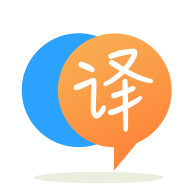
[英]How to merge multiple XML files with data in same format in it to a single XML file with all data together using C#.NET?
[英]How do I merge several different files into a single file with custome file type using c#.net?
我需要將多個文件(視頻,音樂和文本)組合成具有自定義文件類型(例如*.abcd
)和自定義文件數據結構的單個文件,並且該文件只能由我的程序讀取,而我的程序應為能夠分隔此文件的各個部分。 如何在.net
和c#
執行此操作?
https://www.imageupload.co.uk/image/ZWnh
就像M463正確指出的那樣,您可以使用System.IO.Compression
將這些文件壓縮在一起並對其進行加密...盡管加密是一門完全不同的技巧,但又令人頭疼。
更好的選擇是在文件的前幾個字節中包含一些元數據,然后將文件的字節存儲為原始字節。 這樣可以避免任何人僅通過在文本編輯器中查看內容來確定內容。 同樣,如果您想真正保護數據,則不可避免地要進行加密。 但是開始的簡單算法是:
using System;
using System.IO;
using System.Collections.Generic;
namespace Compression
{
public class ClassName
{
public static void Compress(string[] fileNames, string resultantFileName)
{
List<byte> bytesToWrite = new List<byte>();
//add metadata about the number of files
int filesLength = fileNames.Length;
bytesToWrite.AddRange(BitConverter.GetBytes(filesLength));
List<byte[]> files = new List<byte[]>();
foreach(string fileName in fileNames)
{
byte[] bytes = File.ReadAllBytes(fileName);
//add metadata about the size of each file
bytesToWrite.AddRange(BitConverter.GetBytes(bytes.Length));
files.Add(bytes);
}
foreach(byte[] bytes in files)
{
//write the actual files itself
bytesToWrite.AddRange(bytes);
}
File.WriteAllBytes(resultantFileName, bytesToWrite.ToArray());
}
public static void Decompress(string fileName)
{
List<byte> bytes = new List<byte>(File.ReadAllBytes(fileName));
//this int represents the number of files in the byte array
int filesLength = BitConverter.ToInt32(bytes.ToArray(), 0);
List<int> sizes = new List<int>();
//get the size of each file
for(int i = 0; i < filesLength; i++)
{
//the first 2 bytes represent the number of files
//then each succeding int represents the size of each file
int size = BitConverter.ToInt32(bytes.ToArray(), 2 + i * 2);
sizes.Add(size);
}
//now read all the files
for(int i = 0; i < filesLength; i++)
{
int lastByteTillNow = 0;
for(int j = 0; j < i; j++)
lastByteTillNow += sizes[j];
File.WriteAllBytes("file " + i, bytes.GetRange(2 + 2 * filesLength + lastByteTillNow, sizes[i]).ToArray());
}
}
}
}
現在顯然這不是您遇到的最佳算法,也不是最優化的代碼片段。 畢竟,這正是我能在10-15分鍾內得出的結果。 所以我還沒有測試過。 但是,關鍵是,它給您帶來了靈感,不是嗎? 我已經將每個文件的大小限制為Int32
的最大長度(但是,將其更改為Int64
又名long
不會很麻煩)。 但這給你一個主意。 您甚至可以修改代碼段,以通過MemoryStreams(我認為是Systtem.IO.MemorySteam
)在RAM中進行加載和寫入。 但是,無論如何,這應該給您一個開始!
包含文件的加密的.zip容器如何? .NET框架中已經可以處理.zip文件,請查看System.IO.Compression
命名空間。 或者,您可以使用一些第三方庫。
如果您確實要...,甚至可以通過重命名文件來強制使用其他文件擴展名。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.