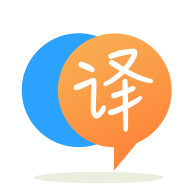
[英]How to parse list of JSON objects surrounded by [] using Retrofit and GSON?
[英]How can I parse JSON Array Using Retrofit and GSON?
我已經學習了如何通過Retrofit Gson解析JSON對象,但是我需要通過Retrofit Gson解析完整的JSON數組。
我需要解析以下內容:
"{\n" +
" \"snappedPoints\": [\n" +
" {\n" +
" \"location\": {\n" +
" \"latitude\": -35.2784167,\n" +
" \"longitude\": 149.1294692\n" +
" },\n" +
" \"originalIndex\": 0,\n" +
" \"placeId\": \"ChIJoR7CemhNFmsRQB9QbW7qABM\"\n" +
" },\n" +
" {\n" +
" \"location\": {\n" +
" \"latitude\": -35.280321693840129,\n" +
" \"longitude\": 149.12908274880189\n" +
" },\n" +
" \"originalIndex\": 1,\n" +
" \"placeId\": \"ChIJiy6YT2hNFmsRkHZAbW7qABM\"\n" +
" },\n" +
" {\n" +
" \"location\": {\n" +
" \"latitude\": -35.280960897210818,\n" +
" \"longitude\": 149.1293250692261\n" +
" },\n" +
" \"originalIndex\": 2,\n" +
" \"placeId\": \"ChIJW9R7smlNFmsRMH1AbW7qABM\"\n" +
" },\n" +
" {\n" +
" \"location\": {\n" +
" \"latitude\": -35.28142839817933,\n" +
" \"longitude\": 149.1298619971291\n" +
" },\n" +
" \"originalIndex\": 3,\n" +
" \"placeId\": \"ChIJy8c0r2lNFmsRQEZUbW7qABM\"\n" +
" },\n" +
" {\n" +
" \"location\": {\n" +
" \"latitude\": -35.28193988170618,\n" +
" \"longitude\": 149.13001013387623\n" +
" },\n" +
" \"originalIndex\": 4,\n" +
" \"placeId\": \"ChIJ58xCoGlNFmsRUEZUbW7qABM\"\n" +
" },\n" +
" {\n" +
" \"location\": {\n" +
" \"latitude\": -35.282819705480151,\n" +
" \"longitude\": 149.1295597114644\n" +
" },\n" +
" \"originalIndex\": 5,\n" +
" \"placeId\": \"ChIJabjuhGlNFmsREIxAbW7qABM\"\n" +
" },\n" +
" {\n" +
" \"location\": {\n" +
" \"latitude\": -35.283139388422363,\n" +
" \"longitude\": 149.12895618087012\n" +
" },\n" +
" \"originalIndex\": 6,\n" +
" \"placeId\": \"ChIJ1Wi6I2pNFmsRQL9GbW7qABM\"\n" +
" },\n" +
" {\n" +
" \"location\": {\n" +
" \"latitude\": -35.284728724835304,\n" +
" \"longitude\": 149.12835061713685\n" +
" },\n" +
" \"originalIndex\": 7,\n" +
" \"placeId\": \"ChIJW5JAZmpNFmsRegG0-Jc80sM\"\n" +
" }\n" +
" ]\n" +
"}"
我只需要緯度和經度。
這是我知道的通過翻新GSON解析JSON對象的方法
package com.example.akshay.retrofitgson;
import com.google.gson.annotations.Expose;
import com.google.gson.annotations.SerializedName;
/**
* Created by Akshay on 9/6/2015.
*/
public class gitmodel {
@SerializedName("latitude")
@Expose
public String latitude;
@SerializedName("longitude")
@Expose
public String longitude;
public void setLatitude(String latitude)
{
this.latitude = latitude;
}
public String getLatitude()
{
return latitude;
}
public void setLongitude(String longitude)
{
this.longitude = longitude;
}
public String getlatitude()
{
return latitude;
}
}
Retrofit使用Gson庫來序列化Json響應。 只要正確設置了模型類,就可以告訴Gson嘗試將json序列化為該模型類的實例。 您的代碼應類似於以下內容:
String json; // retrieved from file/server
MyObject myObject = new Gson().fromJson(json, MyObject.class)
您可能只需要緯度/經度,但是最簡單的方法是設置POJO以獲取所有內容,然后從POJO中提取緯度/經度。 如果需要,您甚至可以設計反序列化的對象以隱藏內部對象。 對於您的JSON,這非常簡單,只需執行以下操作:
public static class SnappedPoints {
private List<Point> snappedPoints;
public String toString() {
return snappedPoints.toString();
}
}
public static class Point {
private Location location;
public double getLatitude() {
return location.getLatitude();
}
public double getLongitude() {
return location.getLongitude();
}
public String toString() {
return "{" + location.getLatitude() + "," + location.getLongitude() + "}";
}
}
public static class Location {
double latitude;
double longitude;
public double getLatitude() {
return latitude;
}
public double getLongitude() {
return longitude;
}
}
您只需執行以下操作即可看到實際效果:
public static void main(String[] args) {
System.out.println(new Gson().fromJson(json, SnappedPoints.class));
}
或者,在翻新的情況下,如下所示:
public interface MyRetrofitAPI {
@GET("/path/to/json")
void getSnappedPoints(/* arguments */, Callback<SnappedPoints> callback);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.