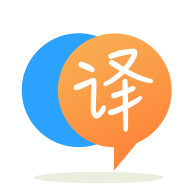
[英]How to change background color of checkbox when checkbox is IsChecked in XAML in windows phone 8.1
[英]change the background of a border when checkbox ischecked
我能夠使用選中的事件更改邊框背景的顏色,並且每次選中/取消選中手動更改背景顏色的單選按鈕,但是我想使用轉換器或可視狀態管理器或任何其他有效的方法來實現相同的效果,因此如何實現它。這是xaml
<Border x:Name="br1" Background="Blue" >
<RadioButton Checked="radio1_Checked" x:Name="radio1" />
</Border>
<Border x:Name="br2" Background="Blue" >
<RadioButton Checked="radio2_Checked" x:Name="radio2" />
</Border>
我想在選中和取消選中單選按鈕時更改邊框背景的顏色,以便如何實現
可能有幾種不同的解決方案,具體取決於您要處理的工作量,靈活性和可重復使用性。
編輯:以下代碼適用於Windows(Phone)8.1 RT,不適用於Windows Phone Silverlight。 不過,將其留在此處以供將來參考,因為無論如何,Silverlight仍將由Windows 10上的Windows運行時代替。
由於標准RadioButton
已經在其可視化樹中內置了邊框,並且為了實現最高的靈活性和可重復使用性,我創建了一個自RadioButton
派生的自定義控件(在Visual Studio項目模板中稱為模板化控件 )。
我提供了CheckedBrush
和UncheckedBrush
(默認為紅色/透明),因此您可以根據需要進行自定義。 在XAML中設置這些值之一將覆蓋默認值。
自定義控件的代碼:
public sealed class MyRadioButton : RadioButton
{
public MyRadioButton()
{
this.DefaultStyleKey = typeof(MyRadioButton);
UnCheckedBrush = new SolidColorBrush(Colors.Transparent);
CheckedBrush = new SolidColorBrush(Colors.Red);
this.Checked += (sender, args) =>
{
this.CustomBackground = CheckedBrush;
};
this.Unchecked += (sender, args) =>
{
this.CustomBackground = UnCheckedBrush;
};
}
public static readonly DependencyProperty CustomBackgroundProperty = DependencyProperty.Register(
"CustomBackground", typeof (Brush), typeof (MyRadioButton), new PropertyMetadata(default(Brush)));
public static readonly DependencyProperty CheckedBrushProperty = DependencyProperty.Register(
"CheckedBrush", typeof(Brush), typeof(MyRadioButton), new PropertyMetadata(default(Brush)));
public static readonly DependencyProperty UnCheckedBrushProperty = DependencyProperty.Register(
"UnCheckedBrush", typeof(Brush), typeof(MyRadioButton), new PropertyMetadata(default(Brush)));
public Brush CustomBackground
{
get { return (Brush) GetValue(CustomBackgroundProperty); }
set { SetValue(CustomBackgroundProperty, value); }
}
public Brush CheckedBrush
{
get { return (Brush) GetValue(CheckedBrushProperty); }
set { SetValue(CheckedBrushProperty, value); }
}
public Brush UnCheckedBrush
{
get { return (Brush) GetValue(UnCheckedBrushProperty); }
set { SetValue(UnCheckedBrushProperty, value); }
}
}
下一步是在Themes / Generic.xaml文件中提供樣式,這是與默認RadioButton樣式相比稍作調整的樣式(用於背景):
<Style TargetType="local:MyRadioButton">
<Setter Property="Foreground" Value="{ThemeResource RadioButtonContentForegroundThemeBrush}"/>
<Setter Property="Padding" Value="1,4,0,0" />
<Setter Property="HorizontalAlignment" Value="Stretch" />
<Setter Property="VerticalAlignment" Value="Center" />
<Setter Property="HorizontalContentAlignment" Value="Left" />
<Setter Property="VerticalContentAlignment" Value="Top" />
<Setter Property="FontFamily" Value="{ThemeResource ContentControlThemeFontFamily}" />
<Setter Property="FontSize" Value="{ThemeResource ControlContentThemeFontSize}" />
<Setter Property="Template">
<Setter.Value>
<ControlTemplate TargetType="local:MyRadioButton">
<Border Background="{TemplateBinding CustomBackground}"
BorderBrush="{TemplateBinding BorderBrush}"
BorderThickness="{TemplateBinding BorderThickness}">
<VisualStateManager.VisualStateGroups>
<VisualStateGroup x:Name="CommonStates">
<VisualState x:Name="Normal" />
<VisualState x:Name="PointerOver">
<Storyboard>
<ObjectAnimationUsingKeyFrames Storyboard.TargetName="BackgroundEllipse"
Storyboard.TargetProperty="Fill">
<DiscreteObjectKeyFrame KeyTime="0" Value="{ThemeResource RadioButtonPointerOverBackgroundThemeBrush}" />
</ObjectAnimationUsingKeyFrames>
<ObjectAnimationUsingKeyFrames Storyboard.TargetName="BackgroundEllipse"
Storyboard.TargetProperty="Stroke">
<DiscreteObjectKeyFrame KeyTime="0" Value="{ThemeResource RadioButtonPointerOverBorderThemeBrush}" />
</ObjectAnimationUsingKeyFrames>
<ObjectAnimationUsingKeyFrames Storyboard.TargetName="CheckGlyph"
Storyboard.TargetProperty="Fill">
<DiscreteObjectKeyFrame KeyTime="0" Value="{ThemeResource RadioButtonPointerOverForegroundThemeBrush}" />
</ObjectAnimationUsingKeyFrames>
</Storyboard>
</VisualState>
<VisualState x:Name="Pressed">
<Storyboard>
<ObjectAnimationUsingKeyFrames Storyboard.TargetName="BackgroundEllipse"
Storyboard.TargetProperty="Fill">
<DiscreteObjectKeyFrame KeyTime="0" Value="{ThemeResource RadioButtonPressedBackgroundThemeBrush}" />
</ObjectAnimationUsingKeyFrames>
<ObjectAnimationUsingKeyFrames Storyboard.TargetName="BackgroundEllipse"
Storyboard.TargetProperty="Stroke">
<DiscreteObjectKeyFrame KeyTime="0" Value="{ThemeResource RadioButtonPressedBorderThemeBrush}" />
</ObjectAnimationUsingKeyFrames>
<ObjectAnimationUsingKeyFrames Storyboard.TargetName="CheckGlyph"
Storyboard.TargetProperty="Fill">
<DiscreteObjectKeyFrame KeyTime="0" Value="{ThemeResource RadioButtonPressedForegroundThemeBrush}" />
</ObjectAnimationUsingKeyFrames>
</Storyboard>
</VisualState>
<VisualState x:Name="Disabled">
<Storyboard>
<ObjectAnimationUsingKeyFrames Storyboard.TargetName="BackgroundEllipse"
Storyboard.TargetProperty="Fill">
<DiscreteObjectKeyFrame KeyTime="0" Value="{ThemeResource RadioButtonDisabledBackgroundThemeBrush}" />
</ObjectAnimationUsingKeyFrames>
<ObjectAnimationUsingKeyFrames Storyboard.TargetName="BackgroundEllipse"
Storyboard.TargetProperty="Stroke">
<DiscreteObjectKeyFrame KeyTime="0" Value="{ThemeResource RadioButtonDisabledBorderThemeBrush}" />
</ObjectAnimationUsingKeyFrames>
<ObjectAnimationUsingKeyFrames Storyboard.TargetName="CheckGlyph"
Storyboard.TargetProperty="Fill">
<DiscreteObjectKeyFrame KeyTime="0" Value="{ThemeResource RadioButtonDisabledForegroundThemeBrush}" />
</ObjectAnimationUsingKeyFrames>
<ObjectAnimationUsingKeyFrames Storyboard.TargetName="ContentPresenter"
Storyboard.TargetProperty="Foreground">
<DiscreteObjectKeyFrame KeyTime="0" Value="{ThemeResource RadioButtonContentDisabledForegroundThemeBrush}" />
</ObjectAnimationUsingKeyFrames>
</Storyboard>
</VisualState>
</VisualStateGroup>
<VisualStateGroup x:Name="CheckStates">
<VisualState x:Name="Checked">
<Storyboard>
<DoubleAnimation Storyboard.TargetName="CheckGlyph"
Storyboard.TargetProperty="Opacity"
To="1"
Duration="0" />
</Storyboard>
</VisualState>
<VisualState x:Name="Unchecked" />
<VisualState x:Name="Indeterminate" />
</VisualStateGroup>
<VisualStateGroup x:Name="FocusStates">
<VisualState x:Name="Focused">
<Storyboard>
<DoubleAnimation Storyboard.TargetName="FocusVisualWhite"
Storyboard.TargetProperty="Opacity"
To="1"
Duration="0" />
<DoubleAnimation Storyboard.TargetName="FocusVisualBlack"
Storyboard.TargetProperty="Opacity"
To="1"
Duration="0" />
</Storyboard>
</VisualState>
<VisualState x:Name="Unfocused" />
<VisualState x:Name="PointerFocused" />
</VisualStateGroup>
</VisualStateManager.VisualStateGroups>
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="29" />
<ColumnDefinition Width="*" />
</Grid.ColumnDefinitions>
<Grid VerticalAlignment="Top">
<Ellipse x:Name="BackgroundEllipse"
Width="23"
Height="23"
UseLayoutRounding="False"
Fill="{ThemeResource RadioButtonBackgroundThemeBrush}"
Stroke="{ThemeResource RadioButtonBorderThemeBrush}"
StrokeThickness="{ThemeResource RadioButtonBorderThemeThickness}" />
<Ellipse x:Name="CheckGlyph"
Width="13"
Height="13"
UseLayoutRounding="False"
Opacity="0"
Fill="{ThemeResource RadioButtonForegroundThemeBrush}" />
<Rectangle x:Name="FocusVisualWhite"
Stroke="{ThemeResource FocusVisualWhiteStrokeThemeBrush}"
StrokeEndLineCap="Square"
StrokeDashArray="1,1"
Opacity="0"
StrokeDashOffset="1.5"
Width="29"
Height="29" />
<Rectangle x:Name="FocusVisualBlack"
Stroke="{ThemeResource FocusVisualBlackStrokeThemeBrush}"
StrokeEndLineCap="Square"
StrokeDashArray="1,1"
Opacity="0"
StrokeDashOffset="0.5"
Width="29"
Height="29" />
</Grid>
<ContentPresenter x:Name="ContentPresenter"
Content="{TemplateBinding Content}"
ContentTransitions="{TemplateBinding ContentTransitions}"
ContentTemplate="{TemplateBinding ContentTemplate}"
Margin="{TemplateBinding Padding}"
HorizontalAlignment="{TemplateBinding HorizontalContentAlignment}"
VerticalAlignment="{TemplateBinding VerticalContentAlignment}"
Grid.Column="1"
AutomationProperties.AccessibilityView="Raw"/>
</Grid>
</Border>
</ControlTemplate>
</Setter.Value>
</Setter>
</Style>
從默認模板更改的唯一內容是刪除Background屬性並更改Border控件上的Background。
大家都准備使用它:
<StackPanel Margin="100">
<RadioButton Content="Check 1" />
<local:MyRadioButton CheckedBrush="Blue" Content="Check 2" />
<local:MyRadioButton CheckedBrush="Green" Content="Check 3" />
</StackPanel>
我將實現一個視圖模型類(請參閱MVVM pattern ),並實現兩個DependencyPropery的一個復選框狀態,一個帶有邊框的顏色。 使用數據綁定將相應的XAML屬性綁定到這些屬性。 復選框狀態更改時,還會引發邊框顏色的PropertyChanged事件。 做完了
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.