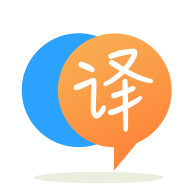
[英]Passing data from Controller, to View, and back to Controller
[英]Passing a value of a textbox from View to a method/action in Controller and then data from response back to form
我在創建視圖中有一個表單(不確定是否相關,但使用ChameleonForms完成)。
名為“ CompanyNumber”的編輯框的波紋管下有一個html按鈕,我希望OnClick將已經寫好的編輯框的值作為參數發送給我的Controller中的方法,但不提交表單,因為在保存表單之前,我必須手動添加未使用json響應檢索的字段值。
主要是該方法發出一個webrequest並返回一個json,我在該方法中使用json來填充與給定視圖相關的模型,其中包含我可以獲得的所有可用信息。
因此,目前,我的觀點是:
@*Form region*@
@s.FieldFor(m => m.CompanyNumber)
<button id="Ask" type="button" class="btn btn-default">Ask the web!</button>
@*Script region*@
<script type="text/javascript">
$('#Ask').click(function(){
var companynumber = $('#CompanyNumber').val();
$.ajax({
type: 'POST',
data:{CompanyNumber:companynumber},
//url from the controller's action method
url: '@Url.Action("Customers","GetCompanyInfo")/?CompanyNumber='+$('#CompanyNumber').val(),
//with the following 2 lines, raise HTTP500
//dataType: 'json',
//contentType: "application/json; charset=utf-8",
success: function (data) {
//Here you would update the textboxes, the 'data' variable contains the html from the partialview
//alert("here we have: " + data.d.toString());
},
error: function () {
//Manage errors
}
});
}
)
</script>
在控制器中:
private InvoiceDBEntities db = new InvoiceDBEntities();
public Customers cModel = new Customers();
//Ask the web for company details
public ActionResult GetCompanyInfo(string CompanyNumber)
{
//call for openapi.ro
string CompanyCUI = CompanyNumber;
// Create a new 'Uri' object with the specified string.
Uri myUri = new Uri("https://api.openapi.ro/api/companies/" + CompanyCUI + ".json");
// Create a new request to the above mentioned URL.
WebRequest myWebRequest = WebRequest.Create(myUri);
//Add the required header to request
myWebRequest.Headers.Add("x-api-key", "some_key_relevant_to_me_and_my_scenario");
// Assign the response object of 'WebRequest' to a 'WebResponse' variable.
WebResponse myWebResponse = myWebRequest.GetResponse();
// Read the response into a stream
var dataStream = myWebResponse.GetResponseStream();
var reader = new StreamReader(dataStream);
var jsonResultString = reader.ReadToEnd();
// Deserialize
var CompanyInfoData = Newtonsoft.Json.JsonConvert.DeserializeObject<CustomerModels>(jsonResultString);
//Bind to model for feed him
cModel.Phone1 = CompanyInfoData.telefon;
cModel.CompanyRegistration = CompanyInfoData.numar_reg_com;
cModel.Name = CompanyInfoData.denumire;
cModel.CompanyNumber = CompanyInfoData.cif;
cModel.Address = CompanyInfoData.adresa;
//other properties of the related model....
ViewData["Customer"] = cModel;
return View(cModel);
}
//other actions
// GET: Customers/Create
public ActionResult Create()
{
return View();
}
// POST: Customers/Create
// To protect from overposting attacks, please enable the specific properties you want to bind to, for
// more details see http://go.microsoft.com/fwlink/?LinkId=317598.
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Create([Bind(Include = "CustomerID,Name,CompanyNumber,CompanyRegistration,CompanyBank,CompanyIBAN,Address,CP,City,ContactPerson,Phone1,Phone2,Fax,Email,Notes")] Customers customers)
{
if (ModelState.IsValid)
{
db.Customers.Add(customers);
db.SaveChanges();
return RedirectToAction("Index");
}
return View(customers);
}
最后,我獲得所需的json響應,然后用數據填充模型。 因此,我的問題是如何指示該方法以相同的形式將獲取的值作為文本值發送回相關的編輯框?
之后,在將表單發送到保存之前,我將能夠進行驗證檢查和其他工作
我必須說,第一步是我對此進行了實驗,將CompanyNumber硬編碼到我的方法中(實際上,所有方法代碼都在控制器的默認Index動作中),並且對於與Controller相關聯的Index視圖,它可以正常工作,已填寫表格以及我能夠檢索到的所有可用數據值。
問候
您應該使用ajax調用來指向控制器中的操作,並以部分視圖(或json,視情況而定)檢索信息。 以下示例使用JQuery
$(#Ask).click(function(){
var companynumber = $('#CompanyNumber').val();
$.ajax({
type: 'POST',
data:{
CompanyNumber:companynumber
},
//url from the controller's action method
url: url,
success: function (data) {
//Here you would update the textboxes, the 'data'
//variable contains the html from the partialview
},
error: function () {
//Manage errors
}
})
}
最后,我使它起作用,我將僅添加與可能需要類似內容的人員相關的信息:
視圖末尾的腳本:
<script type="text/javascript">
$('#Ask').click(function () {
var companynumber = $('#CompanyNumber').val();
$.ajax({
type: 'POST',
data: {
CompanyNumber: companynumber
},
//url from the controller's action method e.g. GetCompanyInfo
url: '/Customers/GetCompanyInfo/',
dataType: "json",
success: function (data) {
//Here you would update the textboxes, the 'data' variable contains the html/text from the fill
$('#CompanyNumber').val(data.cif);
$('#CompanyRegistration').val(data.numar_reg_com);
$('#Name').val(data.denumire);
$('#Phone1').val(data.telefon);
$('#Fax').val(data.fax);
$('#Address').val(data.adresa);
$('#ZIP').val(data.cod_postal);
//alert("here: " + data.toString());
},
error: function () {
//Manage errors
}
});
//console.log();
}
)
</script>
控制器(簡體):
public ActionResult GetCompanyInfo(string CompanyNumber)
{
//call for openapi.ro
string CompanyCUI = CompanyNumber;
// Create a new 'Uri' object with the specified string.
Uri myUri = new Uri("https://api.openapi.ro/api/companies/" + CompanyCUI + ".json");
// Create a new request to the above mentioned URL.
WebRequest myWebRequest = WebRequest.Create(myUri);
//Add the required header to request
myWebRequest.Headers.Add("x-api-key", "some_key_relevant_to_me_and_my_scenario");
// Assign the response object of 'WebRequest' to a 'WebResponse' variable.
WebResponse myWebResponse = myWebRequest.GetResponse();
// Read the response into a stream
var dataStream = myWebResponse.GetResponseStream();
var reader = new StreamReader(dataStream);
var jsonResultString = reader.ReadToEnd();
// Deserialize
var CompanyInfoData = Newtonsoft.Json.JsonConvert.DeserializeObject<CustomerModels>(jsonResultString);
return Json(CompanyInfoData,JsonRequestBehavior.AllowGet);
}
和視圖的表單部分(在我的情況下是使用ChameleonForms完成的,但並不重要):
@using ChameleonForms
@using ChameleonForms.Component
@using ChameleonForms.Enums
@using ChameleonForms.Templates
@using ChameleonForms.ModelBinders
@model DirectInvoice.Models.Customers
@{
ViewBag.Title = "Create";
}
<div>
@using (var f = Html.BeginChameleonForm())
{
@Html.AntiForgeryToken()
using (var s = f.BeginSection("Add a new customer"))
{
@s.FieldFor(m => m.CompanyNumber)
@*<button type="button" onclick="location.href='@Url.Action("GetCompanyInfo", "Customers", new { id = "Ask" })'" class="btn btn-default">Ask the web!</button>*@
<button id="Ask" type="button" class="btn btn-default">Ask the web!</button>
@s.FieldFor(m => m.Name)
@s.FieldFor(m => m.CompanyRegistration)
@s.FieldFor(m => m.Phone1).Placeholder("0XX X XXX XXX")
@s.FieldFor(m => m.Fax).Placeholder("0XX X XXX XXX")
@s.FieldFor(m => m.Email).Placeholder("name@email.com")
@s.FieldFor(m => m.Address)
@s.FieldFor(m => m.ZIP)
@s.FieldFor(m => m.City)
}
using (var n = f.BeginNavigation())
{
@n.Submit("Save the new customer...")
}
}
</div>
謝謝大家的想法!
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.