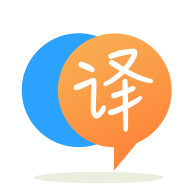
[英]How to create single dimensional, two dimensional,three dimensional as well as multi dimensional array using C#?
[英]How can I create a two dimensional array that containts Ints and Strings in C#?
我正在嘗試使用C#創建一個ATM模擬器,並且有點卡住了登錄。我正在使用一個名為User的類,其定義如下:
public class User
{
public string userName { get; set; }
public string password { get; set; }
public int savingsAcct { get; set; }
public int checkAcct { get; set; }
}
我已經創建了班級的三個實例來代表我將要使用的三個帳戶。 所以我想知道如何制作一個可以同時接受字符串和整數的二維數組。
我想可以將二維數組用於日志,因為可以使用for循環遍歷該數組並檢查每個帳戶的用戶名和密碼,以查看它們是否匹配。 我知道我可以使用數據庫或其他東西,但是我對C#還是比較陌生,所以我不是在尋找高效的東西,而是在尋找有效的東西。 任何幫助將非常感激。
您應該真正學習使用數據庫的一些基礎知識。 您的User
類被稱為Model,而您正在尋找的是集合。
首先,我建議添加id
字段,以使其更容易識別每個對象(盡管它是可選的):
public class User
{
public int id { get; set; }
public string userName { get; set; }
public string password { get; set; }
public int savingsAcct { get; set; }
public int checkAcct { get; set; }
}
您可以使用List<User>
:
List<User> users = new List<User>()
{
new User() { id = 0, userName = "Alex", etc... }
new User() { id = 1, userName = "Joshua", etc... }
new User() { id = 2, userName = "Phil", etc... }
};
要查找具有特定userName的用戶,您必須在此處使用LINQ:
// returns null if no such user is found
User alex = users.FirstOrDefault(u => u.userName == "Alex");
有時使用Dictionary<TKey, TValue>
比List<T>
更合適:
Dictionary<string, User> users = new Dictionary<string, User>()
{
{ "Alex", new User() { id = 0, userName = "Alex", etc... } },
{ "Joshua", new User() { id = 1, userName = "Joshua", etc... } },
{ "Phil", new User() { id = 2, userName = "Phil", etc... } },
};
然后,您可以在indexer的幫助下通過用戶名訪問用戶:
User alex = users["Alex"];
這顯示了一個有效的示例,但需要深入研究您的代碼:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace ConsoleApplication1
{
public class User
{
public string userName { get; set; }
public string password { get; set; }
public int savingsAcct { get; set; }
public int checkAcct { get; set; }
}
class Program
{
static void Main(string[] args)
{
// here you'll take the user and password from the form
string userNameEnteredIntoScreen = "???";
string passwordEnteredIntoScreen = "???";
// build list of valid users (amend to suit)
var users = new List<User>();
users.Add(new User
{
userName = "user1",
password = "abc",
savingsAcct = 1,
checkAcct = 2,
});
users.Add(new User
{
userName = "user2",
password = "xyz",
savingsAcct = 3,
checkAcct = 4,
});
// then to check if they're valid
var user = users.SingleOrDefault(u =>
u.userName == userNameEnteredIntoScreen &&
u.password == passwordEnteredIntoScreen
);
if (user != null)
{
// the user and password was valid, and 'user' variable
// now contains the details of the user
}
}
}
}
正如注釋中所闡明的,您正在尋找一個可以在應用程序中存儲User
對象的集合。
如果您需要能夠更改集合的內容,則List<T>
是一個不錯的選擇-它沒有固定的大小,因此可以輕松添加和刪除對象。
List<User> _allUsers = new List<User>();
_allUsers.Add(/* user object */); //populate the list with Users using .Add()
foreach (var user in _allUsers)
{
if(user.userName == someInputVariable && user.passWord == someOtherInputVariable)
{
...
}
}
您還可以使用一維User
數組,但我認為List<T>
對於您要嘗試做的事情更有意義,並且與剛開始學習該語言的人一起工作非常容易。
當您要存儲User
對象時,請保持其格式不變,而不要試圖將它們不適當地擠壓成某種字符串/整數組合。 因此,讓seay有3個用戶對象:
var bob = new User { userName = "Bob" };
var alice = new User { userName = "Alice" };
var marvin = new User { userName = "Marvin" };
讓我們將它們放入List<>
:
var users = new List<User> { bob, alice, marvin };
現在,您可以按需循環:
foreach(var user in users)
{
Console.WriteLine(user.userName);
}
而且,如果您想查找特定項目,則可以快速獲得:
var user = users.SingleOrDefault(u => u.userName = "Bob");
if (user != null)
{
//We found a user called Bob
}
甚至專門檢查用戶名和密碼:
var userIsValid = users.Any(u => u.userName = "Bob" && u.password = "secret");
在C#中,您不能使用int和string兩個不同的數據類型創建一個二維數組。 您只能創建相同數據類型的二維數組。
如果您需要一個數據結構來容納兩種數據類型,則可以使用字典對。
//e.g.
Dictionary<int, string> data = new Dictionary<int, string>
{
{1, "value 1"},
{2, "value 2"},
{3, "value 3"}
};
//With your POCO can create a Dictionary as below
Dictionary<int, User> data = new Dictionary<int, User>
{
{1, new User{ userName = "username1", password = "password1", savingsAcct = 1,checkAcct = 1 }},
{2, new User{ userName = "username2", password = "password2", savingsAcct = 1,checkAcct = 2 }},
{3, new User{ userName = "username3", password = "password3", savingsAcct = 1,checkAcct = 3}}
};
您可以使用循環來初始化字典
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.