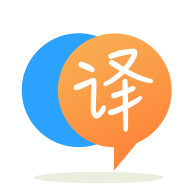
[英]Node.js Express : How to redirect page after processing post request?
[英]Node.js with Express: how to redirect a POST request
我想從一個 URL 請求重定向到另一個“POST”請求,如下所示:
var app = require('express')(); app.get('/', function(req, res) { res.redirect('/test'); }); app.post('/test', function(req, res) { res.send('/test page'); }); app.listen(3000, function() { console.log('listenning on port:3000'); });
但是,我無法重定向到“/test”頁面,因為它是一個 POST 請求。
那么我應該怎么做才能使重定向工作,同時保持 '/test' 請求 POST?
你可以這樣做:
app.post('/', function(req, res) {
res.redirect(307, '/test');
});
這將保留發送方法。
作為參考,307 http 代碼規范是:
307 Temporary Redirect (因為HTTP/1.1) 這種情況下,請求應該用另一個URI重復,但以后的請求仍然可以使用原來的URI。2 與303相反,重新發出原始請求時不應改變請求方法. 例如,必須使用另一個 POST 請求重復 POST 請求。
有關更多信息,請參閱: http : //www.alanflavell.org.uk/www/post-redirect.html
請記住中間件架構:每個處理程序都可以操作上下文,然后響應或調用next()
。
在這個前提下,express router 基本上是一個中間件功能,你可以在“更正”url 后使用。
(順便說一句,請求應用程序也是一個功能,雖然我不確定我是否建議在鏈的早期返回)
這是一個例子:
const router = new require('express').Router()
const user = require('../model/user')
//assume user implements:
// user.byId(id) -> Promise<user>
// user.byMail(email) -> Promise<user>
const reqUser = userPromise => (req, res, next) =>
req.user
? next()
: userPromise(req)
.then(user => { req.user = user })
.then(next, next)
//assume the sever that uses this router has a
//standard (err, req, res, next) handler in the end of the chain...
const byId = reqUser( req => user.byId(req.params.id) )
const byMail = reqUser( req => user.byMail(req.params.mail) )
router.post('/by-id/:id/friends',
byId,
(req, res) => res.render('user-friends', req.user)
)
router.post('/by-email/:email/friends',
byMail,
(req, res, next) => {
req.url = `/by-id/${req.user.id}/friends`
next()
},
router
)
307 和 302 的唯一區別是 307 保證在進行重定向請求時不會更改方法和主體。
https://developer.mozilla.org/en-US/docs/Web/HTTP/Status/307
我相信問題是節點服務器正在接收 POST 請求,但需要將其作為 GET 請求重定向到不同的服務器。 我最近不得不處理類似的事情。 這是我解決它的方法:
var proxy = require('express-http-proxy');
app.use('incomin/url', proxy('forwarding:server', {
//The proxyRqDecorator allows us to change a few things including the request type.
proxyReqOptDecorator: (proxyReqOpts, srcReq) => {
proxyReqOpts.method = 'GET';
return proxyReqOpts;
},
//The proxyReqPathResolver takes the Given URL and updates it to the forward path
proxyReqPathResolver: function (req) {
return new Promise( (resolve, reject) => {
setTimeout( () =>{
var value = req.body.key;
var resolvedPathValue = 'forwarding/url' + value;
console.log(`Inside forward path. The resolved path is ${resolvedPathValue}`);
resolve(resolvedPathValue);
}, 200);
});
}
}));
請記住,上面的 proxyReqPathResolver 是異步設置的。 這里描述了同步版本和 express-http-proxy 的更多信息: https : //www.npmjs.com/package/express-http-proxy
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.