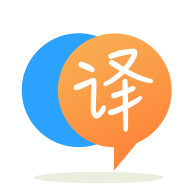
[英]When using dependency injection in C#, why does calling an interface method automatically call the implemented class' method?
[英]How to call a method defined in interface and implemented in class in C#?
我有一個名為IAp.cs
的接口文件,它實現了以下接口:
public interface IAp
{
Output Process(double min, double max, IEnumerable<string> items);
}
然后,我有一個這樣定義的類:
[Export(typeof(IAp))]
public class Ap : IAp
{
readonly ISorter _sorter;
public Ap()
{
_sorter = ContainerProvider.Container.GetExportedValue<ISorter>();
}
Output IAp.Process(double min, double max, IEnumerable<string> items)
{
// rest removed for brevity
}
}
ISorter
接口和ContainerProvider
類的定義如下,但在單獨的文件中:
interface ISorter
{
string Sort(string token);
}
internal static class ContainerProvider
{
private static CompositionContainer container;
public static CompositionContainer Container
{
get
{
if (container == null)
{
List<AssemblyCatalog> catalogList = new List<AssemblyCatalog>();
catalogList.Add(new AssemblyCatalog(typeof(ISorter).Assembly));
container = new CompositionContainer(new AggregateCatalog(catalogList));
}
return container;
}
}
}
現在,在另一個源文件中,我想調用Process
方法。 我做了這樣的事情:
private readonly IAp _ap;
Output res = _ap.Process(50, 100, items);
但這表示_ap
從未分配給它,並且它為null,因此它不調用Process
方法。 它將引發NullReference
錯誤。
我還嘗試如下初始化該類:
private Ap app = new Ap();
但是,如果我做app.
要訪問其成員,它找不到Process
方法,它不存在。 有什么想法如何在另一個源文件和類中調用Process
方法嗎?
您需要實例化該類,然后將其引用作為接口類型保存(因為Ap
從接口IAp
繼承,因此由於多態性而合法),例如:
private readonly IAp _ap = new Ap();
Output res = _ap.Process(50, 100, items);
private IAp app = new Ap();
這是因為Ap
實現了IAp
。 之所以不能做您想做的事情,是因為您使用的是顯式接口實現,所以接口方法僅在對接口的引用上可見,而在類上不可見。
另一個解決方法是不使用顯式接口實現,但是這可能會使您直接引用類型,通常認為這不是最佳實踐。
代碼僅顯示更改的簽名實現。
public class Ap : IAp
{
public Output Process(double min, double max, IEnumerable<string> items)
{
// rest removed for brevity
}
}
現在,使用您現有的參考也可以。
有關更多信息,請參閱《 接口-C#編程指南》
您看到的行為是因為接口已顯式實現。 您需要執行以下一項操作:
將App
實例分配給IAp
類型的變量:
IAp _ap = new Ap(); //now you can do _app.Process
隱式實現接口:
public class Ap: IAp { public Output Process(....) { ... } } Ap _ap = new Ap(); //now you can do _ap.Process
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.