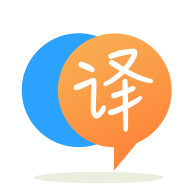
[英]How can I use an int instead of a Guid for the id column in IdentityUser class?
[英]How can I change an int ID column to Guid with EF migration?
我正在使用 EF 代碼優先方法並希望將Id
字段更改為guid
但似乎無法通過以下錯誤。
這是我的第一次遷移:
public partial class CreateDownloadToken : DbMigration
{
public override void Up()
{
CreateTable(
"dbo.DownloadTokens",
c => new
{
Id = c.Int(nullable: false, identity: true),
FileId = c.Int(),
UserId = c.String(nullable: false, maxLength: 128),
ValidUntil = c.DateTime(nullable: false),
})
.PrimaryKey(t => t.Id)
.ForeignKey("dbo.Files", t => t.FileId)
.ForeignKey("dbo.Users", t => t.UserId, cascadeDelete: true)
.Index(t => t.FileId)
.Index(t => t.UserId);
}
public override void Down()
{
DropForeignKey("dbo.DownloadTokens", "UserId", "dbo.Users");
DropForeignKey("dbo.DownloadTokens", "FileId", "dbo.Files");
DropIndex("dbo.DownloadTokens", new[] { "UserId" });
DropIndex("dbo.DownloadTokens", new[] { "FileId" });
DropTable("dbo.DownloadTokens");
}
}
后來我意識到我需要我的Id
列是 GUID,所以我更改了我的模型文件:
public class DownloadToken
{
[Key, DatabaseGenerated(DatabaseGeneratedOption.Computed)]
public Guid Id { get; set; }
public int? FileId { get; set; }
[ForeignKey("FileId")]
public virtual File File { get; set; }
[Required]
public string UserId { get; set; }
[ForeignKey("UserId")]
public virtual User User { get; set; }
[Required]
public DateTime ValidUntil { get; set; }
}
運行Add-Migration ChangeDownloadTokenIdToGuid
時,它會生成此文件:
public partial class ChangeDownloadTokenIdToGuid : DbMigration
{
public override void Up()
{
DropPrimaryKey("dbo.DownloadTokens");
AlterColumn("dbo.DownloadTokens", "Id", c => c.Guid(nullable: false));
AddPrimaryKey("dbo.DownloadTokens", "Id");
}
public override void Down()
{
DropPrimaryKey("dbo.DownloadTokens");
AlterColumn("dbo.DownloadTokens", "Id", c => c.Int(nullable: false, identity: true));
AddPrimaryKey("dbo.DownloadTokens", "Id");
}
}
使用Update-Database
運行此文件會導致此錯誤:
Identity column 'Id' must be of data type int, bigint, smallint, tinyint, or decimal or numeric with a scale of 0, and constrained to be nonnullable.
任何想法為什么會發生這種情況?
這是因為無法將以前的Id
列的int
類型轉換為Guid
類型(正是嘗試執行AlterColumn
方法)。 此外,錯誤消息建議您,新類型的Id
列可以是集合中的一種類型: int、bigint、smallint、tinyint 或 decimal 或 scale 為 0 的數字,對於它們來說,可以從int
執行轉換類型。
解決方案- 只需刪除Id
列,然后使用新的Guid
類型重新創建它,以這種方式更改遷移:
public partial class ChangeDownloadTokenIdToGuid : DbMigration
{
public override void Up()
{
DropPrimaryKey("dbo.DownloadTokens");
DropColumn("dbo.DownloadTokens", "Id");
AddColumn("dbo.DownloadTokens", "Id", c => c.Guid(nullable: false, identity: true));
AddPrimaryKey("dbo.DownloadTokens", "Id");
}
public override void Down()
{
DropPrimaryKey("dbo.DownloadTokens");
DropColumn("dbo.DownloadTokens", "Id");
AddColumn("dbo.DownloadTokens", "Id", c => c.Int(nullable: false, identity: true));
AddPrimaryKey("dbo.DownloadTokens", "Id");
}
}
PS 為什么你使用
DatabaseGeneratedOption.Computed
屬性,而不是DatabaseGeneratedOption.Identity
?
即使 Slava Utesinov 的作品,它只適用於空表或在沒有其他表引用您正在轉換的表的情況下。 所以這個答案將幫助那些最終在這個頁面上進行更復雜的數據庫設置的人。
下面是一個可以從遷移類中使用的實用程序函數,它應該從 Up/Down 函數中調用。 該函數還處理表引用您嘗試從 Int 轉換為 Guid 的表。 此輔助函數假定您要轉換的列稱為“Id”,但在其他方面應該是相當通用的。
public void Convert(bool toGuid, string parent, params string[] children)
{
if (toGuid)
{
AddColumn($"dbo.{parent}s", "Id2", c => c.Guid(nullable: false, identity: true, defaultValueSql: "newid()"));
}
else
{
AddColumn($"dbo.{parent}s", "Id2", c => c.Int(nullable: false, identity: true));
}
foreach (var child in children)
{
DropForeignKey($"dbo.{child}s", $"{parent}_Id", $"dbo.{parent}s");
DropIndex($"dbo.{child}s", new[] { $"{parent}_Id" });
RenameColumn($"dbo.{child}s", $"{parent}_Id", $"old_{parent}_Id");
if (toGuid)
{
AddColumn($"dbo.{child}s", $"{parent}_Id", c => c.Guid());
}
else
{
AddColumn($"dbo.{child}s", $"{parent}_Id", c => c.Int());
}
Sql($"update c set {parent}_Id=p.Id2 from {child}s c inner join {parent}s p on p.Id=c.old_{parent}_Id");
DropColumn($"dbo.{child}s", $"old_{parent}_Id");
}
DropPrimaryKey($"dbo.{parent}s");
DropColumn($"dbo.{parent}s", "Id");
RenameColumn($"dbo.{parent}s", "Id2", "Id");
AddPrimaryKey($"dbo.{parent}s", "Id");
foreach (var child in children)
{
CreateIndex($"dbo.{child}s", $"{parent}_Id");
AddForeignKey($"dbo.{child}s", $"{parent}_Id", $"dbo.{parent}s", "Id");
}
}
因此,在您的情況下,您的 Up/Down 功能將是:
public override void Up()
{
Convert(true,"DownloadToken");
}
public override void Down()
{
Convert(false, "DownloadToken");
}
我知道這是 EF,但對於像我這樣在 2021 年使用 EF Core 絆倒的人來說,這是我為改編 Andreas Willadsen 的代碼所做的:
protected override void Up(MigrationBuilder migrationBuilder)
{
migrationBuilder.AddColumn<Guid>("Id2", "{Parent}s", nullable: false);
migrationBuilder.DropForeignKey("FK_{Child}s_{Parent}s_{Parent}Id", "{Child}s");
migrationBuilder.DropIndex("IX_{Child}s_{Parent}Id", "{Child}s");
//if FK reference exists in child you can wipe the child table or..... instead of drop + add column below, need to call renamecolumn, then addcolumn, then use Andreas' post with migrationBuilder.SQL() and update to convert old keys to guid, then finally drop column.
migrationBuilder.DropColumn("{Parent}Id", "{Child}s");
migrationBuilder.AddColumn<Guid>("{Parent}Id", "{Child}s", nullable: false);
//
migrationBuilder.DropPrimaryKey("PK_{Parent}s", "{Parent}s");
migrationBuilder.DropColumn("Id", "{Parent}s");
migrationBuilder.RenameColumn("Id2", "{Parent}s", "Id");
migrationBuilder.AddPrimaryKey("PK_{Parent}s", "{Parent}s", column: "Id");
migrationBuilder.CreateIndex("IX_{Child}s_{Parent}Id", "{Child}s","{Parent}Id");
migrationBuilder.AddForeignKey(
name: "FK_{Child}s_{Parent}s_{Parent}Id",
table: "{Child}s",
column: "{Parent}Id",
principalTable: "{Parent}s",
principalColumn: "Id",
onDelete: ReferentialAction.Cascade);
}
將 {Parent} 替換為您的父表名稱,將 {Child} 替換為您的子表名稱,並在 Down() 中調用相同的內容,除了將 <Guid> 替換為 <int>。
我想知道為什么 ef core 還沒有在添加遷移中實現自動化。
這是我在一對多關系中用於完整表的。 newid 僅適用於 sql server 和 Azure。 羽絨不適用於此方法。
public void ConvertIntIdToGuidId (string tableName, MigrationBuilder migrationBuilder)
{
migrationBuilder.AddColumn<Guid>("Id2", $"{tableName}", nullable: true, defaultValue: new Guid("00000000-0000-0000-0000-000000000000"));
migrationBuilder.DropPrimaryKey($"PK_{tableName}", $"{tableName}");
migrationBuilder.DropColumn("Id", $"{tableName}");
migrationBuilder.RenameColumn("Id2", $"{tableName}", "Id");
migrationBuilder.Sql(
@$"UPDATE {tableName} SET Id = NEWID () ;");
migrationBuilder.AlterColumn<Guid>(name: "Id",table: $"{tableName}", nullable: false, oldNullable: true);
migrationBuilder.AddPrimaryKey($"PK_{tableName}", $"{tableName}", column: "Id");
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.