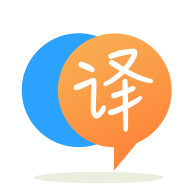
[英]Create a new object from user input using array and not ArrayList in Java
[英]how to create object using user input using array and not array list
當我編譯執行時,我得到java.lang.ArrayOutOfBoundsException
。 我如何解決它? 必須使用array而不是arrayList。 這是BlueJ
。 我正在從台式機和筆記本電腦繼承方法,而這兩個類正在獲取計算機中父類的值。
import java.util.Scanner;
public class testComputer {
public static void main(String args[]) {
String a;
Scanner scanner = new Scanner(System.in);
Desktop[] desk = new Desktop[5];
Laptop[] lap = new Laptop[5];
int Desk = 0;
int Lap = 0;
do {
System.out.println("*************** Artificial Intelligence Co.***************");
System.out.println("Computer Menu");
System.out.println("1. Add a new Desktop Information");
System.out.println("2. Add a new Laptop Information");
System.out.println("3. Display all Computer Information");
System.out.println("4. Quit");
System.out.println("***********************************************************");
System.out.print("Please enter either 1 to 4: ");
a = scanner.nextLine();
if (a.equals("1")) {
desk[Desk] = new Desktop();
desk[Desk].setDisplayDesktopInfo();
} else if (a.equals("2")) {
lap[Lap] = new Laptop();
lap[Lap].setDisplayLaptopInfo();
} else if (a.equals("3")) {
int i = 0;
for (i = 0; i <= desk.length; i++) {
if (desk.length == i) {
System.out.println("================Desktop================");
desk[i] = new Desktop();
desk[i].getDisplayDeskInfo();
System.out.println("");
} else {
System.out.println("Error!");
}
}
int j = 0;
for (j = 0; j <= lap.length; j++) {
if (lap.length == j) {
System.out.println("================Laptop================");
lap[j] = new Laptop();
lap[j].getDisplayLapInfo();
System.out.println("");
} else {
System.out.println("Error");
}
}
} else if (a.equals("4")) {
System.out.println("Good Bye!");
}
} while (!a.equals("4"));
}
}
import java.util.Scanner;
public class Desktop extends Computer
{
private static final String alphanumeric_regex_pattern = "^[a-zA-Z0-9]*$";
private String Monitor;
public Desktop()
{
}
void setMonitor(String monitor)
{
Monitor = monitor;
}
String getMonitor()
{
return Monitor;
}
void setDisplayDesktopInfo()
{
Scanner scanner = new Scanner(System.in);
System.out.println("========================================");
System.out.println("Information for new Desktop");
System.out.println("========================================");
System.out.print("What is the Computer ID: " + "");
setComputerID(scanner.nextLine());
System.out.print("What is the Processor Speed: " + "");
setprocessorSpeed(scanner.nextLine());
System.out.print("What is the RAM: " + "");
setRAM(scanner.nextLine());
System.out.print("What is the Harddisk size: " + "");
setHarddisk(scanner.nextLine());
System.out.print("What is the Monitor Type: " + "");
setMonitor(scanner.nextLine());
System.out.println("");
System.out.println("Your information has been added successfully.");
System.out.print("");
}
void getDisplayDeskInfo()
{
System.out.println("Computer ID: " + getComputerID());
System.out.println("Processor Speed: " + getprocessorSpeed());
System.out.println("RAM: " + getRAM());
System.out.println("Harddisk: " + getHarddisk());
System.out.println("Monitor: " + getMonitor());
System.out.print("");
}
}
import java.util.Scanner;
public class Laptop extends Computer
{
private String Weight;
void setWeight(String weight)
{
Weight = weight;
}
String getWeight()
{
return Weight;
}
void setDisplayLaptopInfo()
{
Scanner scanner = new Scanner(System.in);
System.out.println("========================================");
System.out.println("Information for new Laptop");
System.out.println("========================================");
System.out.print("What is the Computer ID: " + "");
setComputerID(scanner.nextLine());
System.out.print("What is the Processor Speed: " + "");
setprocessorSpeed(scanner.nextLine());
System.out.print("What is the RAM: " + "");
setRAM(scanner.nextLine());
System.out.print("What is the Harddisk size: " + "");
setHarddisk(scanner.nextLine());
System.out.print("What is the Weight: " + "");
setWeight(scanner.nextLine());
System.out.println("");
System.out.println("Your information has been added successfully.");
System.out.print("");
}
void getDisplayLapInfo()
{
System.out.println("Computer ID: " + getComputerID());
System.out.println("Processor Speed: " + getprocessorSpeed());
System.out.println("RAM: " + getRAM());
System.out.println("Harddisk: " + getHarddisk());
System.out.println("Weight: " + getWeight());
System.out.print("");
}
}
*************** Artificial Intelligence Co.***************
Computer Menu
1. Add a new Desktop Information
2. Add a new Laptop Information
3. Display all Computer Information
4. Quit
***********************************************************
Please enter either 1 to 4: 1
========================================
Information for new Desktop
========================================
What is the Computer ID: D001
What is the Processor Speed: 3.3GHZ
What is the RAM: 4GB
What is the Harddisk size: 80GB
What is the Monitor Type: CRT
Your information has been added successfully.
*************** Artificial Intelligence Co.***************
Computer Menu
1. Add a new Desktop Information
2. Add a new Laptop Information
3. Display all Computer Information
4. Quit
***********************************************************
Please enter either 1 to 4: 3
If you see this message means you have not done yet!
If you see this message means you have not done yet!
If you see this message means you have not done yet!
If you see this message means you have not done yet!
If you see this message means you have not done yet!
Error
Error
Error
Error
Error
*************** Artificial Intelligence Co.***************
Computer Menu
1. Add a new Desktop Information
2. Add a new Laptop Information
3. Display all Computer Information
4. Quit
***********************************************************
Please enter either 1 to 4: 4
Good Bye!
如果用戶選擇3,則在顯示信息之前您正在創建一台新的筆記本電腦對象,我刪除了這些行,並且您的代碼將頑固地生成ArrayIndexOutOfBoundsException ,現在此代碼是無錯誤的,其余的一切由您決定,享受。
import java.util.Scanner;
public class testComputer {
public static void main(String args[]) {
String a;
Scanner scanner = new Scanner(System.in);
Desktop[] desk = new Desktop[5];
Laptop[] lap = new Laptop[5];
int Desk = 0;
int Lap = 0;
do {
System.out.println("*************** Artificial Intelligence Co.***************");
System.out.println("Computer Menu");
System.out.println("1. Add a new Desktop Information");
System.out.println("2. Add a new Laptop Information");
System.out.println("3. Display all Computer Information");
System.out.println("4. Quit");
System.out.println("***********************************************************");
System.out.print("Please enter either 1 to 4: ");
a = scanner.nextLine();
if (a.equals("1")) {
desk[Desk] = new Desktop();
desk[Desk].setDisplayDesktopInfo();
++Desk;
} else if (a.equals("2")) {
lap[Lap] = new Laptop();
lap[Lap].setDisplayLaptopInfo();
++Lap;
} else if (a.equals("3")) {
int i = 0;
for (i = 0; i < desk.length; i++) {
System.out.println("================Desktop================");
desk[i].getDisplayDeskInfo();
System.out.println("");
}
int j = 0;
for (j = 0; j < lap.length; j++) {
System.out.println("================Laptop================");
lap[j].getDisplayLapInfo();
System.out.println("");
}
} else if (a.equals("4")) {
System.out.println("Good Bye!");
}
} while (!a.equals("4"));
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.