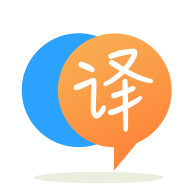
[英]Bind GridView With Database In MVC 5 In C# and i have ajax JavaScript
[英]Add record to database with C#/MVC/Javascript
我有一個高爾夫球場及其各自面值的數據庫表。 我正在嘗試在桌子上增加一排新高爾夫球場。 該表具有3列ID
, CourseName
和Par
。
嘗試添加行時,代碼將僅為CourseName
和Par
設置一個值,因為ID
是一個標識字段。
當我單擊“ New Course
按鈕時,行為是錯誤的-代碼從表中獲取第一行並對其進行編輯,而不是創建新行。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using GolfScore.Data;
namespace GolfScore.Business
{
public class CourseManager
{
public static void AddCourse(string Name, int Par)
{
using (DBContext context = new DBContext())
{
Course j = context.Courses.Create();
context.Courses.Add(j);
context.SaveChanges();
}
}
public static void EditCourse(int? id, string Name, int Par)
{
using (DBContext context = new DBContext())
{
Course t = context.Courses.Where(c => c.CourseId == id).FirstOrDefault();
t.CourseName = Name;
t.Par = Par;
context.SaveChanges();
}
}
public static Course GetCourse(int? id)
{
if (id.HasValue) {
using (DBContext context = new DBContext())
{
return context.Courses.Where(c => c.CourseId == id.Value).FirstOrDefault();
}
}
else
{
using (DBContext context = new DBContext())
{
return context.Courses.FirstOrDefault();
}
}
}
public static IList<Course> GetAllCourses()
{
using(DBContext context = new DBContext())
{
return context.Courses.ToList();
}
}
}
}
CourseController
using GolfScore.Business;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using GolfScore.Web.Models;
namespace GolfScore.Web.Controllers
{
public class CourseController : Controller
{
// GET: Course
public ActionResult Index()
{
return View(CourseManager.GetAllCourses());
}
[HttpGet]
public PartialViewResult AddEdit(int? id)
{
return PartialView("_AddEditCourse", CourseManager.GetCourse(id));
}
[HttpPost]
public JsonResult Save(Course c)
{
if (c.Id.HasValue)
{
CourseManager.EditCourse(c.Id, c.Name, c.Par);
}
else
{
CourseManager.AddCourse(c.Name, c.Par);
}
return Json(new
{
Success = true
});
}
}
}
Java腳本
var Course = function (dialogSelector, addUrl, saveUrl) {
self = this;
self.dialogElement = dialogSelector;
self.addAJAXUrl = addUrl;
self.saveAJAXUrl = saveUrl;
self.dialog = null;
self.Initialize = function () {
self.dialog = $(self.dialogElement).dialog({
autoOpen: false,
height: 400,
width: 350,
modal: true,
buttons: [
{
text: "Save",
click: function () {
var jsonObject = {
"Id": parseInt($("#CourseId").val()),
"Name": $("#CourseName").val(),
"Par": parseInt($("#Par").val())
};
$.ajax({
url: self.saveAJAXUrl,
type: "POST",
contentType: "application/json",
dataType: "json",
data: JSON.stringify(jsonObject),
success: function () {
self.dialog.dialog("close");
location.reload();
},
error: function (a, b, c) {
alert("stupid");
}
})
}
},
{
text: "Cancel",
click: function (){
self.dialog.dialog("close");
}
}
],
close: function () {
self.dialog.dialog("close");
}
});
$(".newBtn").on("click", function () {
$.ajax({
url: self.addAJAXUrl,
type: "GET",
success: function (data) {
$(self.dialogElement).html(data);
self.dialog.dialog("option", "title", "New Course");
self.dialog.dialog("open");
},
error: function (a, b, c) {
alert("Error.");
}
})
});
$(".editBtn").on("click", function (e) {
$.ajax({
url: self.addAJAXUrl + "?Id=" + $(e.target).attr("data-course-id"),
type: "GET",
success: function (data) {
$(self.dialogElement).html(data);
self.dialog.dialog("option", "title", "Edit Course");
self.dialog.dialog("open");
},
error: function (a, b, c) {
alert("Error.");
}
})
});
};
}
如果您還有其他需要,請告訴我。
提前致謝。
抱歉,這是課程。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace GolfScore.Web.Models
{
[Serializable()]
public class Course
{
public int? Id { get; set; }
public string Name { get; set; }
public int Par { get; set; }
}
}
[HttpGet]
public PartialViewResult AddEdit(int? id)
{
return PartialView("_AddEditCourse", CourseManager.GetCourse(id));
}
您需要這樣更改此操作:
[HttpGet]
public PartialViewResult AddEdit(int? id)
{
if(id.HasValue)
return PartialView("_AddEditCourse", CourseManager.GetCourse(id));
return PartialView("_AddEditCourse",new Course())
}
如果id具有值,則GetCourse方法必須僅返回Course。
保存時,您需要檢查id == 0,因為如果是新記錄,則id = 0,而id> 0。
編輯:
您的課程學習方法必須是這樣的:
public static Course GetCourse(int id){
using (DBContext context = new DBContext())
{
return context.Courses.Find(id);
}
}
看看為什么會出錯,我需要您發布剃刀視圖。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.