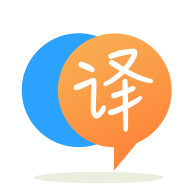
[英]Casting ObservableCollection<Object> to ObservableCollection<Type> Where type is unknown
[英]Casting an object as an ObservableCollection<object>
我正在嘗試編寫一種方法,該方法在viewModel中獲取所有ObservableCollections並將其強制轉換為ObservableCollection<object>
。 使用反射,我已經能夠將每個ObservableCollection<T>
作為一個對象,但是我很難將此對象轉換為ObservableCollection<object>
。 到目前為止,這是我的代碼:
var props = viewModel.GetType().GetProperties();
Type t = viewModel.GetType();
foreach (var prop in props)
{
if (prop.PropertyType.Name == "ObservableCollection`1")
{
Type type = prop.PropertyType;
var property = (t.GetProperty(prop.Name)).GetValue(viewModel);
// cast property as an ObservableCollection<object>
}
}
有人知道我應該怎么做嗎?
將類型名稱與字符串進行比較是一個壞主意。 為了斷言它是一個ObservableCollection
,可以使用以下命令:
if (prop.PropertyType.IsGenericType &&
prop.PropertyType.GetGenericTypeDefinition() == typeof(ObservableCollection<>))
該值可以這樣提取和轉換:
foreach (var prop in viewModel.GetType().GetProperties())
{
if (prop.PropertyType.IsGenericType &&
prop.PropertyType.GetGenericTypeDefinition() == typeof(ObservableCollection<>))
{
var values = (IEnumerable)prop.GetValue(viewModel);
// cast property as an ObservableCollection<object>
var collection = new ObservableCollection<object>(values.OfType<object>());
}
}
如果您希望將它們合並到一個集合中,則可以執行以下操作:
var values = viewModel.GetType().GetProperties()
.Where(p => p.PropertyType.IsGenericType)
.Where(p => p.PropertyType.GetGenericTypeDefinition() == typeof(ObservableCollection<>))
.Select(p => (IEnumerable)p.GetValue(viewModel))
.SelectMany(e => e.OfType<object>());
var collection = new ObservableCollection<object>(values);
這個問題的答案在這里: https : //stackoverflow.com/a/1198760/3179310
但是要明確您的情況:
if (prop.PropertyType.Name == "ObservableCollection`1")
{
Type type = prop.PropertyType;
var property = (t.GetProperty(prop.Name)).GetValue(viewModel);
// cast property as an ObservableCollection<object>
var col = new ObservalbeCollection<object>(property);
// if the example above fails you need to cast the property
// from 'object' to an ObservableCollection<T> and then execute the code above
// to make it clear:
var mecol = new ObservableCollection<object>();
ICollection obscol = (ICollection)property;
for(int i = 0; i < obscol.Count; i++)
{
mecol.Add((object)obscol[i]);
}
// the example above can throw some exceptions but it should work in most cases
}
您可以使用Cast<T>()
擴展方法,但不要忘記,使用此方法(如下)將創建一個新實例,因此原始事件無效。 如果您仍然想接收事件,則應圍繞它創建包裝器。
var prop = viewModel.GetType("ObservableCollection`1");
var type = prop.PropertyType;
var propertyValue = (t.GetProperty(prop.Name)).GetValue(viewModel);
// cast property as an ObservableCollection<object>
var myCollection = new ObservableCollection<object>(
((ICollection)propertyValue).Cast<object>());
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.