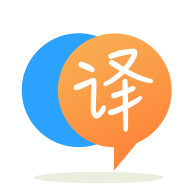
[英]How do I retrieve text from database in list form (SQL Server & ASP.NET MVC)
[英]How do I retrieve an image from a database in MVC?
我一直在研究一個系統,允許我們的用戶將圖像上傳到數據庫中。 將圖像上傳到數據庫似乎工作正常,但我似乎無法將圖像取回並顯示在我的視圖中。 我沒有收到任何錯誤消息,圖像根本沒有顯示。 到目前為止,這是我的代碼。
控制器/創建方法
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Create(UserAccount userAccount, HttpPostedFileBase upload)
{
try
{
if (ModelState.IsValid)
{
if (upload != null && upload.ContentLength > 0)
{
var photo = new UserImage
{
FileName = System.IO.Path.GetFileName(upload.FileName),
FileType = FileType.VesselImage,
ContentType = upload.ContentType
};
using (var reader = new System.IO.BinaryReader(upload.InputStream))
{
photo.Content = reader.ReadBytes(upload.ContentLength);
}
userAccount.UserImages = new List<UserImage> { photo };
}
db.UserAccounts.Add(userAccount);
db.SaveChanges();
return RedirectToAction("Index");
}//if
}//try
catch (RetryLimitExceededException /* dex */)
{
//Log the error (uncomment dex variable name and add a line here to write a log.
ModelState.AddModelError("", "Unable to save changes. Try again, and if the problem persists see your system administrator.");
}
return View(userAccount);
}
Controller / Details Method Breakpoints 顯示在此階段找到並選擇了圖像。
public ActionResult Details(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
UserAccount userAccount = db.UserAccounts
.Include(s => s.UserImages)
.SingleOrDefault(s => s.userId == id);
if (userAccount == null)
{
return HttpNotFound();
}
return View(userAccount);
}
查看/詳細信息
@model Multiple_Table_Post.Models.UserAccount
@{
ViewBag.Title = "Details";
}
<h2>Details</h2>
<div>
<h4>UserAccount</h4>
<hr />
<dl class="dl-horizontal">
<dt>
@Html.DisplayNameFor(model => model.userName)
</dt>
<dd>
@Html.DisplayFor(model => model.userName)
</dd>
<dt>
@Html.DisplayNameFor(model => model.userLocation)
</dt>
<dd>
@Html.DisplayFor(model => model.userLocation)
</dd>
@if (Model.UserImages.Any(f => f.FileType == FileType.VesselImage))
{
<dt>
Image
</dt>
<dd>
<img src="~/File?id=@Model.UserImages.First(f => f.FileType == FileType.VesselImage).FileId" alt="avatar" />
</dd>
}
</dl>
</div>
<p>
@Html.ActionLink("Edit", "Edit", new { id = Model.userId }) |
@Html.ActionLink("Back to List", "Index")
</p>
我認為我的情況有問題:
@if (Model.UserImages.Any(f => f.FileType == FileType.VesselImage))
{
<dt>
Image
</dt>
<dd>
<img src="~/File?id=@Model.UserImages.First(f => f.FileType == FileType.VesselImage).FileId" alt="avatar" />
</dd>
}
如果我在視圖中的這一點中斷,我可以看到圖像已被選中,但注意到一直在輸出。 我只是什么都不回。
我也包括了我的模型,希望我能找到這個問題的根源。 他們來了:
模型/用戶帳戶
namespace Multiple_Table_Post.Models
{
using System;
using System.Collections.Generic;
public partial class UserAccount
{
public int userId { get; set; }
public string userName { get; set; }
public string userLocation { get; set; }
public virtual ICollection<UserImage> UserImages { get; set; }
}
}
Model / UserImage 命名空間 Multiple_Table_Post.Models { using System; 使用 System.Collections.Generic;
public partial class UserImage
{
public int FileId { get; set; }
public string FileName { get; set; }
public string ContentType { get; set; }
public byte[] Content { get; set; }
public Nullable<int> PersonId { get; set; }
public FileType FileType { get; set; }
public virtual UserAccount UserAccount { get; set; }
}
}
型號/文件類型
namespace Multiple_Table_Post.Models
{
public enum FileType
{
VesselImage = 1, Photo
}
}
謝謝,如果我錯過了任何能讓這更容易的東西,請告訴我。
更新:這里我已經包含了關於系統在滿足條件時正在做什么並且數據可以看到的斷點信息。
我更喜歡這樣做的方式是創建一個 ActionResult 來直接為瀏覽器對圖像的請求提供服務。 這種方法的優點之一是如果頁面多次包含圖像,瀏覽器將自動共享資源。 這可以節省數據庫命中、數據傳輸並最大限度地提高緩存機會。
public ActionResult Portrait(int id)
{
vPhotos p = sdb.vPhotos.Where(e => e.ID == id).SingleOrDefault();
byte[] photoArray = new byte[] { };
return File(smallPhotoArray, "image/png");
}
現在,在您看來,只需將 ActionMethod 作為 img src 引用調用:
<img src="@Url.Action("Portrait", "MyController" , new { id = id })" />
嘗試這個:
<img src="data:image/jpg;base64,[byte array]"/>
代替:
jpg
與圖像類型,即FileType
[byte array]
與您的字節數組,即Content
。 如果尚未將Content
轉換為base64
則需要將其轉換。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.