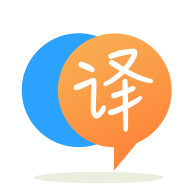
[英]When i try to run my program, the GUI won't load and I can't figure out why
[英]Can't figure out why the program will not run properly
我一直在嘗試使該程序正常運行,該程序應該繼續接受用戶的輸入,直到輸入-1並計算總和。 問題如下:設計並實現一個Java程序(將其命名為InputSum),該程序提示用戶輸入一個正整數。 程序應接受整數,直到用戶輸入值-1(負1)。 用戶輸入-1后,程序將顯示輸入的數字及其總和,如下所示。 請注意,-1不是輸出的一部分。 在繼續處理每個輸入的數字之前,請確保程序已對其進行驗證,因為用戶可能會輸入除負數-1之外的其他負數。
設計您的程序,使其允許用戶在如上所述的同一運行中使用一組不同的輸入重新運行該程序。 記錄您的代碼,並組織和排列輸出,如上所示。
這是我的代碼:
/* Class: CS1301
* Section: 9:30
* Term: Fall 2015
* Name: Matthew Woolridge
* Instructor: Mr. Robert Thorsen
* Assignment: Assignment 6
* Program: 1
* ProgramName: InputSum
* Purpose: The program prompts the user to input numbers until -1 is entered and calculates the sum
* Operation: The information is statically instantiated in the code and
* the data is output to the screen.
* Input(s): The input is the numbers
* Output(s): The output will be the sum of the numbers
* Methodology: The program will use loops to determine if numbers or still to be entered or if -1 was entered
*
*/
import java.util.*;
import java.io.*;
public class InputSum
{
public static void main (String[] args)
{
/******************************************************************************
* Declarations Section *
******************************************************************************/
/****************************CONSTANTS********************************/
Scanner scan = new Scanner(System.in); //Initializes scanner
int n = 1;
int [] num = new int[n]; //Creates array for input numbers
int i;
int sum=0;
/******************************************************************************
* Inputs Section *
******************************************************************************/
System.out.print("Please input integers, note that -1 ends the submissions: "); //Prompts the user for input
/****************************variables********************************/
//***************************Calculations in processing************************//
/******************************************************************************
* Processing Section *
******************************************************************************/
for(i=0; i<num.length; i++)
{
num[i] = scan.nextInt(); //Continues to read numbers and add them to the sum
n = n + 1; //Adds to the array maximum
sum = sum + num[i]; //Calculates the sum
if (num[i] == -1){
break;
}
}
System.out.print("The numbers entered are: " + num[i]);
System.out.print("\nThe sum of the numbers is: " + sum);
/******************************************************************************
* Outputs Section *
******************************************************************************/
//***************Output is in the processing**************************//
}
}
問題是,程序一直掛在應該打印總和的行上。 任何和所有幫助表示贊賞!
代替使用數組(因為它限制了您的輸入數量),您可以使用一個臨時變量來計算總和的值。 如下圖所示:
int sum=0;
int num=0;
while(num != -1) {
sum = sum + num;
num = scan.nextInt(); //note that the variables can be reused
}
您可以使用列表來存儲數字,並可以使用無限循環來不斷接收來自用戶的輸入。 另外,您應該在開始處理數字之前檢查停止條件(因為您的問題提到-1不是輸出的一部分)。 這是一個例子
import java.util.*;
import java.io.*;
public class InputSum
{
public static void main (String[] args)
{
/******************************************************************************
* Declarations Section *
******************************************************************************/
/****************************CONSTANTS********************************/
Scanner scan = new Scanner(System.in); //Initializes scanner
int number; //Declare a variable that will hold the temporal value that is read on the input stream
int sum=0;
// Use a List
List<Integer> numbers = new ArrayList<Integer>();
/******************************************************************************
* Inputs Section *
******************************************************************************/
System.out.print("Please input integers, note that -1 ends the submissions: "); //Prompts the user for input
/****************************variables********************************/
//***************************Calculations in processing************************//
/******************************************************************************
* Processing Section *
******************************************************************************/
// use an infinite loop
for(; ; )
{
// You should normally do this check when you enter the loop
// so that -1 which is a stop token should not be added to the list
// and not taken into account in the sum
number = scan.nextInt(); //Continues to read numbers and add them to the sum
if (number == -1){
break;
}
// You could write numbers.add(number) which would be
// Java's autoboxing feature, but this is what would really take place
numbers.add(Integer.valueOf(number));
sum += number; //Calculates the sum
}
System.out.print("The numbers entered are: " + numbers);
System.out.print("\nThe sum of the numbers is: " + sum);
/******************************************************************************
* Outputs Section *
******************************************************************************/
//***************Output is in the processing**************************//
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.