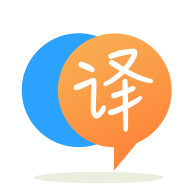
[英]How to get the size of Azure Blob Container without blob list iteration?
[英]How do you get the size of specific directory within a container in Azure Blob Storage?
我正在處理 Azure Blob 存儲實例中特定目錄中包含的大量數據,我只想獲取某個目錄中所有內容的大小。 我知道如何獲取整個容器的大小,但是它讓我逃避如何只指定目錄本身來從中提取數據。
我當前的代碼如下所示:
private static long GetSizeOfBlob(CloudStorageAccount storageAccount, string nameOfBlob)
{
CloudBlobClient blobClient = storageAccount.CreateCloudBlobClient();
// Finding the container
CloudBlobContainer container = blobClient.GetContainerReference(nameOfBlob);
// Iterating to get container size
long containerSize = 0;
foreach (var listBlobItem in container.ListBlobs(null, true))
{
var blobItem = listBlobItem as CloudBlockBlob;
containerSize += blobItem.Properties.Length;
}
return containerSize;
}
如果我將 blob 容器指定為“democontainer/testdirectory”之類的東西,我會得到一個 400 錯誤(我認為因為它是一個目錄,所以使用反斜杠可以讓我導航到我想要遍歷的目錄)。 任何幫助將不勝感激。
您需要先獲取CloudBlobDirectory
然后才能從那里開始工作。
例如:
var directories = new List<string>();
var folders = blobs.Where(b => b as CloudBlobDirectory != null);
foreach (var folder in folders)
{
directories.Add(folder.Uri);
}
我已經解決了我自己的問題,但留給未來的 azure blob 存儲瀏覽器。
您實際上需要在調用container.ListBlobs(prefix: 'somedir', true)
時提供前綴,以便僅訪問所涉及的特定目錄。 此目錄是您正在訪問的容器名稱之后的任何內容。
private string GetBlobContainerSize(CloudBlobContainer contSrc)
{
var blobfiles = new List<string>();
long blobfilesize = 0;
var blobblocks = new List<string>();
long blobblocksize = 0;
foreach (var g in contSrc.ListBlobs())
{
if (g.GetType() == typeof(CloudBlobDirectory))
{
foreach (var file in ((CloudBlobDirectory)g).ListBlobs(true).Where(x => x as CloudBlockBlob != null))
{
blobfilesize += (file as CloudBlockBlob).Properties.Length;
blobfiles.Add(file.Uri.AbsoluteUri);
}
}
else if (g.GetType() == typeof(CloudBlockBlob))
{
blobblocksize += (g as CloudBlockBlob).Properties.Length;
blobblocks.Add(g.Uri.AbsoluteUri);
}
}
string res = string.Empty;
if (blobblocksize > 0) res += "size: " + FormatSize(blobblocksize) + "; blocks: " + blobblocks.Count();
if (!string.IsNullOrEmpty(res) && blobfilesize > 0) res += "; ";
if (blobfilesize > 0) res += "size: " + FormatSize(blobfilesize) + "; files: " + blobfiles.Count();
return res;
}
private string FormatSize(long len)
{
string[] sizes = { "B", "KB", "MB", "GB", "TB" };
int order = 0;
while (len >= 1024 && order < sizes.Length - 1)
{
order++;
len = len/1024;
}
return $"{len:0.00} {sizes[order]}".PadLeft (9, ' ');
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.