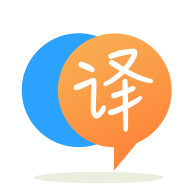
[英]Push Toast Notifications for windows Phone 8.1 by pushwoosh
[英]Understanding Toast push notifications and how to display text on them and launch app by pressing on them. Windows Phone 8.1
我試圖將我的應用程序設置為接收來自服務器的吐司推送通知。
由於此服務器由其他人處理,並且他沒有向WSN請求令牌,因此,我按照一個示例進行操作,並使用“本地網頁”發送通知。
protected void ButtonSendToast_Click(object sender, EventArgs e)
{
try
{
// Get the URI that the Microsoft Push Notification Service returns to the push client when creating a notification channel.
// Normally, a web service would listen for URIs coming from the web client and maintain a list of URIs to send
// notifications out to.
string subscriptionUri = TextBoxUri.Text.ToString();
HttpWebRequest sendNotificationRequest = (HttpWebRequest)WebRequest.Create(subscriptionUri) as HttpWebRequest;
// Create an HTTPWebRequest that posts the toast notification to the Microsoft Push Notification Service.
// HTTP POST is the only method allowed to send the notification.
sendNotificationRequest.Method = "POST";
// The optional custom header X-MessageID uniquely identifies a notification message.
// If it is present, the same value is returned in the notification response. It must be a string that contains a UUID.
// sendNotificationRequest.Headers.Add("X-MessageID", "<UUID>");
// Create the toast message.
string toastMessage = "<?xml version=\"1.0\" encoding=\"utf-8\"?>" +
"<wp:Notification xmlns:wp=\"WPNotification\">" +
"<wp:Toast>" +
"<wp:Text1>" + TextBoxTitle.Text.ToString() + "</wp:Text1>" +
"<wp:Text2>" + TextBoxSubTitle.Text.ToString() + "</wp:Text2>" +
"<wp:Param>/Page2.xaml?NavigatedFrom=Toast Notification</wp:Param>" +
"</wp:Toast> " +
"</wp:Notification>";
// Set the notification payload to send.
byte[] notificationMessage = Encoding.Default.GetBytes(toastMessage);
// Set the web request content length.
sendNotificationRequest.Headers.Add("Authorization", String.Format("Bearer {0}", "EgAdAQMAAAAEgAAAC4AATIYp8fmpjFpbdnRTjf2qfP/GqZ8Bbb62bH6N+0MhSztcV/wXfv9aVjiwbVgF5EX0fgBXC6LvJCpl1+ze7ts9h5je4e1QekryEFqfWl36BtTBnmWqBFk0WmwxpdIgGqhVjAtRdnJ3ODnFSBCfd7dq8nFiFTFDxPcTXhdDbu9W3BKMAFoAjAAAAAAAHFAXTMH+bVbB/m1W60gEAA8AMTkwLjE5My42OS4yMzMAAAAAAF0AbXMtYXBwOi8vcy0xLTE1LTItMTU5OTEyNjk1NS0zODAwNDMxNzQ0LTk2OTg4NTEzNi0xNjkxMDU1MjI4LTcwOTcyNTQ0NC00MDYxNzA4MDczLTI0Mzg0MzM1MzQA"));
sendNotificationRequest.ContentLength = notificationMessage.Length;
sendNotificationRequest.ContentType = "text/xml";
sendNotificationRequest.Headers.Add("X-WNS-Type", "wns/toast");
using (Stream requestStream = sendNotificationRequest.GetRequestStream())
{
requestStream.Write(notificationMessage, 0, notificationMessage.Length);
}
// Send the notification and get the response.
HttpWebResponse response = (HttpWebResponse)sendNotificationRequest.GetResponse();
string notificationStatus = response.Headers["X-NotificationStatus"];
string notificationChannelStatus = response.Headers["X-SubscriptionStatus"];
string deviceConnectionStatus = response.Headers["X-DeviceConnectionStatus"];
// Display the response from the Microsoft Push Notification Service.
// Normally, error handling code would be here. In the real world, because data connections are not always available,
// notifications may need to be throttled back if the device cannot be reached.
TextBoxResponse.Text = notificationStatus + " | " + deviceConnectionStatus + " | " + notificationChannelStatus;
}
catch (Exception ex)
{
TextBoxResponse.Text = "Exception caught sending update: " + ex.ToString();
}
}
現在,在我的應用程序中,我已請求頻道uri和一個處理程序,當該頻道收到推送請求時會調用
PushNotificationChannel channel = null;
try
{
channel = await PushNotificationChannelManager.CreatePushNotificationChannelForApplicationAsync();
Debug.WriteLine(channel.Uri);
if (channel.Uri != null)
{
var localSettings = Windows.Storage.ApplicationData.Current.LocalSettings;
localSettings.Values.Remove("PushToken");
localSettings.Values["PushToken"] = channel.Uri;
channel.PushNotificationReceived += channel_PushNotificationReceived;
}
}
catch (Exception ex)
{
Debug.WriteLine(ex.ToString());
}
}
async void channel_PushNotificationReceived(PushNotificationChannel sender, PushNotificationReceivedEventArgs e)
{
String notificationContent = String.Empty;
notificationContent = e.ToastNotification.Content.GetXml();
Debug.WriteLine(notificationContent);
}
到目前為止,一切都很好:當我的應用程序運行時和關閉時,我都會收到通知。 但是它只顯示“新通知”,當我單擊它時什么也沒有發生。
我試圖添加一個活動
e.ToastNotification.Activated += ToastNotification_Activated;
但這是行不通的,在閱讀了20份文檔后,我對吐司模板以及如何使用它顯示從服務器接收到的內容感到非常困惑
那么,執行此操作,在吐司中顯示推送中收到的一些數據以及使應用程序在用戶單擊時“啟動/轉到特定頁面”的真正方法是什么?
讓應用轉到特定頁面
從Windows 8 / 8.1開始,始終希望應用程序能夠處理激活,以響應用戶單擊該應用程序上的Toast通知–該應用程序應通過執行導航和顯示特定於該Toast的UI進行響應。 這是通過包含在Toast有效負載中的激活字符串完成的,然后將其作為激活事件中的參數傳遞給您的應用。
對於導航到特定頁面,您需要重寫App.Xaml.cs中的OnActivated事件並處理字符串參數。
對於顯示吐司如果要在圖塊中顯示文本,則嘗試使用“徽章”通知或僅通過吐司接收的數據,然后在從onactivated事件導航后在頁面上對其進行處理。 同時檢查此鏈接 。 它顯示了用於不同通知的標准模板。 希望能幫助到你。
當系統無法識別您的吐司通知有效載荷時,您會收到“新通知”。 那是因為您使用的是MPNS快速入門 ,而不是WNS快速入門,並且除了吐司通知格式以外,大部分內容都移至WNS。 您希望您的吐司內容看起來像這樣:
<toast launch="">
<visual lang="en-US">
<binding template="ToastImageAndText01">
<image id="1" src="World" />
<text id="1">Hello</text>
</binding>
</visual>
</toast>
這是WNS快速入門指南: https : //msdn.microsoft.com/zh-cn/library/windows/apps/xaml/hh868252.aspx
以及WNS吐司架構: https : //msdn.microsoft.com/en-us/library/windows/apps/br230849.aspx
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.