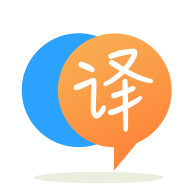
[英]Using fluent API to set up composite foreign key in one-to-one relation in legacy database
[英]How do I set up the relation using Fluent API between an Employee entity and an ICollection<Employee>?
我正在使用實體框架,並且在其他實體中有一個Employee實體。 我希望員工擁有的一個導航屬性是該員工管理的員工列表。 這是Employee類(它實際上使用EF前綴,但是我指的是不帶EF的前綴,以減少混亂):
/// <summary>
/// The Class model for the Employee Entity
/// </summary>
[Table("employee_entity")]
public class EFEmployee : EFBusinessEntity
{
/// <summary>
/// The hire date of the employee
/// </summary>
[Column("hire_date")]
public DateTime HireDate { get; set; }
/// <summary>
/// The list of jobtitles for the employee
/// </summary>
[Column("job_title")]
[Required]
public byte[] JobTitle { get; set; }
/// <summary>
/// The Employee's salary (note: not attached to jobtitle necessarily)
/// </summary>
[Column("salary")]
public int Salary { get; set; }
/// <summary>
/// List of Certifications for the employee
/// </summary>
[Column("certifications")]
public byte[] Certifications { get; set; }
/// <summary>
/// Employee's stored up vacation time
/// </summary>
[Column("vacation_time")]
public int VacationTime { get; set; }
/// <summary>
/// Employee's stored up sick time
/// </summary>
[Column("sick_time")]
public int SickTime { get; set; }
/// <summary>
/// Maps the employee entity to the office entity
/// </summary>
[ForeignKey("office_entity")]
public virtual EFOffice Office { get; set; }
/// <summary>
/// Maps the employee entity to the person entity
/// </summary>
public virtual EFPerson Identity { get; set; }
/// <summary>
/// Maps the employee entity to another employee entity
/// </summary>
public virtual EFEmployee ReportingTo { get; set; }
/// <summary>
/// Maps the employee entity to a collection of employee entites
/// </summary>
public virtual ICollection<EFEmployee> Managing { get; set; }
/// <summary>
/// Constructor for an Entity. Only requires the properties that cannot be null.
/// </summary>
/// <param name="Id"></param>
/// <param name="TenantId"></param>
/// <param name="hire"></param>
/// <param name="titles"></param>
/// <param name="salary"></param>
/// <param name="certifications"></param>
/// <param name="vacationTime"></param>
/// <param name="sickTime"></param>
public EFEmployee(Guid Id, Guid TenantId, DateTime hire, byte[] titles, int salary, byte[] certifications, int vacationTime, int sickTime)
{
this.HireDate = hire;
this.JobTitle = titles;
this.Salary = salary;
this.Certifications = certifications;
this.SickTime = sickTime;
this.VacationTime = vacationTime;
}
}
如您所見,Employee的導航屬性之一是
public virtual ICollection<EFEmployee> Managing { get; set; }
我有一個EmployeeMap類,該類使用Fluent API在Employee實體和其他實體之間建立關系。 現在還不完整,但是看起來是這樣的:
public partial class EmployeeMap : EntityTypeConfiguration<EFEmployee>
{
public EmployeeMap()
{
// Relations
HasRequired(t => t.Office).WithMany(u => u.Employees);
HasMany(t => t.Managing).WithRequired(u => u.Employees);
}
}
與辦公室和雇員的第一個關系是有效的,因為每個辦公室實體都有許多雇員實體。 但是,第二個關系映射出現錯誤。 具體來說,最后的“雇員”給出了錯誤提示
員工不包含“員工”的定義,也沒有擴展方法等。
很明顯為什么我會收到該錯誤。 我尚未在Employee forEmployees中聲明財產,也不知道為什么要這么做。 但是,如何在兩個相同類型的實體之間建立關系? 如果回頭看一下我的Employee實體類,我還將具有導航屬性:
public virtual EFEmployee ReportingTo { get; set; }
在這里,我還試圖在Employee和另一名Employee之間建立關系,但是我不知道該如何工作,因為在實體數據模型中,Employee實體只是表示為具有其屬性的一個表。 有誰知道我在說什么以及如何解決它?
定義關系時,所使用的屬性必須彼此相反。 就Managing
,我認為您正在尋找的是:
HasMany(t => t.Managing).WithRequired(t => t.ReportingTo);
因為我猜你想要:
someEmployee.ReportingTo.Managing.Contains(someEmployee) == true
你希望每個EFEmployee
在someManager.Managing
有ReportingTo == someManager
。
如果您實際上沒有定義逆屬性,那么在模型配置中就不需要一個逆屬性:
// someEmployee can possibly have many other EFEmployees with
// ReportingTo == someEmployee, but no collection property on someEmployee to
// represent that relationship!
HasRequired(t => t.ReportingTo).WithMany();
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.