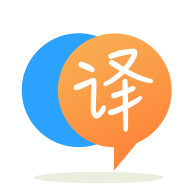
[英]Error when trying to send transactional email from template using sendGrid
[英]Created sendgrid email template, how to call it from Java
我在 sendgrid 中創建了 email 模板 - 具有可替換的值;
我從 rabbitMQ 隊列中獲取 JSON 有效載荷(包含替代值)以處理 email。 我的問題是如何從 Java 調用 sendgrid email 模板?
我找到了解決方案,通過sendgrid調用sendgrid模板和email的方法如下:
SendGrid sendGrid = new SendGrid("username","password"));
SendGrid.Email email = new SendGrid.Email();
//Fill the required fields of email
email.setTo(new String[] { "xyz_to@gmail.com"});
email.setFrom("xyz_from@gmail.com");
email.setSubject(" ");
email.setText(" ");
email.setHtml(" ");
// Substitute template ID
email.addFilter(
"templates",
"template_id",
"1231_1212_2323_3232");
//place holders in template, dynamically fill values in template
email.addSubstitution(
":firstName",
new String[] { firstName });
email.addSubstitution(
":lastName",
new String[] { lastName });
// send your email
Response response = sendGrid.send(email);
這是我的工作解決方案。
import com.fasterxml.jackson.annotation.JsonProperty;
import com.sendgrid.*;
import java.io.IOException;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
public class MailUtil {
public static void main(String[] args) throws IOException {
Email from = new Email();
from.setEmail("fromEmail");
from.setName("Samay");
String subject = "Sending with SendGrid is Fun";
Email to = new Email();
to.setName("Sam");
to.setEmail("ToEmail");
DynamicTemplatePersonalization personalization = new DynamicTemplatePersonalization();
personalization.addTo(to);
Mail mail = new Mail();
mail.setFrom(from);
personalization.addDynamicTemplateData("name", "Sam");
mail.addPersonalization(personalization);
mail.setTemplateId("TEMPLATE-ID");
SendGrid sg = new SendGrid("API-KEY");
Request request = new Request();
try {
request.setMethod(Method.POST);
request.setEndpoint("mail/send");
request.setBody(mail.build());
Response response = sg.api(request);
System.out.println(response.getStatusCode());
System.out.println(response.getBody());
System.out.println(response.getHeaders());
} catch (IOException ex) {
throw ex;
}
}
private static class DynamicTemplatePersonalization extends Personalization {
@JsonProperty(value = "dynamic_template_data")
private Map<String, String> dynamic_template_data;
@JsonProperty("dynamic_template_data")
public Map<String, String> getDynamicTemplateData() {
if (dynamic_template_data == null) {
return Collections.<String, String>emptyMap();
}
return dynamic_template_data;
}
public void addDynamicTemplateData(String key, String value) {
if (dynamic_template_data == null) {
dynamic_template_data = new HashMap<String, String>();
dynamic_template_data.put(key, value);
} else {
dynamic_template_data.put(key, value);
}
}
}
}
這是來自最新 API 規范的示例:
import com.sendgrid.*;
import java.io.IOException;
public class Example {
public static void main(String[] args) throws IOException {
Email from = new Email("test@example.com");
String subject = "I'm replacing the subject tag";
Email to = new Email("test@example.com");
Content content = new Content("text/html", "I'm replacing the <strong>body tag</strong>");
Mail mail = new Mail(from, subject, to, content);
mail.personalization.get(0).addSubstitution("-name-", "Example User");
mail.personalization.get(0).addSubstitution("-city-", "Denver");
mail.setTemplateId("13b8f94f-bcae-4ec6-b752-70d6cb59f932");
SendGrid sg = new SendGrid(System.getenv("SENDGRID_API_KEY"));
Request request = new Request();
try {
request.setMethod(Method.POST);
request.setEndpoint("mail/send");
request.setBody(mail.build());
Response response = sg.api(request);
System.out.println(response.getStatusCode());
System.out.println(response.getBody());
System.out.println(response.getHeaders());
} catch (IOException ex) {
throw ex;
}
}
}
https://github.com/sendgrid/sendgrid-java/blob/master/USE_CASES.md
最近 Sendgrid 升級了 Maven 版本 v4.3.0 這樣您就不必為動態數據內容創建額外的類。 從此鏈接閱讀更多信息https://github.com/sendgrid/sendgrid-java/pull/449
如果您正在執行spring-boot
maven 項目,則需要在 pom.xml 中添加sendgrid-java
maven 依賴pom.xml
。 同樣,在項目resource
文件夾下的application.properties
文件中,分別在spring.sendgrid.api-key=SG.xyz
和templateId=d-cabc
等屬性下添加SENDGRID API KEY
AND TEMPLATE ID
。
完成了預先設置。 您可以創建一個簡單的 controller class,如下所示:
快樂編碼!
@RestController
@RequestMapping("/sendgrid")
public class MailResource {
private final SendGrid sendGrid;
@Value("${templateId}")
private String EMAIL_TEMPLATE_ID;
public MailResource(SendGrid sendGrid) {
this.sendGrid = sendGrid;
}
@GetMapping("/test")
public String sendEmailWithSendGrid(@RequestParam("msg") String message) {
Email from = new Email("bijay.shrestha@f1soft.com");
String subject = "Welcome Fonesal Unit to SendGrid";
Email to = new Email("birat.bohora@f1soft.com");
Content content = new Content("text/html", message);
Mail mail = new Mail(from, subject, to, content);
mail.setReplyTo(new Email("bijay.shrestha@f1soft.com"));
mail.setTemplateId(EMAIL_TEMPLATE_ID);
Request request = new Request();
Response response = null;
try {
request.setMethod(Method.POST);
request.setEndpoint("mail/send");
request.setBody(mail.build());
response = sendGrid.api(request);
System.out.println(response.getStatusCode());
System.out.println(response.getBody());
System.out.println(response.getHeaders());
} catch (IOException ex) {
System.out.println(ex.getMessage());
}
return "Email was successfully sent";
}
}
這是基於com.sendgrid:sendgrid-java:4.8.3
Email from = new Email(fromEmail);
Email to = new Email(toEmail);
String subject = "subject";
Content content = new Content(TEXT_HTML, "dummy value");
Mail mail = new Mail(from, subject, to, content);
// Using template to send Email, so subject and content will be ignored
mail.setTemplateId(request.getTemplateId());
SendGrid sendGrid = new SendGrid(SEND_GRID_API_KEY);
Request sendRequest = new Request();
sendRequest.setMethod(Method.POST);
sendRequest.setEndpoint("mail/send");
sendRequest.setBody(mail.build());
Response response = sendGrid.api(sendRequest);
您還可以在這里找到完整的示例: 完整的 Email object Java 示例
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.