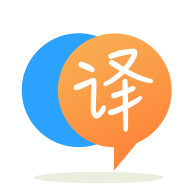
[英]C++: Strange virtual member function pointers in Visual Studio 2013
[英]Wrapping C++ member function in C - Visual Studio 2013 template woes
我正在創建一個輕量級的跨平台插件框架,該框架在應用程序和插件之間使用C接口(通常,但並非總是用C ++編寫)。
幫助C ++應用程序和插件編寫者的挑戰之一是找到一種簡單的方法來跨C接口公開C ++對象功能。 我目前的解決方案感覺很簡單,並基於此出色的stackoverflow問題和解答 ,使用模板來“構建”包裝基本C ++成員函數的C簽名函數。
template <typename Tc, typename F, F>
struct MemberFuncWrapper;
template <typename Tc, // C interface structure tag
typename T, // C++ class, derived from Tc
typename R, // C++ member function return type
typename ...Args, // C++ member function argument types
R (T::*f)(Args...) const> // C++ member function
struct MemberFuncWrapper<Tc, R (T::*)(Args...) const, f> {
static R call(const Tc * tc, Args... args) {
const T * t = static_cast<const T *>(tc);
return ((*t).*f)(args...);
}
};
此模板的實例化可在linux(gcc)和mac(clang)下編譯並正常運行,但在Visual Studio 2013中編譯失敗,並顯示以下信息:
error C2440: 'specialization' : cannot convert from 'overloaded-function' to 'void (__cdecl Greeter::* )(void) const'
error C2973: 'MemberFuncWrapper<Tc,R(__cdecl T::* )(Args...) const,f>' : invalid template argument 'overloaded-function'
下面的獨立示例代碼顯示了Visual Studio失敗的行(在Greeter
類定義中)。 我希望有人可以:
下面的獨立代碼演示了在相當冗長的Hello world
應用程序中使用C ++類實現C接口的上下文中使用的模板代碼:
#include <iostream>
#include <utility>
//
// C interface and function(s) typically defined elsewhere
//
#ifdef __cplusplus
extern "C" {
#endif
// The C interface implemented by a 'greeter'
struct greeter_c {
void(*greet_cb)(const struct greeter_c * greeter,
const char * recipient);
};
// Some C function that makes use of a greeter
void broadcast(const struct greeter_c * greeter) {
greeter->greet_cb(greeter, "world");
}
#ifdef __cplusplus
} // extern "C"
#endif
//
// Template magic that envelopes a C++ member
// function call in a C-signature function
//
template <typename Tc, typename F, F>
struct MemberFuncWrapper;
template <typename Tc, // C interface structure tag
typename T, // C++ class, derived from Tc
typename R, // C++ member function return type
typename ...Args, // C++ member function argument types
R (T::*f)(Args...) const> // C++ member function
struct MemberFuncWrapper<Tc, R (T::*)(Args...) const, f> {
static R call(const Tc * tc, Args... args) {
// Cast C structure to C++ object
const T * t = static_cast<const T *>(tc);
// Details such as catching/handling exceptions omitted.
// Call C++ member function
return ((*t).*f)(args...);
}
};
// Repeat of the above for non-const member functions omitted
//
// A C++ class that implements the C 'greeter' interface
//
class Greeter : public greeter_c {
public:
// Constructor
Greeter(const char * greeting) : m_greeting(greeting) {
// Set up C interface callback by wrapping member function
// !! The following line causes the Visual Studio compilation error !!
greet_cb = MemberFuncWrapper<greeter_c,
void (Greeter::*)(const char *) const,
&Greeter::greet>::call;
}
// C++ member function that 'does' the greeting
void greet(const char * recipient) const {
std::cout << m_greeting << " " << recipient << std::endl;
}
private:
const char * m_greeting;
};
// An application that greets using a Greeter's C interface
int main(int argc, char * argv[]) {
// Create C++ object that implements C interface
Greeter a("Hello");
// Greet using Greeter's C interface
broadcast(&a);
return 0;
}
技術細節:
前言: std::forward
在這里沒有用,因為已明確指定了Args...
換句話說,實例化的C接口不再是模板。 std::forward
在非模板代碼中沒有用。 因此,在以下解決方案中未使用std::forward
。
版本1:
template <typename Base, typename Derived, typename R, typename... Args>
struct c_interface_gen {
template <R(Derived::*mem_fn)(Args...)> inline
static R invoke(Base* pb, Args... args) {
return (static_cast<Derived*>(pb)->*mem_fn)(args...);
}
template <R(Derived::*mem_fn)(Args...) const> inline
static R invoke(const Base* pb, Args... args) {
return (static_cast<const Derived*>(pb)->*mem_fn)(args...);
}
};
此版本有效。 但這絕不是優雅。 主要問題在於使用該工具的冗長且不直觀的語法。
版本2:
template <typename Sig>
struct mem_fn_sig;
template <typename R, typename D, typename... Args>
struct mem_fn_sig<R(D::*)(Args...)> {
template <R(D::*mem_fn)(Args...)>
struct mem_fn_inst {
template <typename Base>
struct base {
inline static R invoke(Base* pb, Args... args) {
return (static_cast<D*>(pb)->*mem_fn)(args...);
}
};
};
};
template <typename R, typename D, typename... Args>
struct mem_fn_sig<R(D::*)(Args...) const> {
template <R(D::*mem_fn)(Args...) const>
struct mem_fn_inst {
template <typename Base>
struct base {
inline static R invoke(const Base* pb, Args... args) {
return (static_cast<const D*>(pb)->*mem_fn)(args...);
}
};
};
};
template <typename Sig, Sig inst, typename Base>
struct c_interface_gen:
mem_fn_sig<Sig>:: template mem_fn_inst<inst>:: template base<Base>
{};
顯然,此版本比以前的版本具有更多的代碼。 但是,好處是使用該工具的語法簡單而直觀。 實際上,語法類似於您的原始工具。 我剛剛添加了一些代碼,以使MSVC的編譯過程更加輕松。
通常,您將使用以下功能:
... = c_interface_gen<decltype(&Derived::f), &Derived::f, Base>::invoke;
如果Derived::f
重載,則必須顯式指定其類型,如下所示:
... = c_interface_gen<void(Derived::*)() const, &Derived::f, Base>::invoke;
注意,這里不需要為const
成員函數指定const Base
。 您只需指定基本類型。 模板將自動確定是否應添加const
修飾符。
以下是使用第二個版本的示例代碼:
#include <iostream>
template <typename Sig>
struct mem_fn_sig;
template <typename R, typename D, typename... Args>
struct mem_fn_sig<R(D::*)(Args...)> {
template <R(D::*mem_fn)(Args...)>
struct mem_fn_inst {
template <typename Base>
struct base {
inline static R invoke(Base* pb, Args... args) {
return (static_cast<D*>(pb)->*mem_fn)(args...);
}
};
};
};
template <typename R, typename D, typename... Args>
struct mem_fn_sig<R(D::*)(Args...) const> {
template <R(D::*mem_fn)(Args...) const>
struct mem_fn_inst {
template <typename Base>
struct base {
inline static R invoke(const Base* pb, Args... args) {
return (static_cast<const D*>(pb)->*mem_fn)(args...);
}
};
};
};
template <typename Sig, Sig inst, typename Base>
struct c_interface_gen:
mem_fn_sig<Sig>:: template mem_fn_inst<inst>:: template base<Base>
{};
//
// C interface and function(s) typically defined elsewhere
//
#ifdef __cplusplus
extern "C" {
#endif
// The C interface implemented by a 'greeter'
struct greeter_c {
void(*greet_cb)(const struct greeter_c * greeter,
const char * recipient);
};
// Some C function that makes use of a greeter
void broadcast(const struct greeter_c * greeter) {
greeter->greet_cb(greeter, "world");
}
#ifdef __cplusplus
} // extern "C"
#endif
//
// A C++ class that implements the C 'greeter' interface
//
class Greeter : public greeter_c {
public:
// Constructor
Greeter(const char * greeting) : m_greeting(greeting) {
// Set up C interface callback by wrapping member function
// !! The following line causes the Visual Studio compilation error !!
greet_cb = c_interface_gen<decltype(&Greeter::greet), &Greeter::greet, greeter_c>::invoke;
}
// C++ member function that 'does' the greeting
void greet(const char * recipient) const {
std::cout << m_greeting << " " << recipient << std::endl;
}
private:
const char * m_greeting;
};
// An application that greets using a Greeter's C interface
int main(int argc, char * argv[]) {
// Create C++ object that implements C interface
Greeter a("Hello");
// Greet using Greeter's C interface
broadcast(static_cast<const greeter_c *>(&a));
return 0;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.