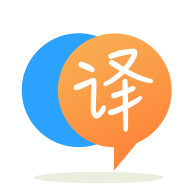
[英]Find index of all rows with null values in a particular column in pandas dataframe
[英]How to find rows with column values having a particular datatype in a Pandas DATAFRAME
我有一個數據框
name col1
satya 12
satya abc
satya 109.12
alex apple
alex 1000
所以現在我需要顯示其中'col1'列具有int值的行。
name col1
satya 12
alex 1000
如果搜索字符串值
name col1
satya abc
alex apple
一樣明智..請建議一些代碼行(可能正在使用reg)。
讓我們從一個簡單的正則表達式開始,如果您有一個整數,它將計算為True
,否則為False
:
import re
regexp = re.compile('^-?[0-9]+$')
bool(regexp.match('1000'))
True
bool(regexp.match('abc'))
False
一旦有了這樣的正則表達式,您可以按照以下步驟操作:
mask = df['col1'].map(lambda x: bool(regexp.match(x)) )
df.loc[mask]
name col1
0 satya 12
4 alex 1000
要搜索字符串,您將執行以下操作:
regexp_str = re.compile('^[a-zA-Z]+$')
mask_str = df['col1'].map(lambda x: bool(regexp_str.match(x)))
df.loc[mask_str]
name col1
1 satya abc
3 alex apple
編輯
如果通過以下方式創建數據框,則以上代碼將起作用:
df = pd.read_clipboard()
(或者,所有變量都作為字符串提供)。
regex方法是否有效取決於df
創建方式。 例如,如果它是通過以下方式創建的:
df = pd.DataFrame({'name': ['satya','satya','satya', 'alex', 'alex'],
'col1': [12,'abc',109.12,'apple',1000] },
columns=['name','col1'])
上面的代碼將因TypeError: expected string or bytes-like object
失敗TypeError: expected string or bytes-like object
為了使其在任何情況下都能正常工作,需要將類型強制轉換為str
:
mask = df['col1'].astype('str').map(lambda x: bool(regexp.match(x)) )
df.loc[mask]
name col1
0 satya 12
4 alex 1000
和字符串相同:
regexp_str = re.compile('^[a-zA-Z]+$')
mask_str = df['col1'].astype('str').map(lambda x: bool(regexp_str.match(x)))
df.loc[mask_str]
name col1
1 satya abc
3 alex apple
EDIT2
查找浮點數:
regexp_float = re.compile('^[-\+]?[0-9]*(\.[0-9]+)$')
mask_float = df['col1'].astype('str').map(lambda x: bool(regexp_float.match(x)))
df.loc[mask_float]
name col1
2 satya 109.12
在pandas
您將執行以下操作:
mask = df.col1.apply(lambda x: type(x) == int)
print df[mask]
這將產生您的預期輸出。
您可以檢查該值是否僅包含數字:
In [104]: df
Out[104]:
name col1
0 satya 12
1 satya abc
2 satya 109.12
3 alex apple
4 alex 1000
整數:
In [105]: df[~df.col1.str.contains(r'\D')]
Out[105]:
name col1
0 satya 12
4 alex 1000
非整數:
In [106]: df[df.col1.str.contains(r'\D')]
Out[106]:
name col1
1 satya abc
2 satya 109.12
3 alex apple
如果要過濾所有數值(整數/浮點數/小數),則可以使用pd.to_numeric(...,errors ='coerce') :
In [75]: df
Out[75]:
name col1
0 satya 12
1 satya abc
2 satya 109.12
3 alex apple
4 alex 1000
In [76]: df[pd.to_numeric(df.col1, errors='coerce').notnull()]
Out[76]:
name col1
0 satya 12
2 satya 109.12
4 alex 1000
In [77]: df[pd.to_numeric(df.col1, errors='coerce').isnull()]
Out[77]:
name col1
1 satya abc
3 alex apple
def is_integer(element):
try:
int(element) #if this is str then there will be error
return 1
except:
return 0
您可以簡單地定義如下函數,然后使用for循環列出您的項目。
def list_str(list_of_data):
str_list=[]
for item in list_of_data: #list_of_data = [[names],[col1s]] if just col1s replace item[2] with item[1]
if not is_integer(item[2]):
str_list.append(item)
return str_list
def list_int(list_of_data):
int_list=[]
for item in list_of_data:
if is_integer(item[2]):
int_list.append(item)
return int_list
希望這可以幫到你
您可以使用df.applymap(np.isreal)
df = pd.DataFrame({'col1': [12,'abc',109.12,'apple',1000], 'name': ['satya','satya','satya', 'alex', 'alex']})
df
col1 name
0 12 satya
1 abc satya
2 109.12 satya
3 apple alex
4 1000 alex
df2 = df[df.applymap(np.isreal)]
df2
col1 name
0 12 NaN
1 NaN NaN
2 109.12 NaN
3 NaN NaN
4 1000 NaN
df2 = df2[df2.col1.notnull()]
df2
col1 name
0 12 NaN
2 109.12 NaN
4 1000 NaN
index_list = df2.index.tolist()
index_list
[0, 2, 4]
df = df.iloc[index_list]
df
col1 name
0 12 satya
2 109.12 satya
4 1000 alex
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.