP.NET</h1>
<p class="lead">
<asp:TextBox runat="server" ID="tbForValidation"></asp:TextBox>
<asp:Button runat="server" ID="btnSubmit" Text="Validate" OnClick="btnSubmit_Click" />
</p>
</div>
后面的代碼:
protected void btnSubmit_Click(object sender, EventArgs e)
{
Regex regularExpression = new Regex(@"^[0-6]$");
if (regularExpression.IsMatch(tbForValidation.Text))
{
//Is matching 0-6
}
else
{
//Is not matching 0-6
}
}
我還建議您在向服務器發送請求之前在客戶端運行 RegEx 驗證。 這不會向服務器創建任何不必要的請求,以便對文本框進行簡單驗證。
<asp:RegularExpressionValidator ID="RegularExpressionValidator1" runat="server" ErrorMessage="Invalid number" ValidationExpression="^[0-6]$" ControlToValidate="tbForValidation"></asp:RegularExpressionValidator>
你試過 SupressKeyPress 嗎?
if (e.KeyCode < Keys.D1 || e.KeyCode > Keys.D6 || e.Shift || e.Alt)
{
e.SuppressKeyPress = true;
}
if (e.KeyCode == Keys.Back)
{
e.SuppressKeyPress = false;
}
如果您想更改您所寫的內容,則第二個 If 使您能夠按退格鍵。
我會做這樣的事情
private void textBox1_KeyDown(object sender, KeyEventArgs e)
{
if (e.KeyCode == Keys.D1 || e.KeyCode == Keys.D2 || e.KeyCode == Keys.D3 || e.KeyCode == Keys.D4 || e.KeyCode == Keys.D5 || e.KeyCode == Keys.D6)
{
e.Handled = true;
}
}
您也可以允許使用控制按鈕,例如 Backspace
嘗試這個:
private void textBox1_TextChanged(object sender, EventArgs e)
{
int tester = 0;
try
{
if (textBox1.Text != null)
{
tester = Convert.ToInt32(textBox1.Text);
if (tester >= 30)
{
textBox1.Text = textBox1.Text.Substring(0, textBox1.Text.Length - 1);
textBox1.Select(textBox1.Text.Length, 0);
}
}
}
catch (Exception)
{
if (textBox1.Text != null)
{
try
{
if (textBox1.Text != null)
{
textBox1.Text = textBox1.Text.Substring(0, textBox1.Text.Length - 1);
textBox1.Select(textBox1.Text.Length, 0);
}
}
catch (Exception)
{
textBox1.Text = null;
}
}
}
}
使用正則表達式 @"^[1-6]$" 來驗證文本框中的此類輸入。
這會將您限制為 1 - 6。我不知道您想如何使用它。 因此,我只是給你提供限制的代碼。 這是非常古老的,更像是平民。
首先,我做了一個表格:
然后我在按鈕中添加了以下代碼:
int testValue;
// only numbers will drop into this block
if(int.TryParse(textBox1.Text, out testValue)){
// hard coded test for your desired values
if(testValue == 1 || testValue == 2 || testValue == 3 || testValue == 4 || testValue == 5 || testValue == 6){
// this is here just to give us proof of the return
textBox1.Text = "Yep, 1 - 6";
}
else{
// you can throw an exception here, popup a message, or populate as I did here.
// this is here just to give us proof of the return
textBox1.Text = "Nope, not 1 - 6";
}
}
// you can throw an exception here, popup a message, or populate as I did here.
else{
textBox1.Text = "Not a number";
}
如果您輸入任何非數字,文本框會顯示“不是數字”。
1 - 6 將讀作“是的,1 - 6”。
任何數字 < 或 > 1 - 6 將讀作“不,不是 1 - 6”。
祝你好運!
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.