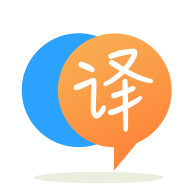
[英]Method that returns a new array where each element is the square or the original element
[英]New array for each N element
我有一個200~元素的數組,我試圖通過對每N個元素執行它來將該數組拆分成更小的數組,這意味着我不能使用.take / .skip命令,我目前嘗試使用不同的解決方案如:
一個Parallel.for和parallel.foreach(如果我能算出來那將是最好的)
和普通的for和foreach循環一樣,但是我現在所能做的就是這個靜態解決方案,我自己創建了一個新的組,在arrEtikets中有N個元素
string[] arrEtikets = Directory.GetFiles("");
public string[] Group2()
{
arrEtikets.Skip(arrEtikets.Length / 10);
return arrEtikets.Take(arrEtikets.Length / 10).ToArray();
}
您可以使用Linq使用GroupBy
按塊大小將數組拆分為數組列表,不使用Skip
或Take
:
private static List<T[]> SplitToChunks<T>(T[] sequence, int chunkSize)
{
return sequence.Select((item, index) => new { Index = index, Item = item })
.GroupBy(item => item.Index / chunkSize)
.Select(itemPerPage => itemPerPage.Select(v => v.Item).ToArray())
.ToList();
}
用法:
string[] arr = Enumerable.Range(0, 1000).Select(x=> x.ToString()).ToArray();
var result = SplitToChunks(arr, 101);
典型的Skip
+ Take
解決方案可以是這樣的:
public static IEnumerable<T[]> SplitArrayWithLinq<T>(T[] source, int size) {
if (null == source)
throw new ArgumentNullException("source");
else if (size <= 0)
throw new ArgumentOutOfRangeException("size", "size must be positive");
return Enumerable
.Range(0, source.Length / size + (source.Length % size > 0 ? 1 : 0))
.Select(index => source
.Skip(size * index)
.Take(size)
.ToArray());
}
如果你不能使用Linq( Skip
以及Take
包括):
public static IEnumerable<T[]> SplitArray<T>(T[] source, int size) {
if (null == source)
throw new ArgumentNullException("source");
else if (size <= 0)
throw new ArgumentOutOfRangeException("size", "size must be positive");
int n = source.Length / size + (source.Length % size > 0 ? 1 : 0);
for (int i = 0; i < n; ++i) {
T[] item = new T[i == n - 1 ? source.Length - size * i : size];
Array.Copy(source, i * size, item, 0, item.Length);
yield return item;
}
}
測試(讓我們將[1, 2, ... 8, 9]
數組分成4
項目塊):
var source = Enumerable.Range(1, 9).ToArray();
var result = SplitArray(source, 4);
string report = String.Join(Environment.NewLine,
result.Select(item => String.Join(", ", item)));
// 1, 2, 3, 4
// 5, 6, 7, 8
// 9 // <- please, notice that the last chunk has 1 item only
Console.Write(report);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.