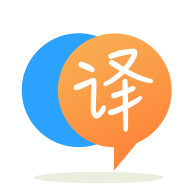
[英]inserting a dynamically generated $_POST values from a form into mysql from php
[英]PHP: Inserting Values from the Form into MySQL
我從終端在mysql
創建了一個users
表,我正在嘗試創建簡單的任務:從表單插入值。 這是我的dbConfig file
<?php
$mysqli = new mysqli("localhost", "root", "pass", "testDB");
/* check connection */
if (mysqli_connect_errno()) {
printf("Connect failed: %s\n", mysqli_connect_error());
exit();
}
?>
這是我的Index.php
。
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1">
<meta name="description" content="$1">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" type="text/css" href="style.css">
<title>test</title>
<?php
include_once 'dbConfig.php';
?>
</head>
<body>
<?php
if(isset($_POST['save'])){
$sql = "INSERT INTO users (username, password, email)
VALUES ('".$_POST["username"]."','".$_POST["password"]."','".$_POST["email"]."')";
}
?>
<form method="post">
<label id="first"> First name:</label><br/>
<input type="text" name="username"><br/>
<label id="first">Password</label><br/>
<input type="password" name="password"><br/>
<label id="first">Email</label><br/>
<input type="text" name="email"><br/>
<button type="submit" name="save">save</button>
<button type="submit" name="get">get</button>
</form>
</body>
</html>
點擊我的保存按鈕后,沒有任何反應,數據庫仍然是空的。 我嘗試echo'ing
顯INSERT
查詢,它按照預期從表單中獲取所有值。 在我嘗試從終端檢查這是否有效后,我登錄到我的sql
嘗試從用戶表中返回所有數據,但我得到了空集。
以下代碼僅聲明了一個包含 MySQL 查詢的字符串變量:
$sql = "INSERT INTO users (username, password, email)
VALUES ('".$_POST["username"]."','".$_POST["password"]."','".$_POST["email"]."')";
它不執行查詢。 為了做到這一點,你需要使用一些函數,但讓我先解釋一下其他的東西。
永遠不要信任用戶輸入:您永遠不應該將用戶輸入(例如來自$_GET
或$_POST
表單輸入)直接附加到您的查詢中。 有人可以以這種方式小心地操縱輸入,從而可能對您的數據庫造成很大的損害。 這就是所謂的 SQL 注入。 你可以在這里閱讀更多關於它的信息
為了保護您的腳本免受此類攻擊,您必須使用准備好的語句。 更多關於准備好的聲明在這里
在您的代碼中包含准備好的語句,如下所示:
$sql = "INSERT INTO users (username, password, email)
VALUES (?,?,?)";
注意如何?
用作值的占位符。 接下來,您應該使用mysqli_prepare
准備語句:
$stmt = $mysqli->prepare($sql);
然后開始將輸入變量綁定到准備好的語句:
$stmt->bind_param("sss", $_POST['username'], $_POST['email'], $_POST['password']);
最后執行准備好的語句。 (這是實際插入發生的地方)
$stmt->execute();
注意雖然不是問題的一部分,但我強烈建議您永遠不要以明文形式存儲密碼。 相反,您應該使用password_hash
來存儲password_hash
的哈希值
您的代碼中有兩個問題。
配置文件
<?php
$conn=mysqli_connect("localhost","root","password","testDB");
if(!$conn)
{
die("Connection failed: " . mysqli_connect_error());
}
?>
索引.php
include('dbConfig.php');
<!Doctype html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1">
<meta name="description" content="$1">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" type="text/css" href="style.css">
<title>test</title>
</head>
<body>
<?php
if(isset($_POST['save']))
{
$sql = "INSERT INTO users (username, password, email)
VALUES ('".$_POST["username"]."','".$_POST["password"]."','".$_POST["email"]."')";
$result = mysqli_query($conn,$sql);
}
?>
<form action="index.php" method="post">
<label id="first"> First name:</label><br/>
<input type="text" name="username"><br/>
<label id="first">Password</label><br/>
<input type="password" name="password"><br/>
<label id="first">Email</label><br/>
<input type="text" name="email"><br/>
<button type="submit" name="save">save</button>
</form>
</body>
</html>
嘗試這個:
dbConfig.php
<?php
$mysqli = new mysqli('localhost', 'root', 'pwd', 'yr db name');
if($mysqli->connect_error)
{
echo $mysqli->connect_error;
}
?>
Index.php
<html>
<head><title>Inserting data in database table </title>
</head>
<body>
<form action="control_table.php" method="post">
<table border="1" background="red" align="center">
<tr>
<td>Login Name</td>
<td><input type="text" name="txtname" /></td>
</tr>
<br>
<tr>
<td>Password</td>
<td><input type="text" name="txtpwd" /></td>
</tr>
<tr>
<td> </td>
<td><input type="submit" name="txtbutton" value="SUBMIT" /></td>
</tr>
</table>
control_table.php
<?php include 'config.php'; ?>
<?php
$name=$pwd="";
if(isset($_POST['txtbutton']))
{
$name = $_POST['txtname'];
$pwd = $_POST['txtpwd'];
$mysqli->query("insert into users(name,pwd) values('$name', '$pwd')");
if(!$mysqli)
{ echo mysqli_error(); }
else
{
echo "Successfully Inserted <br />";
echo "<a href='show.php'>View Result</a>";
}
}
?>
<!DOCTYPE html>
<?php
$con = new mysqli("localhost","root","","form");
?>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
<meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" />
<title>Untitled Document</title>
<script type="text/javascript">
$(document).ready(function(){
//$("form").submit(function(e){
$("#btn1").click(function(e){
e.preventDefault();
// alert('here');
$(".apnew").append('<input type="text" placeholder="Enter youy Name" name="e1[]"/><br>');
});
//}
});
</script>
</head>
<body>
<h2><b>Register Form<b></h2>
<form method="post" enctype="multipart/form-data">
<table>
<tr><td>Name:</td><td><input type="text" placeholder="Enter youy Name" name="e1[]"/>
<div class="apnew"></div><button id="btn1">Add</button></td></tr>
<tr><td>Image:</td><td><input type="file" name="e5[]" multiple="" accept="image/jpeg,image/gif,image/png,image/jpg"/></td></tr>
<tr><td>Address:</td><td><textarea cols="20" rows="4" name="e2"></textarea></td></tr>
<tr><td>Contact:</td><td><div id="textnew"><input type="number" maxlength="10" name="e3"/></div></td></tr>
<tr><td>Gender:</td><td><input type="radio" name="r1" value="Male" checked="checked"/>Male<input type="radio" name="r1" value="feale"/>Female</td></tr>
<tr><td><input id="submit" type="submit" name="t1" value="save" /></td></tr>
</table>
<?php
//echo '<pre>';print_r($_FILES);exit();
if(isset($_POST['t1']))
{
$values = implode(", ", $_POST['e1']);
$imgarryimp=array();
foreach($_FILES["e5"]["tmp_name"] as $key=>$val){
move_uploaded_file($_FILES["e5"]["tmp_name"][$key],"images/".$_FILES["e5"]["name"][$key]);
$fname = $_FILES['e5']['name'][$key];
$imgarryimp[]=$fname;
//echo $fname;
if(strlen($fname)>0)
{
$img = $fname;
}
$d="insert into form(name,address,contact,gender,image)values('$values','$_POST[e2]','$_POST[e3]','$_POST[r1]','$img')";
if($con->query($d)==TRUE)
{
echo "Yoy Data Save Successfully!!!";
}
}
exit;
// echo $values;exit;
//foreach($_POST['e1'] as $row)
//{
$d="insert into form(name,address,contact,gender,image)values('$values','$_POST[e2]','$_POST[e3]','$_POST[r1]','$img')";
if($con->query($d)==TRUE)
{
echo "Yoy Data Save Successfully!!!";
}
//}
//exit;
}
?>
</form>
<table>
<?php
$t="select * from form";
$y=$con->query($t);
foreach ($y as $q);
{
?>
<tr>
<td>Name:<?php echo $q['name'];?></td>
<td>Address:<?php echo $q['address'];?></td>
<td>Contact:<?php echo $q['contact'];?></td>
<td>Gender:<?php echo $q['gender'];?></td>
</tr>
<?php }?>
</table>
</body>
</html>
<?php
$username="root";
$password="";
$database="test";
#get the data from form fields
$Id=$_POST['Id'];
$P_name=$_POST['P_name'];
$address1=$_POST['address1'];
$address2=$_POST['address2'];
$email=$_POST['email'];
mysql_connect(localhost,$username,$password);
@mysql_select_db($database) or die("unable to select database");
if($_POST['insertrecord']=="insert"){
$query="insert into person values('$Id','$P_name','$address1','$address2','$email')";
echo "inside";
mysql_query($query);
$query1="select * from person";
$result=mysql_query($query1);
$num= mysql_numrows($result);
#echo"<b>output</b>";
print"<table border size=1 >
<tr><th>Id</th>
<th>P_name</th>
<th>address1</th>
<th>address2</th>
<th>email</th>
</tr>";
$i=0;
while($i<$num)
{
$Id=mysql_result($result,$i,"Id");
$P_name=mysql_result($result,$i,"P_name");
$address1=mysql_result($result,$i,"address1");
$address2=mysql_result($result,$i,"address2");
$email=mysql_result($result,$i,"email");
echo"<tr><td>$Id</td>
<td>$P_name</td>
<td>$address1</td>
<td>$address2</td>
<td>$email</td>
</tr>";
$i++;
}
print"</table>";
}
if($_POST['searchdata']=="Search")
{
$P_name=$_POST['name'];
$query="select * from person where P_name='$P_name'";
$result=mysql_query($query);
print"<table border size=1><tr><th>Id</th>
<th>P_name</th>
<th>address1</th>
<th>address2</th>
<th>email</th>
</tr>";
while($row=mysql_fetch_array($result))
{
$Id=$row[Id];
$P_name=$row[P_name];
$address1=$row[address1];
$address2=$row[address2];
$email=$row[email];
echo"<tr><td>$Id</td>
<td>$P_name</td>
<td>$address1</td>
<td>$address2</td>
<td>$email</td>
</tr>";
}
echo"</table>";
}
echo"<a href=lab2.html> Back </a>";
?>
當您單擊按鈕時
if(isset($_POST['save'])){
$sql = "INSERT INTO `members`(`id`, `membership_id`, `email`, `first_name`)
VALUES ('".$_POST["id"]."','".$_POST["membership_id"]."','".$_POST["email"]."','".$_POST["firstname"]."')";
**if ($conn->query($sql) === TRUE) {
echo "New record created successfully";
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}**
}
這將在變量$ sql中執行查詢
if ($conn->query($sql) === TRUE) {
echo "New record created successfully";
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.