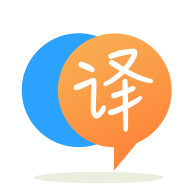
[英]How to give the permissions to sql server 2008 database to insert the data from client system in c# windows application?
[英]How to insert records from data gridview entry to SQL database in C# windows application?
我正在嘗試將記錄插入 sql 數據庫,下面是我通過單擊按鈕插入的代碼。
我無法插入記錄,並且在我執行代碼時一直在拋出錯誤.....我知道代碼中有問題,但我不確定問題出在哪里.... .
錯誤消息是“','附近的語法不正確..”
private void ADD_button_Click(object sender, EventArgs e)
{
try
{
using (SqlConnection con = new SqlConnection(sqlconn))
{
con.Open();
for (int i = 1; i < dataGridView.Rows.Count; i++)
{
string sql = @"INSERT INTO ERSBusinessLogic VALUES ("
+ dataGridView.Rows[i].Cells["ERSBusinessLogic_ID"].Value + ", "
+ dataGridView.Rows[i].Cells["ERSBusinessLogic_Formula"].Value + ", "
+ dataGridView.Rows[i].Cells["ERSBusinessLogic_InputsCount"].Value + ", "
+ dataGridView.Rows[i].Cells["ERSBusinessLogic_Inputs"].Value + ");";
SqlCommand cmd = new SqlCommand(sql, con);
cmd.ExecuteNonQuery();
}
}
}
catch (Exception ex)
{
MessageBox.Show("Error : " + ex.Message);
}
finally
{
con.Close();
}
試試這個
private void button1_Click(object sender, EventArgs e)
{
// Getting data from DataGridView
DataTable myDt = new DataTable();
myDt = GetDTfromDGV(dataGridView1);
// Writing to sql
WriteToSQL(myDt);
}
private DataTable GetDTfromDGV(DataGridView dgv)
{
// Macking our DataTable
DataTable dt = new DataTable();
foreach (DataGridViewColumn column in dgv.Columns)
{
dt.Columns.Add(column.Name, typeof(string));
}
// Getting data
foreach (DataGridViewRow dgvRow in dgv.Rows)
{
DataRow dr = dt.NewRow();
for (int col = 0; col < dgv.Columns.Count; col++)
{
dr[col] = dgvRow.Cells[col].Value;
}
dt.Rows.Add(dr);
}
// removing empty rows
for (int row = dt.Rows.Count - 1; row >= 0; row--)
{
bool flag = true;
for (int col = 0; col < dt.Columns.Count; col++)
{
if (dt.Rows[row][col] != DBNull.Value)
{
flag = false;
break;
}
}
if (flag == true)
{
dt.Rows.RemoveAt(row);
}
}
return dt;
}
private void WriteToSQL(DataTable dt)
{
string connectionStringSQL = "Your connection string";
using (SqlConnection sqlConn = new SqlConnection(connectionStringSQL))
{
SqlBulkCopy sqlBulkCopy = new SqlBulkCopy(sqlConn);
// Setting the database table name
sqlBulkCopy.DestinationTableName = "Table_1_temp";
// Mapping the DataTable columns with that of the database table
sqlBulkCopy.ColumnMappings.Add(dt.Columns[0].ColumnName, "sql_col1");
sqlBulkCopy.ColumnMappings.Add(dt.Columns[1].ColumnName, "sql_col2");
sqlBulkCopy.ColumnMappings.Add(dt.Columns[2].ColumnName, "sql_col3");
sqlBulkCopy.ColumnMappings.Add(dt.Columns[3].ColumnName, "sql_col4");
sqlBulkCopy.ColumnMappings.Add(dt.Columns[4].ColumnName, "sql_col5");
sqlBulkCopy.ColumnMappings.Add(dt.Columns[5].ColumnName, "sql_col6");
sqlConn.Open();
sqlBulkCopy.WriteToServer(dt);
}
}
這似乎是一個數據類型不匹配問題。 如果表列是數字類型(例如:ERSBusinessLogic_ID 是整數),請檢查您的數據表,然后代碼應類似於“+Convert.ToInt32(dataGridView.Rows[i].Cells["ERSBusinessLogic_ID"].Value) + ",
如果它是 var-char 類型,那么值應該是單引號 ('') 像 '"+Convert.ToString(dataGridView.Rows[i].Cells["ERSBusinessLogic_Formula"].Value) + "',
try
{
using (SqlConnection con = new SqlConnection(sqlconn))
{
using (SqlCommand cmd = new SqlCommand())
{
cmd.Connection = con;
con.Open();
for (int i = 1; i < dataGridView.Rows.Count; i++)
{
string sql = @"INSERT INTO ERSBusinessLogic VALUES ("
+dataGridView.Rows[i].Cells["ERSBusinessLogic_ID"].Value + ", "
+ dataGridView.Rows[i].Cells["ERSBusinessLogic_Formula"].Value + ", "
+ dataGridView.Rows[i].Cells["ERSBusinessLogic_InputsCount"].Value + ", "
+ dataGridView.Rows[i].Cells["ERSBusinessLogic_Inputs"].Value + ");";
cmd.CommandText = sql;
cmd.ExecuteNonQuery();
}
}
}
catch (Exception ex)
{
MessageBox.Show("Error : " + ex.Message);
}
finally
{
con.Close();
}
如果仍然不起作用,請在字符串后面放置一個斷點,然后復制字符串值並在您的數據庫上對其進行測試。 也許你的價值觀是問題,不同類型。
嘗試將 '' 添加到每個數據列。 如下,
for (int i = 1; i < dataGridView.Rows.Count; i++)
{
string sql = @"INSERT INTO ERSBusinessLogic VALUES ('"
+ dataGridView.Rows[i].Cells["ERSBusinessLogic_ID"].Value + "', '"
+ dataGridView.Rows[i].Cells["ERSBusinessLogic_Formula"].Value + "', '"
+ dataGridView.Rows[i].Cells["ERSBusinessLogic_InputsCount"].Value + "', '"
+ dataGridView.Rows[i].Cells["ERSBusinessLogic_Inputs"].Value + "');";
SqlCommand cmd = new SqlCommand(sql, con);
cmd.ExecuteNonQuery();
}
祝你有美好的一天。 您也可以嘗試執行此選項。 小心寫你的變量記錄。
using (SqlCommand cmd = new SqlCommand("INSERT INTO MyTable(Column1,Column2) VALUES (@Value1 @Value2)",con))
cmd.Parameters.Add(newSqlParameter("@Value1",SqlDbType.VarChar));
cmd.Parameters.Add(newSqlParameter("@Value2",SqlDbType.VarChar));
con.Open();
foreach (DataGridViewRow row in myDataGridView.Rows)
{
if (!row.IsNewRow)
{
cmd.Parameters["@C1"].Value = row.Cells[0].Value;
cmd.Parameters["@C2"].Value = row.Cells[1].Value;
cmd.ExecuteNonQuery();
}
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.