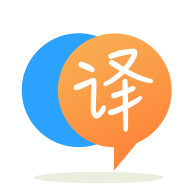
[英]WCF Service, the type provided as the service attribute values…could not be found
[英]WCF Singleton-Service error: The service type provided could not be loaded as a service because it does not have a default constructor
我在WCF中遇到以下奇怪的問題,無法確定原因:
我正在與WCF合作,以確定是否將其用於需要為類似打印機的設備實現的遠程控制API。 該設備由Windows-PC控制,該Windows-PC運行.Net中實現的控制器軟件。 我需要為該軟件實現API。
該服務是從控制器軟件內部自我托管的,目前我正在弄清楚如何創建WCF服務的單例實例,以便可以通過控制器軟件來創建具有相應對象/類的實例。 我已經使用簡化版本使它工作了,但是奇怪的是,如果服務不包含默認(無參數)構造函數,則會收到此警告。 即使是很奇怪,我也確實在做,第二句話告訴我的異常(或者至少我想以為是)。 此異常在帶有標題WCF Service Host
單獨窗口中引發,此后此程序繼續正常執行:
System.InvalidOperationException:由於提供的服務類型沒有默認(無參數)構造函數,因此無法作為服務加載。 要解決此問題,請向類型添加默認構造函數,或將類型的實例傳遞給主機。
在System.ServiceModel.Description.ServiceDescription.CreateImplementation(Type serviceType)
在System.ServiceModel.Description.ServiceDescription.SetupSingleton(ServiceDescription serviceDescription,對象實現,布爾值isWellKnown)
在System.ServiceModel.Description.ServiceDescription.GetService(類型serviceType)
在System.ServiceModel.ServiceHost.CreateDescription(IDictionary`2&ImplementedContracts)
在System.ServiceModel.ServiceHostBase.InitializeDescription(UriSchemeKeyedCollection baseAddresses)
在System.ServiceModel.ServiceHost..ctor(類型serviceType,Uri [] baseAddresses)
在Microsoft.Tools.SvcHost.ServiceHostHelper.CreateServiceHost(類型,ServiceKind類型)
在Microsoft.Tools.SvcHost.ServiceHostHelper.OpenService(ServiceInfo信息)
這是我用來創建服務的代碼。 我在Service.cs
注釋了注釋行,其中包含默認構造函數。 有趣的是,當我包含默認構造函數時(因此永遠不會引發錯誤),它永遠不會被調用(我通過設置斷點來確認)。 如果您沒有對此進行評論,則不會引發異常。
Server.cs
:
public class Server
{
private ServiceHost svh;
private Service service;
public Server()
{
service = new Service("A fixed ctor test value that the service should return.");
svh = new ServiceHost(service);
}
public void Open(string ipAdress, string port)
{
svh.AddServiceEndpoint(
typeof(IService),
new NetTcpBinding(),
"net.tcp://"+ ipAdress + ":" + port);
svh.Open();
}
public void Close()
{
svh.Close();
}
}
Service.cs
:
[ServiceBehavior(ConcurrencyMode = ConcurrencyMode.Reentrant,
InstanceContextMode = InstanceContextMode.Single)]
public class Service : IService
{
private string defaultString;
public Service(string ctorTestValue)
{
this.defaultString = ctorTestValue;
}
//// when this constructor is uncommented, I do not get the error
//public Service()
//{
// defaultString = "Default value from the ctor without argument.";
//}
public string GetDefaultString()
{
return defaultString;
}
public string GetData(int value)
{
return string.Format("You entered: {0}", value);
}
public CompositeType GetDataUsingDataContract(CompositeType composite)
{
if (composite == null)
{
throw new ArgumentNullException("composite");
}
if (composite.BoolValue)
{
composite.StringValue += "Suffix";
}
return composite;
}
public string Ping(string name)
{
Console.WriteLine("SERVER - Processing Ping('{0}')", name);
return "Hello, " + name;
}
static Action m_Event1 = delegate { };
static Action m_Event2 = delegate { };
public void SubscribeEvent1()
{
IMyEvents subscriber = OperationContext.Current.GetCallbackChannel<IMyEvents>();
m_Event1 += subscriber.Event1;
}
public void UnsubscribeEvent1()
{
IMyEvents subscriber = OperationContext.Current.GetCallbackChannel<IMyEvents>();
m_Event1 -= subscriber.Event1;
}
public void SubscribeEvent2()
{
IMyEvents subscriber = OperationContext.Current.GetCallbackChannel<IMyEvents>();
m_Event2 += subscriber.Event2;
}
public void UnsubscribeEvent2()
{
IMyEvents subscriber = OperationContext.Current.GetCallbackChannel<IMyEvents>();
m_Event2 -= subscriber.Event2;
}
public static void FireEvent1()
{
m_Event1();
}
public static void FireEvent2()
{
m_Event2();
}
public static Timer Timer1;
public static Timer Timer2;
public void OpenSession()
{
Timer1 = new Timer(1000);
Timer1.AutoReset = true;
Timer1.Enabled = true;
Timer1.Elapsed += OnTimer1Elapsed;
Timer2 = new Timer(500);
Timer2.AutoReset = true;
Timer2.Enabled = true;
Timer2.Elapsed += OnTimer2Elapsed;
}
void OnTimer1Elapsed(object sender, ElapsedEventArgs e)
{
FireEvent1();
}
void OnTimer2Elapsed(object sender, ElapsedEventArgs e)
{
FireEvent2();
}
}
IServices.cs
:
public interface IMyEvents
{
[OperationContract(IsOneWay = true)]
void Event1();
[OperationContract(IsOneWay = true)]
void Event2();
}
// NOTE: You can use the "Rename" command on the "Refactor" menu to change the interface name "IService1" in both code and config file together.
[ServiceContract(CallbackContract = typeof(IMyEvents))]
public interface IService
{
[OperationContract]
string GetData(int value);
[OperationContract]
string GetDefaultString();
[OperationContract]
CompositeType GetDataUsingDataContract(CompositeType composite);
// TODO: Add your service operations here
[OperationContract]
string Ping(string name);
[OperationContract]
void SubscribeEvent1();
[OperationContract]
void UnsubscribeEvent1();
[OperationContract]
void SubscribeEvent2();
[OperationContract]
void UnsubscribeEvent2();
[OperationContract]
void OpenSession();
}
// Use a data contract as illustrated in the sample below to add composite types to service operations.
// You can add XSD files into the project. After building the project, you can directly use the data types defined there, with the namespace "WcfService.ContractType".
[DataContract]
public class CompositeType
{
bool boolValue = true;
string stringValue = "Hello ";
[DataMember]
public bool BoolValue
{
get { return boolValue; }
set { boolValue = value; }
}
[DataMember]
public string StringValue
{
get { return stringValue; }
set { stringValue = value; }
}
}
Main
用於啟動服務器:
static void Main(string[] args)
{
// start server
var server = new Server();
server.Open("localhost", "6700");
Console.WriteLine("Server started.");
Console.ReadLine();
server.Close();
}
該問題是由在Visual Studio中調試時運行的WcfSvcHost引起的。 根據此 ,“WCF服務主機在一個WCF服務項目中列舉的服務,加載項目的配置,並實例為發現的每個服務的主機。該工具通過WCF服務模板集成到Visual Studio和被調用時,你開始調試您的項目。”
由於您是自托管的,因此不需要使用WCF服務主機,因此可以通過包含該服務的項目的項目屬性頁將其禁用。 您應該在屬性頁上看到“ WCF選項”的選項卡。 然后,關閉“調試時啟動WCF服務主機...”選項。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.