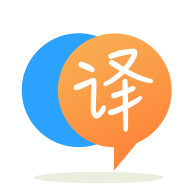
[英]WPF TreeView (hierarchicaldatatemplate ) with 2 tables
[英]WPF MVVM TreeView using HierarchicalDataTemplate not updating
所以我一直在努力讓我的TreeViews在很長一段時間內正確更新,所以我問是否有人能告訴我為什么我的代碼沒有在添加或減少時正確更新我的TreeView節點。 我提前為有點大規模的代碼轉儲道歉,但我覺得解釋這個問題非常重要。
對於初學者我的ObservableObject類
public abstract class ObservableObject : INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
[NotifyPropertyChangedInvocator]
protected virtual void OnPropertyChanged([CallerMemberName] string propertyName = null)
{
PropertyChangedEventHandler handler = this.PropertyChanged;
if (handler != null)
{
handler(this, new PropertyChangedEventArgs(propertyName));
}
}
}
TreeNodeBase類
public abstract class TreeNodeBase : ObservableObject
{
protected const string ChildNodesPropertyName = "ChildNodes";
protected string name;
public string Name
{
get
{
return this.name;
}
set
{
this.name = value;
this.OnPropertyChanged();
}
}
protected IList<TreeNode> childNodes;
protected TreeNodeBase(string name)
{
this.Name = name;
this.childNodes = new List<TreeNode>();
}
public IEnumerable<TreeNode> ChildNodes
{
get
{
return this.childNodes;
}
}
public TreeNodeBase AddChildNode(string name)
{
var treeNode = new TreeNode(this, name);
this.childNodes.Add(treeNode);
this.OnPropertyChanged(ChildNodesPropertyName);
return treeNode;
}
public TreeNode RemoveChildNode(string name)
{
var nodeToRemove = this.childNodes.FirstOrDefault(node => node.Name.Equals(name));
if (nodeToRemove != null)
{
this.childNodes.Remove(nodeToRemove);
this.OnPropertyChanged(ChildNodesPropertyName);
}
return nodeToRemove;
}
}
public class TreeNode : TreeNodeBase
{
public TreeNodeBase Parent { get; protected set; }
public TreeNode(TreeNodeBase parent, string name)
: base(name)
{
this.Parent = parent;
}
}
TreeNodeRoot類
public class TreeViewRoot : TreeNodeBase
{
public TreeViewRoot(string name)
: base(name)
{
}
}
TreeNode類
public class TreeNode : TreeNodeBase
{
public TreeNodeBase Parent { get; protected set; }
public TreeNode(TreeNodeBase parent, string name)
: base(name)
{
this.Parent = parent;
}
}
TreeView UserControl Xaml
<UserControl x:Class="TreeViewExperiment.TreeView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:treeViewExperiment="clr-namespace:TreeViewExperiment"
xmlns:i="http://schemas.microsoft.com/expression/2010/interactivity"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="400"
d:DataContext="{d:DesignInstance treeViewExperiment:TreeViewmodel}">
<UserControl.DataContext>
<treeViewExperiment:TreeViewmodel/>
</UserControl.DataContext>
<Grid Background="White">
<Grid.Resources>
<HierarchicalDataTemplate x:Key="TreeViewHierarchicalTemplate" ItemsSource="{Binding ChildNodes}">
<TextBlock Text="{Binding Name}"/>
</HierarchicalDataTemplate>
<Style TargetType="Button">
<Setter Property="FontFamily" Value="Verdana"/>
<Setter Property="FontWeight" Value="Bold"/>
</Style>
</Grid.Resources>
<Grid.RowDefinitions>
<RowDefinition Height="6*"/>
<RowDefinition Height="Auto"/>
<RowDefinition Height="Auto"/>
</Grid.RowDefinitions>
<TreeView Grid.Row="0" x:Name="Tree" ItemsSource="{Binding RootLevelNodes}" ItemTemplate="{StaticResource TreeViewHierarchicalTemplate}">
<i:Interaction.Triggers>
<i:EventTrigger EventName="SelectedItemChanged">
<i:InvokeCommandAction
Command="{Binding SetSelectedNode}"
CommandParameter="{Binding SelectedItem, ElementName=Tree}"/>
</i:EventTrigger>
</i:Interaction.Triggers>
</TreeView>
<Grid Grid.Row="1" Height="25">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="4*"/>
<ColumnDefinition Width="2*"/>
<ColumnDefinition Width="2*"/>
</Grid.ColumnDefinitions>
<TextBox x:Name="NameTextBox" Grid.Column="0" VerticalAlignment="Center" FontFamily="Verdana"/>
<Button Grid.Column="1" Content="Add Node" Command="{Binding AddNode}" CommandParameter="{Binding Text, ElementName=NameTextBox}" Background="Green"/>
<Button Grid.Column="2" Content="Remove Node" Command="{Binding RemoveNode}" Background="Red"/>
</Grid>
</Grid>
</UserControl>
最后是TreeView模型
public class TreeViewmodel : ObservableObject
{
public ICommand SetSelectedNode { get; private set; }
public ICommand AddNode { get; private set; }
public ICommand RemoveNode { get; private set; }
public TreeViewmodel()
{
this.SetSelectedNode = new ParamaterizedDelegateCommand(
node =>
{
this.SelectedTreeNode = (TreeNodeBase)node;
});
this.AddNode = new ParamaterizedDelegateCommand(name => this.SelectedTreeNode.AddChildNode((string)name));
this.RemoveNode = new DelegateCommand(
() =>
{
if (selectedTreeNode.GetType() == typeof(TreeNode))
{
var parent = ((TreeNode)this.SelectedTreeNode).Parent;
parent.RemoveChildNode(this.SelectedTreeNode.Name);
this.SelectedTreeNode = parent;
}
});
var adam = new TreeViewRoot("Adam");
var steve = adam.AddChildNode("Steve");
steve.AddChildNode("Jack");
this.rootLevelNodes = new List<TreeViewRoot> { adam, new TreeViewRoot("Eve") };
}
private TreeNodeBase selectedTreeNode;
private readonly IList<TreeViewRoot> rootLevelNodes;
public IEnumerable<TreeViewRoot> RootLevelNodes
{
get
{
return this.rootLevelNodes;
}
}
public TreeNodeBase SelectedTreeNode
{
get
{
return this.selectedTreeNode;
}
set
{
this.selectedTreeNode = value;
this.OnPropertyChanged();
}
}
}
因此我知道在添加子元素時應該通知UI,因為我調試它時可以看到在兩種情況下都會調用ChildNodes屬性上的get訪問器,但UI上顯示的內容保持不變。
在過去,我已經解決了這個問題,但是使用了ObservableCollections,這似乎就是這種問題的大多數解決方案都指向了StackOverflow,但為什么這個解決方案也不起作用呢? 我錯過了什么?
問題是你誤用了INotifyPropertyChanged
。 在您的代碼中,您通知視圖您的屬性ChildNodes
更改,但它不是真的,因為TreeViewItem.ItemsSource
仍然等於您的ChildNodes
屬性。
當您查看模型更改中的基礎集合對象時, INotifyPropertyChanged
將導致UI更新。
要在集合中出現新項目時更新ItemsSource
,您需要使用實現INotifyCollectionChanged
的集合。
正如MSDN所說 :
您可以枚舉實現IEnumerable接口的任何集合。 但是,要設置動態綁定以便集合中的插入或刪除自動更新UI,集合必須實現INotifyCollectionChanged接口。 此接口公開了一個事件,只要底層集合發生更改,就應該引發該事件。
這就是為什么SO上的每個人都建議使用ObservableCollection
。
編輯:
如果要公開只讀集合,則應檢查ReadOnlyObservableCollection<T>
Class 。 它作為ObservableCollection
的包裝器,可以非公開。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.