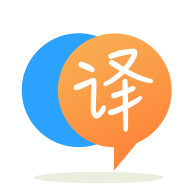
[英]C# How to represent (unsigned long)(unsigned int) (ulong)(uint) with XOR (^)
[英]Cast negative number to unsigned types (ushort, uint or ulong)
如何將一些負數轉換為unsigned types
。
Type type = typeof (ushort);
short num = -100;
ushort num1 = unchecked ((ushort) num); //When type is known. Result 65436
ushort num2 = unchecked(Convert.ChangeType(num, type)); //Need here the same value
只有4種類型。 所以,你只需編寫自己的方法即可。
private static object CastToUnsigned(object number)
{
Type type = number.GetType();
unchecked
{
if (type == typeof(int)) return (uint)(int)number;
if (type == typeof(long)) return (ulong)(long)number;
if (type == typeof(short)) return (ushort)(short)number;
if (type == typeof(sbyte)) return (byte)(sbyte)number;
}
return null;
}
以下是測試:
short sh = -100;
int i = -100;
long l = -100;
Console.WriteLine(CastToUnsigned(sh));
Console.WriteLine(CastToUnsigned(i));
Console.WriteLine(CastToUnsigned(l));
輸出
65436
4294967196
18446744073709551516
更新10/10/2017
對於泛型類型的C#7.1模式匹配功能,您現在可以使用switch語句。
感謝@quinmars的建議。
private static object CastToUnsigned<T>(T number) where T : struct
{
unchecked
{
switch (number)
{
case long xlong: return (ulong) xlong;
case int xint: return (uint)xint;
case short xshort: return (ushort) xshort;
case sbyte xsbyte: return (byte) xsbyte;
}
}
return number;
}
如果您可以使用unsafe
代碼,您可以隨時編寫這樣的函數並使用指針進行轉換:
public static unsafe TTo Convert<TFrom, TTo>(TFrom value) where TFrom : unmanaged where TTo : unmanaged
{
if (sizeof(TFrom) != sizeof(TTo))
{
throw new ArgumentException("Source and target types must be the same size!");
}
return *(TTo*)&value;
}
你會像這樣使用:
short num = -100;
ushort uNum = Convert<short, ushort>(num);
這里的好處是沒有裝箱,沒有類型檢查,無需分配代碼,如果您大膽並且知道自己在做什么,您甚至可以刪除大小檢查以獲得無分支的實現。 這里的明顯缺點是unsafe
關鍵字,它在許多業務應用程序中通常是unsafe
,並且通用實現將允許將任何非托管結構轉換為任何其他非托管結構,只要sizeof
表示它們具有相同的大小(不會深入研究整個sizeof
與Marshal.SizeOf()
事情在這里)。 如果您知道您只是在轉換原始整數類型,那應該沒問題。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.