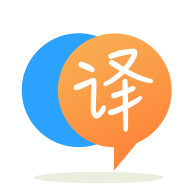
[英]How do I make a class that can create an instance of another class and access private members of the owner
[英]How do I make a method private and access it from another class?
我很好奇如何使addOperands方法私有,而不是像現在這樣編碼的公共方法。 我已經閱讀了一些get和set訪問器的示例,但我仍然不理解這個概念。 我該如何將addOperands方法設置為CalcEngine類的私有方法,並且仍然能夠從另一個類使用此方法?
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Project3_WindowsCalculator
{
class CalcEngine
{
private int operationResult;
public int addOperands(int operand1, int operand2)
{
operationResult = operand1 + operand2;
return operationResult;
}
public int subtractOperands(int operand1, int operand2)
{
operationResult = operand1 - operand2;
return operationResult;
}
public int multiplyOperands(int operand1, int operand2)
{
operationResult = operand1 * operand2;
return operationResult;
}
public int divideOperands(int operand1, int operand2)
{
operationResult = operand1 / operand2;
return operationResult;
}
}
}
這是使用它的類:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace Project3_WindowsCalculator
{
public partial class MainWindow : Window
{
CalcEngine c = new CalcEngine();
bool addSelected;
bool subtractSelected;
bool multiplySelected;
bool divideSelected;
bool operationSelected;
int operationResult;
int operand1;
int operand2;
public MainWindow()
{
InitializeComponent();
}
private void C_Click(object sender, RoutedEventArgs e)
{
txtDisplay.Text = "0";
operationResult = 0;
addSelected = false;
subtractSelected = false;
multiplySelected = false;
divideSelected = false;
operationSelected = false;
operand1 = 0;
operand2 = 0;
}
private void Multiply_Click(object sender, RoutedEventArgs e)
{
if (operand1 == 0 && operand2 == 0 && operationSelected == false) //operationSelected makes sure * cannot be pressed more than once at a time
{ //if + is clicked and nothing has been stored into operand1 yet
Int32.TryParse(txtDisplay.Text, out operand1); //store the displayed text into the operand1 variable
}
else if (operand1 != 0 && operand2 == 0 && operationSelected == false)
{ //if + is clicked and both operand variables are stored with something
Int32.TryParse(txtDisplay.Text, out operand2); //store what's on the display into the operand 2 variable
if (addSelected)
{
operand1 = c.addOperands(operand1, operand2);
addSelected = false;
}
else if (subtractSelected)
{
operand1 = c.subtractOperands(operand1, operand2);
subtractSelected = false;
}
else if (multiplySelected)
{
operand1 = c.multiplyOperands(operand1, operand2);
multiplySelected = false;
}
else if (divideSelected)
{
operand1 = c.divideOperands(operand1, operand2);
divideSelected = false;
}
txtDisplay.Text = operand1.ToString();
operand2 = 0; //empty the operand2 variable
}
operationSelected = true; multiplySelected = true;
}
private void Divide_Click(object sender, RoutedEventArgs e)
{
if (operand1 == 0 && operand2 == 0 && operationSelected == false)
{ //if + is clicked and nothing has been stored into operand1 yet
Int32.TryParse(txtDisplay.Text, out operand1); //store the displayed text into the operand1 variable
}
else if (operand1 != 0 && operand2 == 0 && operationSelected == false)
{ //if + is clicked and both operand variables are stored with something
Int32.TryParse(txtDisplay.Text, out operand2); //store what's on the display into the operand 2 variable
if (addSelected)
{
operand1 = c.addOperands(operand1, operand2);
addSelected = false;
}
else if (subtractSelected)
{
operand1 = c.subtractOperands(operand1, operand2);
subtractSelected = false;
}
else if (multiplySelected)
{
operand1 = c.multiplyOperands(operand1, operand2);
multiplySelected = false;
}
else if (divideSelected)
{
operand1 = c.divideOperands(operand1, operand2);
divideSelected = false;
}
txtDisplay.Text = operand1.ToString();
operand2 = 0; //empty the operand2 variable
}
operationSelected = true; divideSelected = true;
}
private void Subtract_Click(object sender, RoutedEventArgs e)
{
if (operand1 == 0 && operand2 == 0 && operationSelected == false)
{ //if + is clicked and nothing has been stored into operand1 yet
Int32.TryParse(txtDisplay.Text, out operand1); //store the displayed text into the operand1 variable
}
else if (operand1 != 0 && operand2 == 0 && operationSelected == false)
{ //if + is clicked and both operand variables are stored with something
Int32.TryParse(txtDisplay.Text, out operand2); //store what's on the display into the operand 2 variable
if (addSelected)
{
operand1 = c.addOperands(operand1, operand2);
addSelected = false;
}
else if (subtractSelected)
{
operand1 = c.subtractOperands(operand1, operand2);
subtractSelected = false;
}
else if (multiplySelected)
{
operand1 = c.multiplyOperands(operand1, operand2);
multiplySelected = false;
}
else if (divideSelected)
{
operand1 = c.divideOperands(operand1, operand2);
divideSelected = false;
}
txtDisplay.Text = operand1.ToString();
operand2 = 0; //empty the operand2 variable
}
operationSelected = true; subtractSelected = true;
}
private void Add_Click(object sender, RoutedEventArgs e)
{
if (operand1 == 0 && operand2 == 0 && operationSelected == false){ //if + is clicked and nothing has been stored into operand1 yet
Int32.TryParse(txtDisplay.Text, out operand1); //store the displayed text into the operand1 variable
}
else if (operand1 != 0 && operand2 == 0 && operationSelected == false)
{ //if + is clicked and both operand variables are stored with something
Int32.TryParse(txtDisplay.Text, out operand2); //store what's on the display into the operand 2 variable
if (addSelected)
{
operand1 = c.addOperands(operand1, operand2);
addSelected = false;
}
else if (subtractSelected)
{
operand1 = c.subtractOperands(operand1, operand2);
subtractSelected = false;
}
else if (multiplySelected)
{
operand1 = c.multiplyOperands(operand1, operand2);
multiplySelected = false;
}
else if (divideSelected)
{
operand1 = c.divideOperands(operand1, operand2);
divideSelected = false;
}
txtDisplay.Text = operand1.ToString();
operand2 = 0; //empty the operand2 variable
}
operationSelected = true; addSelected = true;
}
private void Equals_Click(object sender, RoutedEventArgs e)
{
Int32.TryParse(txtDisplay.Text, out operand2);
if(addSelected){
operationResult = c.addOperands(operand1, operand2);
//operand1 = operationResult;
}
if(subtractSelected){
operationResult = c.subtractOperands(operand1, operand2);
}
if(multiplySelected){
operationResult = c.multiplyOperands(operand1, operand2);
}
if(divideSelected){
operationResult = c.divideOperands(operand1, operand2);
}
txtDisplay.Text = operationResult.ToString();
Int32.TryParse(txtDisplay.Text, out operand1);
addSelected = false;
subtractSelected = false;
multiplySelected = false;
divideSelected = false;
operationSelected = false;
}
private void Seven_Click(object sender, RoutedEventArgs e)
{
txtDisplay.Text = txtDisplay.Text == "0" || operationSelected ? "7" : txtDisplay.Text + "7";
operationSelected = false;
}
private void Eight_Click(object sender, RoutedEventArgs e)
{
txtDisplay.Text = txtDisplay.Text == "0" || operationSelected ? "8" : txtDisplay.Text + "8";
operationSelected = false;
}
private void Nine_Click(object sender, RoutedEventArgs e)
{
txtDisplay.Text = txtDisplay.Text == "0" || operationSelected ? "9" : txtDisplay.Text + "9";
operationSelected = false;
}
private void Four_Click(object sender, RoutedEventArgs e)
{
txtDisplay.Text = txtDisplay.Text == "0" || operationSelected ? "4" : txtDisplay.Text + "4";
operationSelected = false;
}
private void Five_Click(object sender, RoutedEventArgs e)
{
txtDisplay.Text = txtDisplay.Text == "0" || operationSelected ? "5" : txtDisplay.Text + "5";
operationSelected = false;
}
private void Six_Click(object sender, RoutedEventArgs e)
{
txtDisplay.Text = txtDisplay.Text == "0" || operationSelected ? "6" : txtDisplay.Text + "6";
operationSelected = false;
}
private void One_Click(object sender, RoutedEventArgs e)
{
txtDisplay.Text = txtDisplay.Text == "0" || operationSelected ? "1" : txtDisplay.Text + "1";
operationSelected = false;
}
private void Two_Click(object sender, RoutedEventArgs e)
{
txtDisplay.Text = txtDisplay.Text == "0" || operationSelected ? "2" : txtDisplay.Text + "2";
operationSelected = false;
}
private void Three_Click(object sender, RoutedEventArgs e)
{
txtDisplay.Text = txtDisplay.Text == "0" || operationSelected ? "3" : txtDisplay.Text + "3";
operationSelected = false;
}
private void btn_0_Click(object sender, RoutedEventArgs e){}
private void Off_Click(object sender, RoutedEventArgs e)
{
C_Click(sender, e);
txtDisplay.Foreground = new SolidColorBrush(Colors.White);
}
private void On__Click(object sender, RoutedEventArgs e){}
private void On_Click(object sender, RoutedEventArgs e)
{
C_Click(sender, e);
txtDisplay.Foreground = new SolidColorBrush(Colors.Black);
}
}
}
看看有關訪問修飾符的 MSDN文章 。 如果要保護您的類不受外部程序集的影響,只需將其設置為internal
但是如果您想要不同的內容,我有幾點建議。 (我不確定您需要什么,所以我現在發布了所有建議)
方法1:僅限Getter屬性
public class MyClass
{
// Read-only access from outside, no 'set'
public object RestrictedMember { get; private set; }
}
方法2:受保護的修飾符
使用此修飾符,僅派生類可以訪問該成員。
public class BaseClass
{
protected object RestrictedMember;
}
public DerivedClass : BaseClass
{
public DerivedClass() : base()
{
// You can access base class's protected member
RestrictedMember = new object();
}
}
方法3:代表
對於方法,您可以聲明自己的委托。
public delegate string MyDelegate(int a, int b);
public class MyClass
{
// Also you should make it readonly or getter-only property if you don't want outside changes
public MyDelegate restrictedMethod = _restrictedMethod;
private string _restrictedMethod(int a, int b)
{
return (a + b).ToString();
}
}
public class Test
{
public void It(MyClass mc)
{
// You can call method and get result
string sumAsStr = mc.restrictedMethod(3, 5);
}
}
我沒有測試他們。 可以修復一些小錯誤/語法錯誤。
如果兩個類都在同一程序集中,則可以將函數設為內部函數,而不是私有函數。 內部的任何內容都可以在同一程序集中訪問,但是該程序之外的任何類都不可見。
您可能會看到的getter和setter訪問器可能是在使類的屬性在類外部不可變。
考慮一個類Foo
公共類Foo {公共字符串Bar {get; 私人套裝; }
..}
在這種情況下,可以在Foo外部訪問Bar屬性,但是不能在Foo外部對其進行修改。
我剛剛編寫了一個可能會幫助另一個問題的答案:
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.