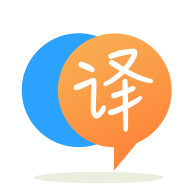
[英]How do you display a list of images, from a folder on hard drive, on ASP.NET website?
[英]How can do asp.net mvc5 caching to hard drive?
目前我正在處理一個 .net MVC 5 項目,我需要在硬盤上緩存一些數據。 如何在服務器的硬盤上緩存數據和頁面?
您可以實現自定義 OutputCacheProvider。
public class FileCacheProvider : OutputCacheProvider
{
private string _cachePath;
private string CachePath
{
get
{
if (!string.IsNullOrEmpty(_cachePath))
return _cachePath;
_cachePath = ConfigurationManager.AppSettings["OutputCachePath"];
var context = HttpContext.Current;
if (context != null)
{
_cachePath = context.Server.MapPath(_cachePath);
if (!_cachePath.EndsWith("\\"))
_cachePath += "\\";
}
return _cachePath;
}
}
public override object Add(string key, object entry, DateTime utcExpiry)
{
Debug.WriteLine("Cache.Add(" + key + ", " + entry + ", " + utcExpiry + ")");
var path = GetPathFromKey(key);
if (File.Exists(path))
return entry;
using (var file = File.OpenWrite(path))
{
var item = new CacheItem { Expires = utcExpiry, Item = entry };
var formatter = new BinaryFormatter();
formatter.Serialize(file, item);
}
return entry;
}
public override object Get(string key)
{
Debug.WriteLine("Cache.Get(" + key + ")");
var path = GetPathFromKey(key);
if (!File.Exists(path))
return null;
CacheItem item = null;
using (var file = File.OpenRead(path))
{
var formatter = new BinaryFormatter();
item = (CacheItem)formatter.Deserialize(file);
}
if (item == null || item.Expires <= DateTime.Now.ToUniversalTime())
{
Remove(key);
return null;
}
return item.Item;
}
public override void Remove(string key)
{
Debug.WriteLine("Cache.Remove(" + key + ")");
var path = GetPathFromKey(key);
if (File.Exists(path))
File.Delete(path);
}
public override void Set(string key, object entry, DateTime utcExpiry)
{
Debug.WriteLine("Cache.Set(" + key + ", " + entry + ", " + utcExpiry + ")");
var item = new CacheItem { Expires = utcExpiry, Item = entry };
var path = GetPathFromKey(key);
using (var file = File.OpenWrite(path))
{
var formatter = new BinaryFormatter();
formatter.Serialize(file, item);
}
}
private string GetPathFromKey(string key)
{
return CachePath + MD5(key) + ".txt";
}
private string MD5(string s)
{
var provider = new MD5CryptoServiceProvider();
var bytes = Encoding.UTF8.GetBytes(s);
var builder = new StringBuilder();
bytes = provider.ComputeHash(bytes);
foreach (var b in bytes)
builder.Append(b.ToString("x2").ToLower());
return builder.ToString();
}
}
並在 web.config 中注冊您的提供商
<appSettings>
<add key="OutputCachePath" value="~/Cache/" />
</appSettings>
<caching>
<outputCache defaultProvider="FileCache">
<providers>
<add name="FileCache" type="MyCacheProvider.FileCacheProvider, MyCacheProvider"/>
</providers>
</outputCache>
</caching>
我確實得到了亞歷山大的回答,但我將在這里添加一些我在途中學到的東西。 它正在使用:
使用 System.Security.Cryptography;
使用 System.IO;
使用 System.Runtime.Serialization.Formatters.Binary;
使用 System.Diagnostics;
使用 System.Web.Caching;
我需要添加這個類,它必須是可序列化的,否則會引發錯誤:
[Serializable]
public class CacheItem
{
public DateTime Expires { get; set; }
public object Item { get; set; }
}
我不得不將 web.config 條目更改為此,因為答案似乎引用了不在代碼中的命名空間。 對此不太確定,我找不到有關此標簽的任何文檔:
<outputCache defaultProvider="FileCache">
<providers>
<add name="FileCache" type="FileCacheProvider"/>
</providers>
</outputCache>
這個可能有點明顯,但是當您開始測試和使用它時,請確保到期時間為 UTC 時間:
DateTime expireTime = DateTime.UtcNow.AddHours(1);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.