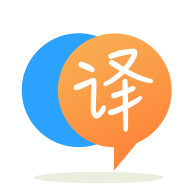
[英]How to add left diagonal of a 2D square matrix using user-defined functions in C++
[英]How to calculate the true square root of a number with 3 user-defined functions
我需要一些幫助和一些關於去哪里的提示 對於編程作業,我必須編寫一個程序來計算用戶輸入的數字的平方根,並且有某些要求。
主要要求輸入數字並顯示它,在循環內運行,以便用戶可以重復該程序而無需關閉它
計算必須在名為sqRoot的函數中完成,該函數將由main使用以下算法調用:
newValue = 0.5 * (oldValue + (X / oldValue))
我什至不知道從哪里開始這樣的程序。 但是,這是我到目前為止所擁有的:
#include <iostream>
#include <cmath>
#include <cstdlib>
using namespace std;
double sqRoot();
double absVal();
int i = 0;
double X;
int main()
{
sqRoot = sqrt(X);
double X;
// Calculations
cout << "Please enter a number: ";
cin >> X;
while (X <= 0)
{
cout << "*** Error: Invalid Number! *** " << endl;
cout << "Please enter a number: ";
cin >> X;
}
while (X >= 1)
{
cout << "The Square Root is: " << sqRoot << endl;
}
}
double sqRoot ()
{
double newValue;
double oldValue ;
while (abs(newValue - oldValue) > 0.0001)
{
newValue = 0.5 * (oldValue + ( X / oldValue));
oldValue = newValue;
cout << " The square root is: " << newValue << endl;
}
return (newValue);
}
我只是堅持下一步要做什么以及如何正確編寫程序。 感謝您的幫助和提示!
在您的代碼段中,您沒有展示如何實現absVal()
,這是微不足道的:
double absVal( double x )
{
return x < 0.0 ? -x : x;
}
假設您知道三元運算符。 否則使用if
。
您發布的main()
的實現基本上是一個無限循環,它僅重復計算和打印用戶輸入的第一個等於或大於 1.0 的數字。 這不是你被要求的,我想
我不確定 x >= 1 條件是否是強制性的(微小的值需要更多的迭代)或你的假設以及在負數的情況下你應該做什么(你可以使用 absVal 而不是打印錯誤) ,但你可以這樣寫:
#include <iostream>
// #include <cmath> <-- you are not supposed to use that
// #include <cstdlib> <-- why do you want that?
// using namespace std; <-- bad idea
using std::cin;
using std::cout;
double absVal( double x );
double sqRoot( double x );
int main()
{
double num;
cout << "This program calculate the square root of the numbers you enter.\n"
<< "Please, enter a number or something else to quit the program:\n";
// this will loop till std::cin fails
while ( cin >> num )
{
if ( num < 0.0 ) {
cout << "*** Error: Invalid input! ***\n"
<< "Please enter a positive number: ";
continue;
}
cout << "The square root of " << num << " is: " << sqRoot(num);
cout << "\nPlease enter a number: ";
}
return 0; // you missed this
}
然后,在您的sqRoot()
實現中,您忘記將變量 x 作為參數傳遞,以初始化 oldValue 和 newValue,如果執行流程碰巧進入 while 循環,它將在第一個循環后退出,因為oldValue = newValue;
在條件之前評估。 嘗試這樣的事情(我使用相對誤差而不是絕對差來獲得更小的 x 值的更好精度,但需要更多的迭代):
double sqRoot(double x)
{
const double eps = 0.0001;
double newValue = 0;
double oldValue = x;
while ( oldValue != 0.0 )
{
newValue = 0.5 * (oldValue + (x / oldValue));
// check if the relative error is small enough
if ( absVal(newValue - oldValue) / oldValue < eps )
break;
oldValue = newValue;
}
return newValue;
}
希望它有所幫助。
只是一些更正
#include <iostream>
#include <cmath>
#include <cstdlib>
using namespace std;
double sqRoot(double X);
int main()
{
double X;
// Calculations
cout << "Please enter a number: ";
cin >> X;
while (X <= 0)
{
cout << "*** Error: Invalid Number! *** " << endl;
cout << "Please enter a number: ";
cin >> X;
}
while (X >= 1)
{
cout << "The Square Root is: " << sqRoot(X) << endl;
}
}
double sqRoot(double X)
{
double newValue = 0;
double oldValue = X;
while (true)
{
newValue = 0.5 * (oldValue + (X / oldValue));
if (abs(newValue - oldValue) < 0.0001)
break;
oldValue = newValue;
//cout << " The square root is: " << newValue << endl;
}
return (newValue);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.