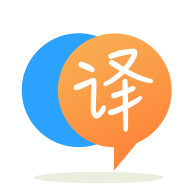
[英]How do I send a SIGTERM or SIGINT signal to the server in the boost HTML3 example?
[英]how to send SIGINT signal multiple times
我試圖從其他線程向主線程發送SIGINT信號。 主線程已為信號分配了一個處理程序。 當我發送第一個信號時,它被處理程序捕獲。 但是我想連續發送信號。 但是在處理完第一個信號后,程序終止。 我放了一會兒循環。 因此,我期望它應該繼續發送這些信號。 以下是我的代碼
#include <signal.h>
#include <stdio.h>
#include <stdlib.h>
#include <windows.h>
static void catch_function(int signo) {
puts("Interactive attention signal caught.");
}
DWORD WINAPI MyThreadFunction( LPVOID lpParam )
{
while(1)
{
Sleep(50);
puts("Raising the interactive attention signal.");
if (raise(SIGINT) != 0)
{
fputs("Error raising the signal.\n", stderr);
return EXIT_FAILURE;
}
}
return 0;
}
int main(void)
{
if (signal(SIGINT, catch_function) == SIG_ERR) {
fputs("An error occurred while setting a signal handler.\n", stderr);
return EXIT_FAILURE;
}
HANDLE thread;
DWORD threadId;
thread = CreateThread(NULL, 0, &MyThreadFunction, NULL, 0, &threadId);
if(!thread)
{
printf("CreateThread() failed");
}
while(1)
{
Sleep(50);
}
puts("Exiting.");
return 0;
}
以下代碼的輸出是
Raising the interactive attention signal.
Interactive attention signal caught.
Raising the interactive attention signal.
我也嘗試過使用一個簡單的例子。 在這里,我發送了3次信號,但只有第一次捕獲到信號。 之后,程序終止。 以下是代碼
#include <signal.h>
#include <stdio.h>
void signal_handler(int signal)
{
printf("Received signal %d\n", signal);
}
int main(void)
{
// Install a signal handler.
signal(SIGINT, signal_handler);
printf("Sending signal %d\n", SIGINT);
raise(SIGINT);
raise(SIGINT);
raise(SIGINT);
printf("Exit main()\n");
}
輸出是
sending signal 2
Received signal 2
所以我想知道如何繼續從一個線程向另一個線程發送一些信號? 我希望我的一個線程可以發送SIGINT信號,而main可以捕獲它們並相應地執行一些操作。
通常,在第一個信號之后,信號處理程序將重置為SIG_DFL
(Unix V信號),並且SIG_INT
的默認行為是退出程序。 這就是您觀察到的。 因此,將需要再次重新安裝處理程序。 再次在處理程序中安裝它:
void signal_handler(int signal)
{
signal(SIGINT, signal_handler);
printf("Received signal %d\n", signal);
}
更好的方法是改用sigaction
因為它沒有這種行為。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.