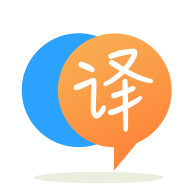
[英]How Do You Iterate Through An JObject If You Are Deleting Certain Elements?
[英]How do you iterate through every day of the year?
鑒於開始日期為2009年1月1日,結束日期為12/31/2009,如何迭代每個日期並使用c#檢索DateTime值?
謝謝!
我會使用一個看起來像這樣的循環
for(DateTime date = begin; date <= end; date = date.AddDays(1))
{
}
相應地設置開始和結束
實現Iterator設計模式的另一個選擇:
這可能聽起來沒必要,但我依賴於您如何使用此功能,您也可以實現Iterator設計模式 。
想一想。 假設一切正常,你復制/粘貼“for”句子。 突然作為要求的一部分,你必須迭代所有的日子,但跳過其中一些(如日歷,跳過瞻禮,周末,習慣等)
您必須創建一個新的“剪切”並改為使用日歷。 然后搜索並替換所有你的。
在OOP中,這可以使用Iterator模式來實現。
來自Wikpedia:
在面向對象的編程中,Iterator模式是一種設計模式,其中迭代器用於順序訪問聚合對象的元素, 而不暴露其底層表示 。 Iterator對象封裝了迭代發生方式的內部結構。
所以我的想法是使用這樣的結構:
DateTime fromDate = DateTime.Parse("1/1/2009");
DateTime toDate = DateTime.Parse("12/31/2009");
// Create an instance of the collection class
DateTimeEnumerator dateTimeRange =
new DateTimeEnumerator( fromDate, toDate );
// Iterate with foreach
foreach (DateTime day in dateTimeRange )
{
System.Console.Write(day + " ");
}
然后,如果需要,您可以創建子類來實現不同的算法,一個使用AddDay(1),另一個使用AddDay(7)或其他簡單使用Calendar。 等等
想法是降低對象之間的耦合。
同樣,對於大多數情況來說,這將是過度的,但如果迭代形成系統的相關部分(假設您正在為企業創建某種類型的通知,並且應該遵循不同的全球化)
當然,基本實現將使用for。
public class DateTimeEnumerator : System.Collections.IEnumerable
{
private DateTime begin;
private DateTime end;
public DateTimeEnumerator ( DateTime begin , DateTime end )
{
// probably create a defensive copy here...
this.begin = begin;
this.end = end;
}
public System.Collections.IEnumerator GetEnumerator()
{
for(DateTime date = begin; date < end; date = date.AddDays(1))
{
yield return date;
}
}
}
只是一個想法:)
DateTime dateTime = new DateTime(2009, 1, 1);
while(dateTime.Year < 2010)
{
dateTime = dateTime.AddDays(1);
}
我使用MiscUtil及其擴展方法:
foreach(DateTime date in 1.January(2009)
.To(31.December(2009))
.Step(1.Days())
{
Console.WriteLine(date);
}
設置兩個變量:
DateTime lowValue = DateTime.Parse("1/1/2009");
DateTime highValue = DateTime.Parse("12/31/2009");
然后,將一天添加到低值,直到它等於highvalue:
while (lowValue <= highValue)
{
//Do stuff here
lowValue = lowValue.AddDays(1);
}
或類似的東西。
可能更可重用的替代方法是在DateTime上編寫擴展方法並返回IEnumerable。
例如,您可以定義一個類:
public static class MyExtensions
{
public static IEnumerable EachDay(this DateTime start, DateTime end)
{
// Remove time info from start date (we only care about day).
DateTime currentDay = new DateTime(start.Year, start.Month, start.Day);
while (currentDay <= end)
{
yield return currentDay;
currentDay = currentDay.AddDays(1);
}
}
}
現在在調用代碼中,您可以執行以下操作:
DateTime start = DateTime.Now;
DateTime end = start.AddDays(20);
foreach (var day in start.EachDay(end))
{
...
}
這種方法的另一個優點是它使添加EveryWeek,EachMonth等變得微不足道。然后,這些都可以在DateTime上訪問。
int day; for (int i = 1; i<365;i++) { day++; }
對不起,忍不住了。
DateTime current = DateTime.Parse("1/1/2009");
DateTime nextYear = current.AddYears(1);
do
{
Console.WriteLine(current);
current = current.AddDays(1);
} while (current < nextYear) ;
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.