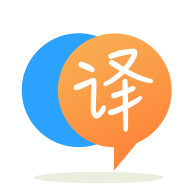
[英]How can I merge two almost identical javascript objects into one using Lodash?
[英]How to merge two almost identical arrays/objects (with underscorejs or similiar libary)?
我們得到了一個舊清單和一個新清單。 即使添加了一些新的鍵值對,該計划也要合並兩者。
var oldList = [{
id: 1,
name: 'Michael',
sex: 'male',
goodlooking: 1
}, {
id: 2,
name: 'John',
sex: 'male'
}, {
id: 3,
name: 'Laura',
sex: 'female'
}];
和...
var newList = [{
id: 1,
name: 'Cindy',
sex: 'female'
}, {
id: 2,
name: 'Herry',
sex: 'male'
}, {
id: 3,
name: 'Laura',
sex: 'female',
goodlooking: 1
}];
現在,我嘗試將兩者合並在一起,並通過替換相等鍵的值來充分利用兩者。 特別是合並后的列表應如下所示:
var mergedList = [{
id: 1,
name: 'Cindy',
sex: 'female',
goodlooking: 1
}, {
id: 2,
name: 'Herry',
sex: 'male'
}, {
id: 3,
name: 'Laura',
sex: 'female',
goodlooking: 1
}];
邁克爾改變了名字和性別,但保持好看。 約翰(John)改名亨利(Henry),勞拉(Laura)發現了她的內在美。
var mergedList = _.map(oldList, function (oldElem) {
var newElem = _.find(newList, {id: oldElem.id});
return _.merge(oldElem, newElem);
});
用純Javascript解決方案:
var oldList = [{ id: 1, name: 'Michael', sex: 'male', goodlooking: 1 }, { id: 2, name: 'John', sex: 'male' }, { id: 3, name: 'Laura', sex: 'female' }], newList = [{ id: 1, name: 'Cindy', sex: 'female' }, { id: 2, name: 'Herry', sex: 'male' }, { id: 3, name: 'Laura', sex: 'female', goodlooking: 1 }], theList = JSON.parse(JSON.stringify(oldList)); // make copy newList.forEach(function (a) { theList.some(function (b) { if (a.id === b.id) { Object.keys(a).forEach(function (k) { b[k] = a[k]; }); return true; } }); }); document.write('<pre>' + JSON.stringify(theList, 0, 4) + '</pre>');
像這樣的東西?
將使用newList中可用的值覆蓋oldList中的所有內容,並添加新值(如果存在)。
mergedList = [];
_.each(oldList, function(listItem, index){
copy = listItem;
newValues = _.findWhere(newList, {"id": listItem.id});
_.each(newValues, function(val,key){
copy[key] = val;
});
mergedList[index] = copy
});
像這樣:
var a = oldList.length,
mergedList = [];
while(a--){
var oldData = oldList[a];
newKeys = Object.keys(newList[a]);
for (var i=0;i<newKeys.length;i++){
var key = newKeys[i];
oldData[key] = newList[a][key]
}
}
直接使用lodash的merge方法。
var mergedList = _.merge(oldList, newList);
它遞歸合並自己的可枚舉屬性。
https://lodash.com/docs#merge
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.