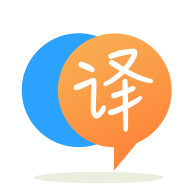
[英]Getting portfolio names and existing orders using Interactive Brokers IBPy
[英]ibpy Getting portfolio information: Interactive Broker, Python
我已經成功編寫了代碼,使用代碼從TWS演示版中提取有關我的職位的信息:
tws_conn = conn.Connection.create(port=7497, clientId=100)
tws_conn.register( acct_update, msg.updateAccountValue,
msg.updateAccountTime, msg.updatePortfolio)
tws_conn.connect()
tws_conn.reqPositions()
tws_conn.reqAccountUpdates(True,'DU15181')
但是,它將信息轉儲為:
<updatePortfolio contract=<Packages.IbPy.ib.ext.Contract.Contract object at 0x06B0FE30>, position=-10, marketPrice=3.4000001, marketValue=-3400.0, averageCost=334.345, unrealizedPNL=-56.55, realizedPNL=0.0, accountName=DU15181>
我想知道如何將上述信息存儲到一個數組中,該數組中包含合同或股票行情記錄,投資組合中的數量和購買價格的不同列
給定長度和主要更改,添加另一個答案。 鑒於我正在編寫某些代碼,因此我用它回答了另一個問題,並且稍作改動也可以用於產品組合。
碼:
from __future__ import (absolute_import, division, print_function,)
# unicode_literals)
import collections
import sys
if sys.version_info.major == 2:
import Queue as queue
import itertools
map = itertools.imap
else: # >= 3
import queue
import ib.opt
import ib.ext.Contract
class IbManager(object):
def __init__(self, timeout=20, **kwargs):
self.q = queue.Queue()
self.timeout = 20
self.con = ib.opt.ibConnection(**kwargs)
self.con.registerAll(self.watcher)
self.msgs = {
ib.opt.message.error: self.errors,
ib.opt.message.updatePortfolio: self.acct_update,
ib.opt.message.accountDownloadEnd: self.acct_update,
}
# Skip the registered ones plus noisy ones from acctUpdate
self.skipmsgs = tuple(self.msgs.keys()) + (
ib.opt.message.updateAccountValue,
ib.opt.message.updateAccountTime)
for msgtype, handler in self.msgs.items():
self.con.register(handler, msgtype)
self.con.connect()
def watcher(self, msg):
if isinstance(msg, ib.opt.message.error):
if msg.errorCode > 2000: # informative message
print('-' * 10, msg)
elif not isinstance(msg, self.skipmsgs):
print('-' * 10, msg)
def errors(self, msg):
if msg.id is None: # something is very wrong in the connection to tws
self.q.put((True, -1, 'Lost Connection to TWS'))
elif msg.errorCode < 1000:
self.q.put((True, msg.errorCode, msg.errorMsg))
def acct_update(self, msg):
self.q.put((False, -1, msg))
def get_account_update(self):
self.con.reqAccountUpdates(True, 'D999999')
portfolio = list()
while True:
try:
err, mid, msg = self.q.get(block=True, timeout=self.timeout)
except queue.Empty:
err, mid, msg = True, -1, "Timeout receiving information"
break
if isinstance(msg, ib.opt.message.accountDownloadEnd):
break
if isinstance(msg, ib.opt.message.updatePortfolio):
c = msg.contract
ticker = '%s-%s-%s' % (c.m_symbol, c.m_secType, c.m_exchange)
entry = collections.OrderedDict(msg.items())
# Don't do this if contract object needs to be referenced later
entry['contract'] = ticker # replace object with the ticker
portfolio.append(entry)
# return list of contract details, followed by:
# last return code (False means no error / True Error)
# last error code or None if no error
# last error message or None if no error
# last error message
return portfolio, err, mid, msg
ibm = IbManager(clientId=5001)
portfolio, err, errid, errmsg = ibm.get_account_update()
if portfolio:
print(','.join(portfolio[0].keys()))
for p in portfolio:
print(','.join(map(str, p.values())))
sys.exit(0) # Ensure ib thread is terminated
結果
Server Version: 76
TWS Time at connection:20160113 00:15:29 CET
---------- <managedAccounts accountsList=D999999>
---------- <nextValidId orderId=1>
---------- <error id=-1, errorCode=2104, errorMsg=Market data farm connection is OK:usfuture>
---------- <error id=-1, errorCode=2104, errorMsg=Market data farm connection is OK:eufarm>
---------- <error id=-1, errorCode=2104, errorMsg=Market data farm connection is OK:cashfarm>
---------- <error id=-1, errorCode=2104, errorMsg=Market data farm connection is OK:usfarm.us>
---------- <error id=-1, errorCode=2106, errorMsg=HMDS data farm connection is OK:ushmds.us>
---------- <error id=-1, errorCode=2106, errorMsg=HMDS data farm connection is OK:ilhmds>
---------- <error id=-1, errorCode=2106, errorMsg=HMDS data farm connection is OK:cashhmds>
---------- <error id=-1, errorCode=2106, errorMsg=HMDS data farm connection is OK:ethmds>
contract,position,marketPrice,marketValue,averageCost,unrealizedPNL,realizedPNL,accountName
IBM-STK-,10,25.0,250.0,210.0,40.0,0.0,D999999
最后兩行可以直接導入到(例如)Excel。 或者給定字典列表(要打印的內容),可以在腳本中對其進行進一步操作。
如果列表中的每個條目都是列表本身(並且子列表中的每個索引位置始終包含相同的字段),則鑒於列表已經滿足該要求,因此“列”的要求有些模糊
您收到的消息將發送到您在tws中注冊的回調。 每個“轉儲”字段都可以使用“點”符號或類似dict的方法(例如“鍵”,“值”和“項目”)進行訪問
主要挑戰是合同:IB允許訪問大量交易所和不同的交易資產。 “合同”對象(以及IB后端中的信息)似乎反映了對所有內容的統一/統一訪問的努力,同時表明他們必須在現有基礎架構上構建。
您提到“股票行情自動收錄器”,所以我想您可能會對以下內容感到滿意:具有“ ib”行業標准符號的IBM-STK-SMART(第2字段表示是股票,第3字段表示IB將使用SMART路由用於訂單和價格更新)
讓我們去看一個列表列表:
def acct_update(self, msg):
# Assume the function is a method in a class with access to a member
# 'portfolio' which is a list
if isinstance(msg, ib.opt.message.updatePortfolio):
c = msg.contract
ticker = '%s-%s-%s' % (contract.m_symbol, c.m_secType, c.m_exchange)
entry = [ticker]
entry.extend(msg.values)
self.portfolio.append(entry)
不幸的是, ibpy
消息中的“ keys”方法不是classmethod
,但是名稱實際上是__slots__
。 在持有acct_update
方法的類中,您可以執行以下操作:
class MyClass(object):
portfields = ['ticker'] + ib.opt.message.updatePortfolio.__slots__
如果您更喜歡ibpy已經提供的字段名稱,而不是按索引訪問列表中的字段,則還可以對dict
def __init__(self, ...)
self.portfolio = collections.OrderedDict()
def acct_update(self, msg):
# Assume the function is a method in a class with access to a member
# 'portfolio' which is a list
if isinstance(msg, ib.opt.message.updatePortfolio):
c = msg.contract
ticker = '%s-%s-%s' % (contract.m_symbol, c.m_secType, c.m_exchange)
self.portfolio[ticker] = collections.OrderedDict(msg.items())
這樣您就可以按名稱獲取最新的股票行情資訊,並按名稱訪問字段。
如果您需要保留每個股票行情記錄
def __init__(self, ...)
self.portfolio = collections.defaultdict(list)
def acct_update(self, msg):
# Assume the function is a method in a class with access to a member
# 'portfolio' which is a list
if isinstance(msg, ib.opt.message.updatePortfolio):
c = msg.contract
ticker = '%s-%s-%s' % (contract.m_symbol, c.m_secType, c.m_exchange)
self.portfolio[ticker].extend(msg.values())
您可以存儲“項目”而不是值,然后在需要時訪問元組。
除了使用ibpy,我還將導入IBWrapper,可以從Github下載該文件: https : //github.com/anthonyng2/ib
import pandas as pd
import numpy as np
import time
from IBWrapper import IBWrapper, contract
from ib.ext.EClientSocket import EClientSocket
accountName = "Your Account ID"
callback = IBWrapper() # Instantiate IBWrapper. callback
tws = EClientSocket(callback) # Instantiate EClientSocket and return data to callback
host = ""
port = 4002 # It is for default port no. in demo account
clientId = 25
tws.eConnect(host, port, clientId) # connect to TWS
create = contract() # Instantiate contract class
callback.initiate_variables()
tws.reqAccountUpdates(1, accountName)
time.sleep(2)
它們是您更新后的帳戶價值和投資組合摘要:
accvalue = pd.DataFrame(callback.update_AccountValue, columns = ['key', 'value', 'currency', 'accountName']) #[:199]
portfolio = pd.DataFrame(callback.update_Portfolio, columns=['Contract ID','Currency', 'Expiry','Include Expired','Local Symbol','Multiplier','Primary Exchange','Right',
'Security Type','Strike','Symbol','Trading Class','Position','Market Price','Market Value',
'Average Cost', 'Unrealised PnL', 'Realised PnL', 'Account Name'])
callback.update_AccountTime
print("AccountValue: \n" + str(accvalue))
print("portfolio: \n" + str(portfolio))
這是您更新的職位摘要:
# Position Summary
tws.reqPositions()
time.sleep(2)
dat = pd.DataFrame(callback.update_Position,
columns=['Account','Contract ID','Currency','Exchange','Expiry',
'Include Expired','Local Symbol','Multiplier','Right',
'Security Type','Strike','Symbol','Trading Class',
'Position','Average Cost'])
dat[dat["Account"] == accountName]
print("Position Summary: \n" + str(dat))
我編寫了以下代碼,使我可以直接從Interactive Brokers API中讀取頭寸和資產凈值。
# Interactive Brokers functions to import data
def read_positions(): #read all accounts positions and return DataFrame with information
from ibapi.client import EClient
from ibapi.wrapper import EWrapper
from ibapi.common import TickerId
import pandas as pd
class ib_class(EWrapper, EClient):
def __init__(self):
EClient.__init__(self, self)
self.all_positions = pd.DataFrame([], columns = ['Account','Symbol', 'Quantity', 'Average Cost'])
def position(self, account, contract, pos, avgCost):
index = str(account)+str(contract.symbol)
self.all_positions.loc[index]=account,contract.symbol,pos,avgCost
def error(self, reqId:TickerId, errorCode:int, errorString:str):
if reqId > -1:
print("Error. Id: " , reqId, " Code: " , errorCode , " Msg: " , errorString)
def positionEnd(self):
super().positionEnd()
self.disconnect()
ib_api = ib_class()
ib_api.connect("127.0.0.1", 7496, 0)
ib_api.reqPositions()
current_positions = ib_api.all_positions
ib_api.run()
return(current_positions)
def read_navs(): #read all accounts NAVs
from ibapi.client import EClient
from ibapi.wrapper import EWrapper
from ibapi.common import TickerId
import pandas as pd
class ib_class(EWrapper, EClient):
def __init__(self):
EClient.__init__(self, self)
self.all_accounts = pd.DataFrame([], columns = ['reqId','Account', 'Tag', 'Value' , 'Currency'])
def accountSummary(self, reqId, account, tag, value, currency):
if tag == 'NetLiquidationByCurrency':
index = str(account)
self.all_accounts.loc[index]=reqId, account, tag, value, currency
def error(self, reqId:TickerId, errorCode:int, errorString:str):
if reqId > -1:
print("Error. Id: " , reqId, " Code: " , errorCode , " Msg: " , errorString)
def accountSummaryEnd(self, reqId:int):
super().accountSummaryEnd(reqId)
self.disconnect()
ib_api = ib_class()
ib_api.connect("127.0.0.1", 7496, 0)
ib_api.reqAccountSummary(9001,"All","$LEDGER")
current_nav = ib_api.all_accounts
ib_api.run()
return(current_nav)
為了測試代碼,我將其保存在名為IB_API的.py文件中,然后運行以下命令:
import IB_API
print("Testing IB's API as an imported library:")
all_positions = IB_API.read_positions()
all_navs = IB_API.read_navs()
print("Test ended")
我仍在嘗試避免顯示錯誤“ [WinError 10038]”,該錯誤為“試圖對非套接字的對象進行操作”-請參閱關於此主題的問題
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.