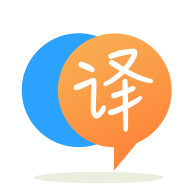
[英]How could I split a string after the n-th field and create an array from the remaining parts in PHP?
[英]How to reduce time to find the n-th place from consecutive digits number for less than 1 second
我正在進行編程測試,並且有這樣的問題
從這個連續的數字:
123456789101112131415161718192021 ....
例如,第10位是1,第11位是0,依此類推
第1,000,000位是什么?
第十億位數是多少?
第1,000,000,000,000位數字是多少?
您的解決方案應在1秒以下運行。
我得到了答案,但仍然超過1秒。 我嘗試使用多項式。
那么,如何減少從上述數字中找到第n位的時間?
這是我的答案,(PHP):
function digit_pol ($n) {
$counter = 1;
$digit_arr = array();
for ($i = 1; $i <= $n; $i++) {
for ($j = 0; $j < strlen($i); $j++) {
// If array more than 100, always shift (limit array length to 11)
if($i > 10) {
array_shift($digit_arr);
}
// Fill array
$digit_arr[$counter] = substr($i, $j, 1);
// Found
if($counter == $n) {
return $digit_arr[$counter];
}
$counter++;
}
}
}
/**
* TESTING
*/
$find_place = 1000000;
$result = digit_pol($find_place);
echo "Digit of " . $find_place . "th is <b>" . $result . "</b>";
重要的是要意識到,采取重要步驟很容易:
1 digit numbers: 123456789 - 9 * 1 digit
2 digit numbers: 101112...9899 - 90 * 2 digits
3 digit numbers: 100101102...998999 - 900 * 3 digits
4 digit numbers: ...
現在,您可以執行一次遞歸解決方案,一次跳過9×10 k × k個數字,直到達到n
處於當前幅度的數字范圍內的基本情況。
當您知道要查找的特定范圍時,很容易找到第n
個數字。 首先將n
除以每個數字的長度。 這將數字偏移量轉換為數字偏移量 。 現在,向其添加10 k以獲取實際的數字。此時,只需在給定數字中查找特定數字即可。
以n = 1234
為例:
n > 9
,所以它不在單位范圍內:
n -= 9
),然后繼續輸入兩位數字... n > 90 * 2
所以也不在兩位數范圍內
n -= 90*2
)並繼續輸入3位數字... n < 900 * 3
所以我們要在100101102...
序列中尋找一個數字 100 + n / 3
來查找要查找的特定數字。 在這種情況下等於448
。 n % 3
(在這種情況下等於1
)來查找要選擇的數字。 因此,最終結果為4
。 這是Java中的解決方案:
public static char nthDigit(int n, int digitsInFirstNum) {
int magnitude = (int) Math.pow(10, digitsInFirstNum - 1);
int digitsInMagnitude = 9 * magnitude * digitsInFirstNum;
if (n < digitsInMagnitude) {
int number = magnitude + n / digitsInFirstNum;
int digitPos = n % digitsInFirstNum;
return String.valueOf(number).charAt(digitPos);
}
return nthDigit(n - digitsInMagnitude, digitsInFirstNum + 1);
}
public static char nthDigit(int n) {
return nthDigit(n, 1);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.