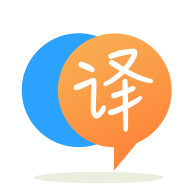
[英]Openpyxl - How to read only one column from Excel file in Python?
[英]How to use Python to read one column from Excel file?
我想在excel中讀取一列中的數據,這是我的代碼:
import xlrd
file_location = "location/file_name.xlsx"
workbook = xlrd.open_workbook(file_location)
sheet = workbook.sheet_by_name('sheet')
x = []
for cell in sheet.col[9]:
if isinstance(cell, float):
x.append(cell)
print(x)
這是錯誤的,因為工作表中沒有名為col[col.num]的方法,但我只想從第8列(H列)中提取數據,我該怎么辦?
如果你沒有被 xlrd 鎖定,我可能會使用 Pandas,這在處理來自任何地方的數據時非常好:
import pandas as pd
df = pd.ExcelFile('location/test.xlsx').parse('Sheet1') #you could add index_col=0 if there's an index
x=[]
x.append(df['name_of_col'])
然后,您可以使用 pandas df.to_excel()
將新提取的列寫入新的 excel 文件
您可以像這樣獲取第 8 列的值:
for rownum in range(sheet.nrows):
x.append(sheet.cell(rownum, 7))
到目前為止,使用xlrd
獲取列中所有值的最簡單方法是col_values()
工作表方法:
x = []
for value in sheet.col_values(8):
if isinstance(value, float):
x.append(value)
(請注意,如果您想要 H 列,則應使用 7,因為索引從 0 開始。)
順便說一句,您可以使用col()
獲取列中的單元格對象:
x = []
for cell in sheet.col(8):
if isinstance(cell.value, float):
x.append(cell.value)
找到這些東西的最佳位置是官方教程(它是xlrd
、 xlwt
和xlutils
的不錯參考)。 您當然也可以查看文檔和源代碼。
我建議這樣做:
import openpyxl
fname = 'file.xlsx'
wb = openpyxl.load_workbook(fname)
sheet = wb.get_sheet_by_name('sheet-name')
for rowOfCellObjects in sheet['C5':'C7']:
for cellObj in rowOfCellObjects:
print(cellObj.coordinate, cellObj.value)
結果:
C5 70.82
C6 84.82
C7 96.82
注意: fname指的是 excel 文件, get_sheet_by_name('sheet-name')指的是所需的工作表,並且在sheet['C5':'C7']中提到了列的范圍。
查看鏈接了解更多詳情。 代碼段也取自這里。
XLRD 很好,但對於這種情況,您可能會發現 Pandas 很好,因為它具有使用運算符“[]”選擇列的例程
您的上下文的完整工作代碼將是
import pandas as pd
file_location = "file_name.xlsx"
sheet = pd.read_excel(file_location)
print(x['column name of col 9'])
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.