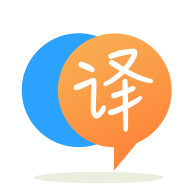
[英]System.Data.SqlClient.SqlException: 'Conversion failed when converting date and/or time from character string.'
[英]SqlException: Conversion failed when converting date and/or time from character string
使用“添加”用戶信用卡詳細信息的C#網絡表單時出現此錯誤。 以下是我的aspx.cs頁面上的“添加信用卡”按鈕的代碼
protected void Button1_Click(object sender, EventArgs e)
{
//declare and initialize connection object to connect to the database
SqlConnection conn = new SqlConnection(
WebConfigurationManager.ConnectionStrings["DefaultConnection"].ConnectionString);
SqlCommand cmd; //declare a command object that will be used to send commands to database.
conn.Open(); //open a connection to the database
cmd = conn.CreateCommand(); //create a command object
cmd.CommandText = "Insert into CreditCard Values ('" +
txtCCNo.Text + "', '" +
txtFName.Text + "', '" +
txtMidInitial.Text + "', '" +
txtLName.Text + "', '" +
txtExpirationDate.Text + "', '" + txtType.Text + "', " +
txtCVC.Text + ", '" + txtIsDefault.Text + "', '" +
Session["userID"].ToString() + "')";
cmd.CommandType = CommandType.Text;
cmd.ExecuteNonQuery();
conn.Close();
// added for navigation
Response.Redirect("~/selectCC.aspx");
}
以下是.aspx主頁
<%@ Page Title="" Language="C#" MasterPageFile="~/Site.master" AutoEventWireup="true" CodeFile="AddCC.aspx.cs" Inherits="AddCC" %>
<table style="width: 100%;">
<tr>
<td> <asp:Label ID="Label1" runat="server" Text="First Name"></asp:Label></td>
<td> <asp:TextBox ID="txtFName" runat="server"></asp:TextBox></td>
<td> <asp:RequiredFieldValidator ID="RequiredFieldValidator1" runat="server" ErrorMessage="First Name is Required" ControlToValidate="txtFName"></asp:RequiredFieldValidator></td>
</tr>
<tr>
<td> <asp:Label ID="Label2" runat="server" Text="Last Name"></asp:Label></td>
<td> <asp:TextBox ID="txtLName" runat="server"></asp:TextBox></td>
<td> <asp:RequiredFieldValidator ID="RequiredFieldValidator2" runat="server" ErrorMessage="Last Name is Required" ControlToValidate="txtLName"></asp:RequiredFieldValidator></td>
</tr>
<tr>
<td> <asp:Label ID="Label3" runat="server" Text="Middle Initial"></asp:Label></td>
<td> <asp:TextBox ID="txtMidInitial" runat="server" MaxLength="1"></asp:TextBox></td>
<td> </td>
</tr>
<tr>
<td> <asp:Label ID="Label4" runat="server" Text="Credit Card No"></asp:Label></td>
<td> <asp:TextBox ID="txtCCNo" runat="server"></asp:TextBox></td>
<td> <asp:RequiredFieldValidator ID="RequiredFieldValidator4" runat="server" ErrorMessage="Credit Card is Required" ControlToValidate="txtCCNo"></asp:RequiredFieldValidator></td>
</tr>
<tr>
<td> <asp:Label ID="Label5" runat="server" Text="Expiration Date"></asp:Label></td>
<td> <asp:TextBox ID="txtExpirationDate" runat="server"></asp:TextBox></td>
<td> <asp:RequiredFieldValidator ID="RequiredFieldValidator3" runat="server" ErrorMessage="Expiration Date is Required" ControlToValidate="txtExpirationDate"></asp:RequiredFieldValidator></td>
</tr>
<tr>
<td> <asp:Label ID="Label6" runat="server" Text="CVC"></asp:Label></td>
<td> <asp:TextBox ID="txtCVC" runat="server"></asp:TextBox></td>
<td> <asp:RequiredFieldValidator ID="RequiredFieldValidator5" runat="server" ErrorMessage="CVC is Required" ControlToValidate="txtCVC"></asp:RequiredFieldValidator></td>
</tr>
<tr>
<td> <asp:Label ID="Label7" runat="server" Text="Type"></asp:Label></td>
<td> <asp:TextBox ID="txtType" runat="server"></asp:TextBox></td>
<td> <asp:RequiredFieldValidator ID="RequiredFieldValidator6" runat="server" ErrorMessage="Type is Required" ControlToValidate="txtType"></asp:RequiredFieldValidator></td>
</tr>
<tr>
<td> <asp:Label ID="Label8" runat="server" Text="Default"></asp:Label></td>
<td> <asp:TextBox ID="txtIsDefault" runat="server"></asp:TextBox></td>
<td> </td>
</tr>
<tr>
<td></td>
<td>
<asp:Button ID="Button1" runat="server" Text="Add Credit Card" OnClick="Button1_Click" OnCommand="Button1_Command" /></td>
<td></td>
</tr>
</table>
我相信該錯誤即將到來,因為ExpirationDate具有DateTime數據類型,但不確定如何在aspx.cs代碼中指定此類型,以便由SQL代碼處理。
正確的方法是對插入使用參數化查詢,並使用SqlParameter類指定參數值。 它還將處理不同語言的日期格式。 並且可以保護您免受SQL注入的侵害,
// 1. declare command object with parameter
SqlCommand cmd = new SqlCommand("select * from Customers where city = @City", conn);
// 2. define parameters used in command object
SqlParameter param = new SqlParameter();
param.ParameterName = "@City";
param.Value = inputCity;
記得:
首先,即使您正在執行此動態SQL創建,也要始終嘗試使用參數,它將保護您免受SQL注入的侵害。
其次,嘗試首先獲取txtExpirationDate.Text的值,然后將其強制轉換為DateTime。 嘗試使用標准格式將值發送到sql Query中,這可能是無法識別字符串格式(例如,發送類似“ 2015/03/03”的內容)。
看一下DateTime.Parse()
和DateTime.ParseExact()
方法,了解將字符串解析為日期的方法。
人們所說的有關SQL注入的內容非常重要。 如果您只是通過一個接一個的添加一串字符串來構造自己的sql查詢,那么如果人們在輸入sql查詢的控件中鍵入精心制作的字符串,那么勢必會受到討厭的攻擊。 @Oscar的評論談到了這種風險(有人可以輸入一個值,這會導致整個數據庫的刪除),他的回答向您展示了如何使用參數。
SqlParameter
機制可以防止這種情況,因為在將參數值替換為@Parameter占位符的查詢字符串中時,有一些代碼會在替換之前先仔細清理參數值。
如果您有任何更安全的選擇,則基本上根本不需要通過串聯構造自己的sql字符串。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.