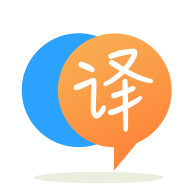
[英]unable to sign In for MultiTenant Azure AD using Custom policies in AAD B2C from my asp.net core web app
[英]Azure AD B2C with ASP.NET Core - Unable to go to edit profile
我試着尋找與此有關的問題,但找不到任何東西。
我有一個ASP.NET Core 1.0應用程序,它使用Azure AD B2C進行身份驗證。 簽署和注冊以及簽署工作就好了。 當我嘗試編輯用戶的個人資料時出現問題。 這是我的Startup.cs的樣子:
namespace AspNetCoreBtoC
{
public class Startup
{
private IConfigurationRoot Configuration { get; }
public Startup(IHostingEnvironment env)
{
var builder = new ConfigurationBuilder()
.SetBasePath(env.ContentRootPath)
.AddJsonFile("appsettings.json", optional: false, reloadOnChange: true)
.AddEnvironmentVariables();
Configuration = builder.Build();
}
// This method gets called by the runtime. Use this method to add services to the container.
// For more information on how to configure your application, visit http://go.microsoft.com/fwlink/?LinkID=398940
public void ConfigureServices(IServiceCollection services)
{
services.AddSingleton<IConfiguration>(Configuration);
services.AddMvc();
services.AddAuthentication(
opts => opts.SignInScheme = CookieAuthenticationDefaults.AuthenticationScheme);
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IHostingEnvironment env, ILoggerFactory loggerFactory)
{
loggerFactory.AddConsole();
if (env.IsDevelopment())
{
loggerFactory.AddDebug(LogLevel.Debug);
app.UseDeveloperExceptionPage();
}
app.UseStaticFiles();
app.UseCookieAuthentication(new CookieAuthenticationOptions
{
AutomaticChallenge = false
});
string signUpPolicyId = Configuration["AzureAd:SignUpPolicyId"];
string signUpCallbackPath = Configuration["AzureAd:SignUpCallbackPath"];
app.UseOpenIdConnectAuthentication(CreateOidConnectOptionsForPolicy(signUpPolicyId, false, signUpCallbackPath));
string userProfilePolicyId = Configuration["AzureAd:UserProfilePolicyId"];
string profileCallbackPath = Configuration["AzureAd:ProfileCallbackPath"];
app.UseOpenIdConnectAuthentication(CreateOidConnectOptionsForPolicy(userProfilePolicyId, false, profileCallbackPath));
string signInPolicyId = Configuration["AzureAd:SignInPolicyId"];
string signInCallbackPath = Configuration["AzureAd:SignInCallbackPath"];
app.UseOpenIdConnectAuthentication(CreateOidConnectOptionsForPolicy(signInPolicyId, true, signInCallbackPath));
app.UseMvc(routes =>
{
routes.MapRoute(
name: "Default",
template: "{controller=Home}/{action=Index}/{id?}");
});
}
private OpenIdConnectOptions CreateOidConnectOptionsForPolicy(string policyId, bool autoChallenge, string callbackPath)
{
string aadInstance = Configuration["AzureAd:AadInstance"];
string tenant = Configuration["AzureAd:Tenant"];
string clientId = Configuration["AzureAd:ClientId"];
string redirectUri = Configuration["AzureAd:RedirectUri"];
var opts = new OpenIdConnectOptions
{
AuthenticationScheme = policyId,
MetadataAddress = string.Format(aadInstance, tenant, policyId),
ClientId = clientId,
PostLogoutRedirectUri = redirectUri,
ResponseType = "id_token",
TokenValidationParameters = new TokenValidationParameters
{
NameClaimType = "name"
},
CallbackPath = callbackPath,
AutomaticChallenge = autoChallenge
};
opts.Scope.Add("openid");
return opts;
}
}
}
這是我的AccountController,從中我向中間件發出挑戰:
namespace AspNetCoreBtoC.Controllers
{
public class AccountController : Controller
{
private readonly IConfiguration config;
public AccountController(IConfiguration config)
{
this.config = config;
}
public IActionResult SignIn()
{
return Challenge(new AuthenticationProperties
{
RedirectUri = "/"
},
config["AzureAd:SignInPolicyId"]);
}
public IActionResult SignUp()
{
return Challenge(new AuthenticationProperties
{
RedirectUri = "/"
},
config["AzureAd:SignUpPolicyId"]);
}
public IActionResult EditProfile()
{
return Challenge(new AuthenticationProperties
{
RedirectUri = "/"
},
config["AzureAd:UserProfilePolicyId"]);
}
public IActionResult SignOut()
{
string returnUrl = Url.Action(
action: nameof(SignedOut),
controller: "Account",
values: null,
protocol: Request.Scheme);
return SignOut(new AuthenticationProperties
{
RedirectUri = returnUrl
},
config["AzureAd:UserProfilePolicyId"],
config["AzureAd:SignUpPolicyId"],
config["AzureAd:SignInPolicyId"],
CookieAuthenticationDefaults.AuthenticationScheme);
}
public IActionResult SignedOut()
{
return View();
}
}
}
我試圖從OWIN示例中調整它。 我遇到的問題是,為了編輯配置文件,我必須向負責此操作的OpenIdConnect中間件發出挑戰。 問題是它調用了中間件(Cookies)中的默認簽名,這意味着用戶已經過身份驗證,因此操作必須是未經授權的操作,並嘗試重定向到/ Account / AccessDenied(即使我甚至沒有在該路線上有任何東西),而不是去Azure AD來編輯它應該的配置文件。
有沒有人在ASP.NET Core中成功實現用戶配置文件編輯?
好吧,我終於解決了。 我寫了一篇關於設置的博客文章,其中包括解決方案: https : //joonasw.net/view/azure-ad-b2c-with-aspnet-core 。 問題是ChallengeBehavior,必須設置為Unauthorized,而不是默認值Automatic。 目前無法使用ChallengeResult框架定義它,所以我自己做了:
public class MyChallengeResult : IActionResult
{
private readonly AuthenticationProperties authenticationProperties;
private readonly string[] authenticationSchemes;
private readonly ChallengeBehavior challengeBehavior;
public MyChallengeResult(
AuthenticationProperties authenticationProperties,
ChallengeBehavior challengeBehavior,
string[] authenticationSchemes)
{
this.authenticationProperties = authenticationProperties;
this.challengeBehavior = challengeBehavior;
this.authenticationSchemes = authenticationSchemes;
}
public async Task ExecuteResultAsync(ActionContext context)
{
AuthenticationManager authenticationManager =
context.HttpContext.Authentication;
foreach (string scheme in authenticationSchemes)
{
await authenticationManager.ChallengeAsync(
scheme,
authenticationProperties,
challengeBehavior);
}
}
}
抱歉這個名字......但是這一個可以從一個控制器動作返回,通過指定ChallengeBehavior.Unauthorized,我得到了一切正常的工作。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.